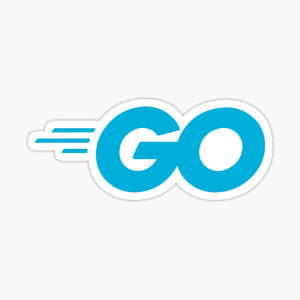
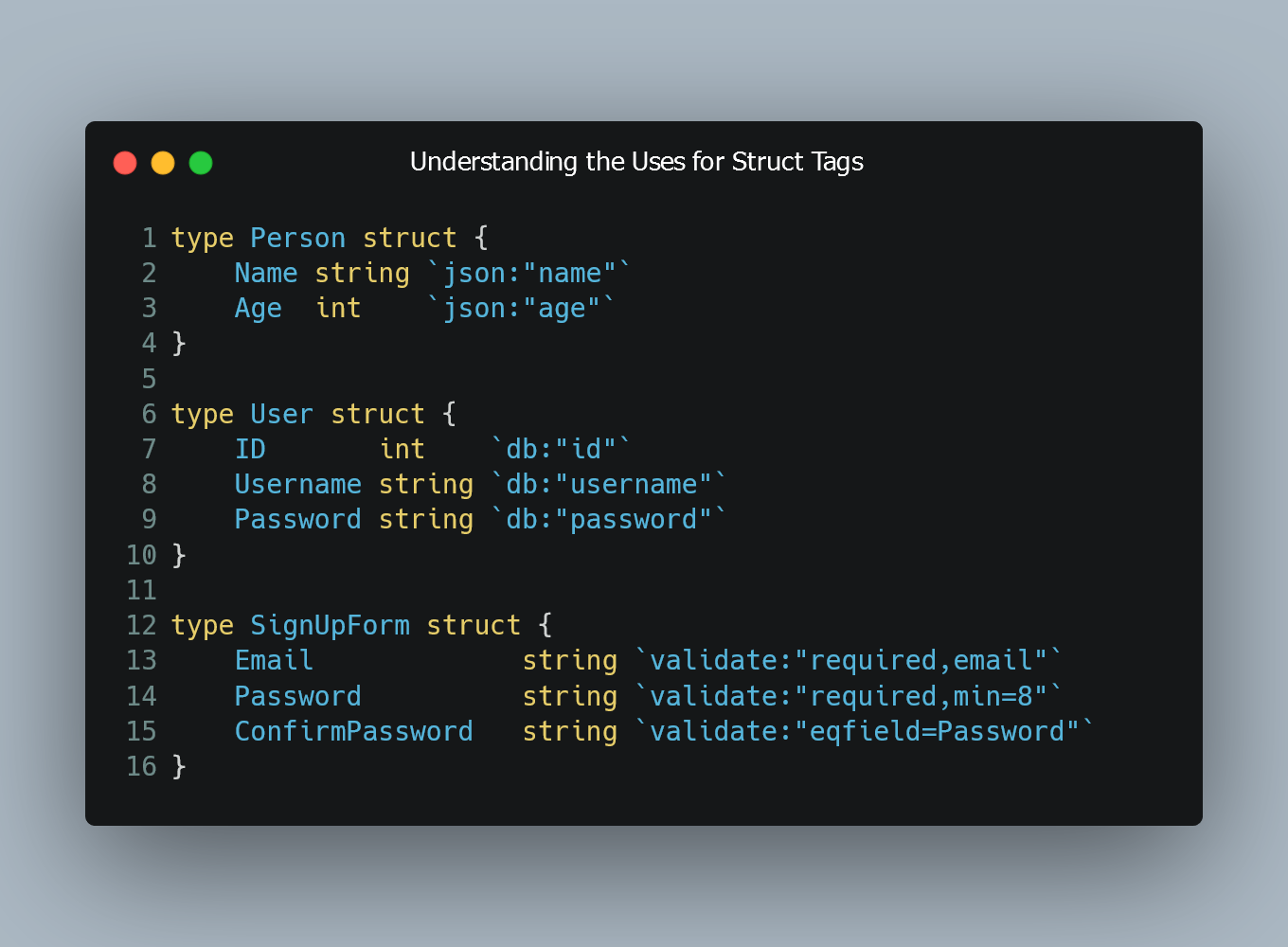
Struct tags are essential for many packages and are extensively used in libraries like encoding/json and database/sql. Struct tags are specified using backticks (``) immediately before the field declaration.
Serialization and Deserialization with encoding/json
One of the primary uses of struct tags is for controlling the serialization and deserialization of data structures, particularly with JSON. For instance, a struct field can be annotated with metadata to specify the JSON key it should map to when serialized or deserialized.
Example
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
}
In this example, when an instance of Person
is serialized to JSON, the Name
field will map to the JSON key name
, and Age
will map to age
.
Working with Database Operations in database/sql
Struct tags also play a pivotal role in database operations, specifically when we use ORMs or similar packages. They can define how struct fields map to database column names, which is particularly useful for performing CRUD operations.
Example
type User struct {
ID int `db:"id"`
Username string `db:"username"`
Password string `db:"password"`
}
In this instance, the User
struct maps to a database table where the columns are named id
, username
, and password
. When performing queries, these struct tags help translate the Go struct fields to their corresponding database columns.
Customizing Field Behavior with Validator Packages
Some Go packages allow developers to use struct tags for custom validations. The go-playground/validator
package, for example, enables developers to specify validation rules directly within struct tags.
Example
type SignUpForm struct {
Email string `validate:"required,email"`
Password string `validate:"required,min=8"`
ConfirmPassword string `validate:"eqfield=Password"`
}
In the SignUpForm
struct, the Email
field has two validation rules: it is required and must be a valid email address. The Password
field is also required and must be at least 8 characters long. The ConfirmPassword
field must be equal to the Password
field. These validation rules are defined directly in the struct tags, simplifying the validation process.
0 Comment