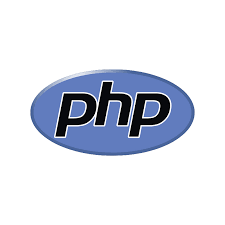
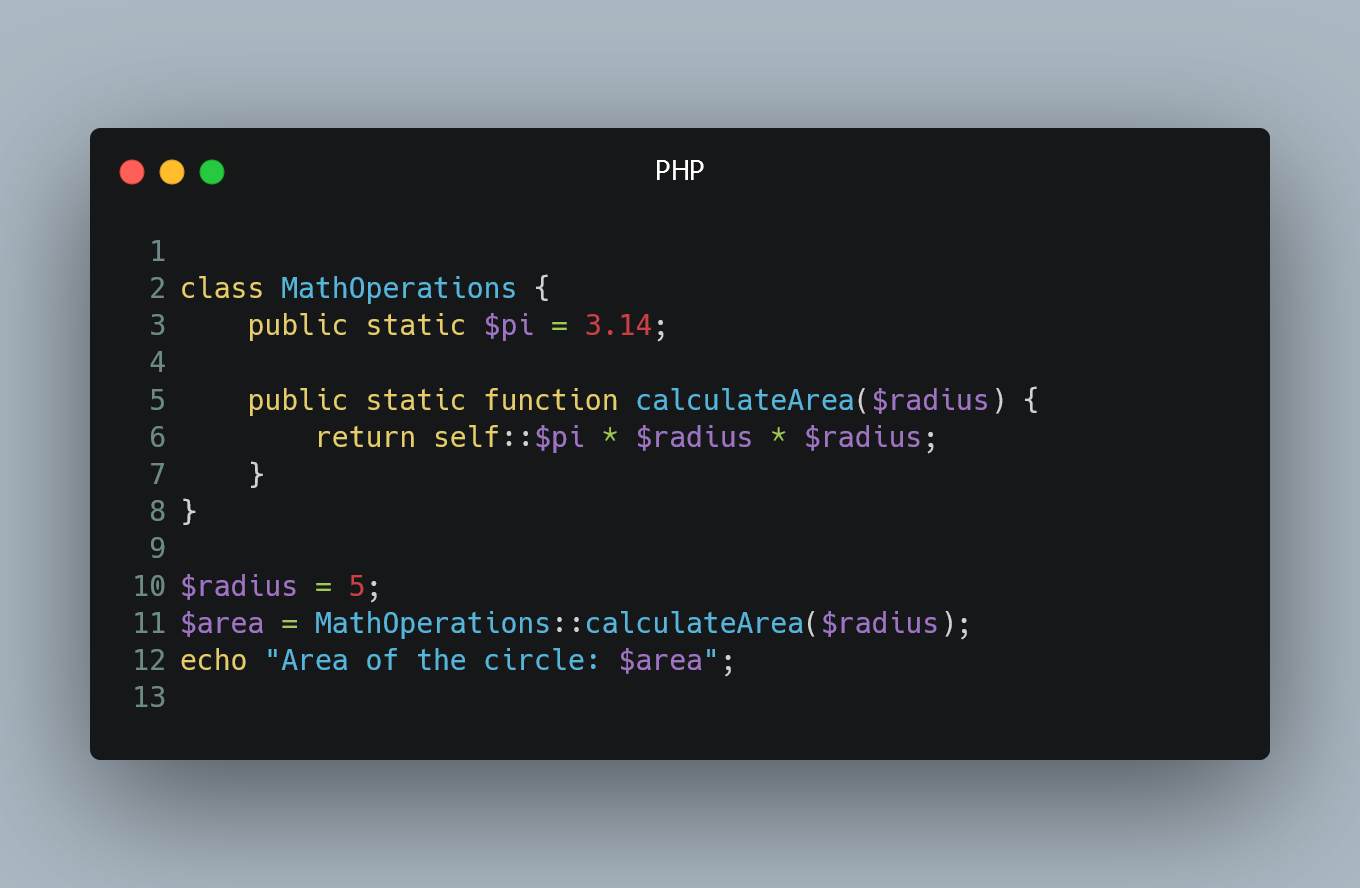
In PHP, both 'self' and '$this' are used to refer to class members, but they have different contexts and usages.
Using 'self'
What is 'self'?
'self' is a keyword in PHP that refers to the current class itself. It is mainly used within the class to access its own static properties and methods.
When to use 'self'
'self' should be used when accessing static properties or calling static methods from within the class definition.
Example
class MathOperations {
public static $pi = 3.14;
public static function calculateArea($radius) {
return self::$pi * $radius * $radius;
}
}
$radius = 5;
$area = MathOperations::calculateArea($radius);
echo "Area of the circle: $area";
In this example, we use 'self' to access the static property 'pi' and call the static method 'calculateArea' within the 'MathOperations' class.
Using '$this'
What is '$this'?
'$this' is a special variable in PHP that refers to the current instance of the class. It is used to access non-static properties and call non-static methods within the class.
When to use '$this'
'$this' should be used when accessing non-static properties or calling non-static methods from within the class methods.
Example
class Person {
public $name;
public function greet() {
echo "Hello, my name is " . $this->name;
}
}
$person = new Person();
$person->name = "John";
$person->greet();
In this example, we use '$this' to access the 'name' property and call the 'greet' method within the 'Person' class instance.
0 Comment