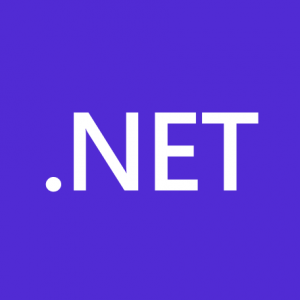
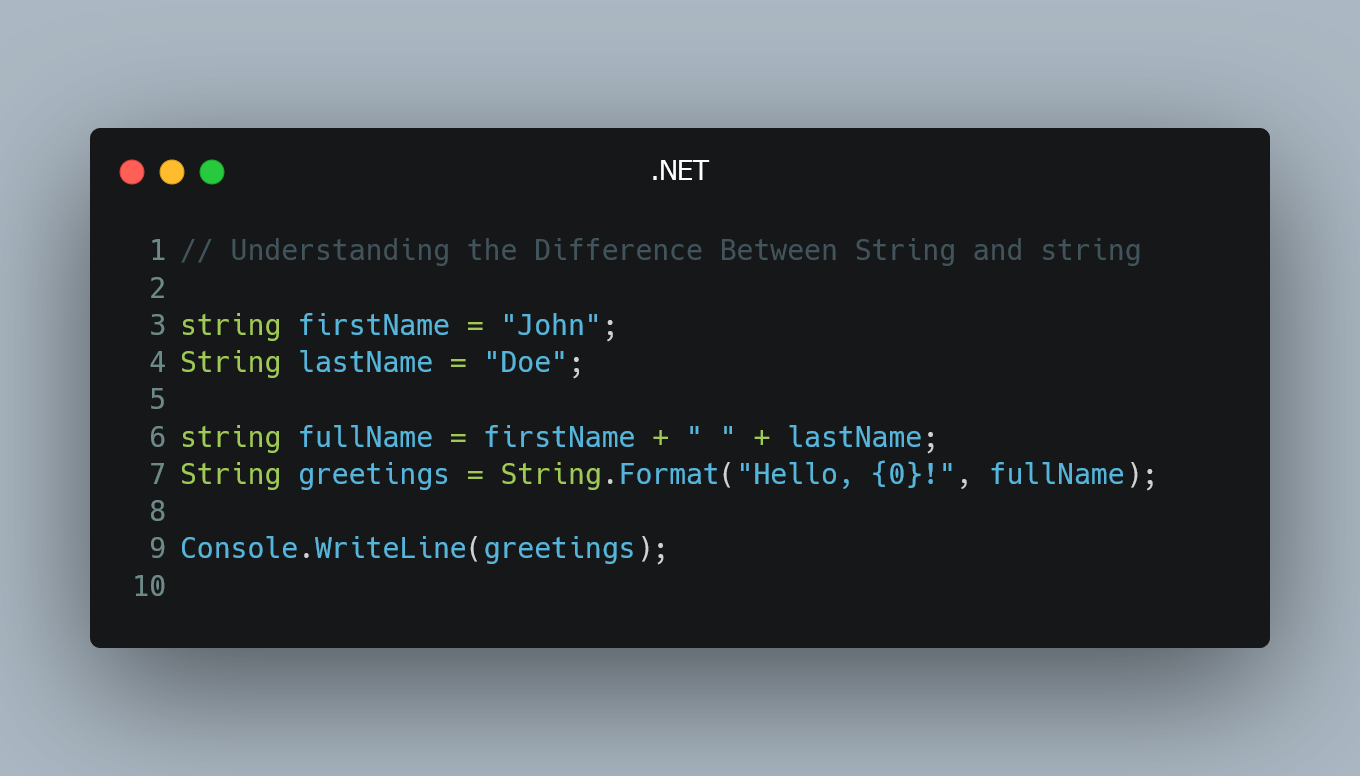
In C#, you might come across two similar-looking terms: "String" and "string." Although they might appear interchangeable, there is a distinction between the two.
String (System.String)
The term "String" with a capital 'S' represents a class in the .NET Framework's System namespace. It is a reference type and serves as the official string class provided by C#. The "String" class provides various methods and properties for working with strings, such as string concatenation, substring extraction, case manipulation, and more.
string (lowercase)
On the other hand, "string" with a lowercase 's' is an alias for the "String" class. It is a C# language keyword that provides a shorthand notation for creating instances of the "String" class. Both "String" and "string" are synonymous and refer to the same data type, representing a sequence of characters.
Usage
In practice, you can use "String" or "string" interchangeably based on your preference. However, the convention in C# programming is to use the lowercase "string" keyword for better code readability and consistency.
Example:
string firstName = "John";
String lastName = "Doe";
string fullName = firstName + " " + lastName;
String greetings = String.Format("Hello, {0}!", fullName);
Console.WriteLine(greetings);
In the above example, both "string" and "String" are used to declare variables and perform string concatenation.
0 Comment