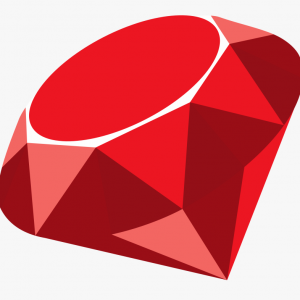
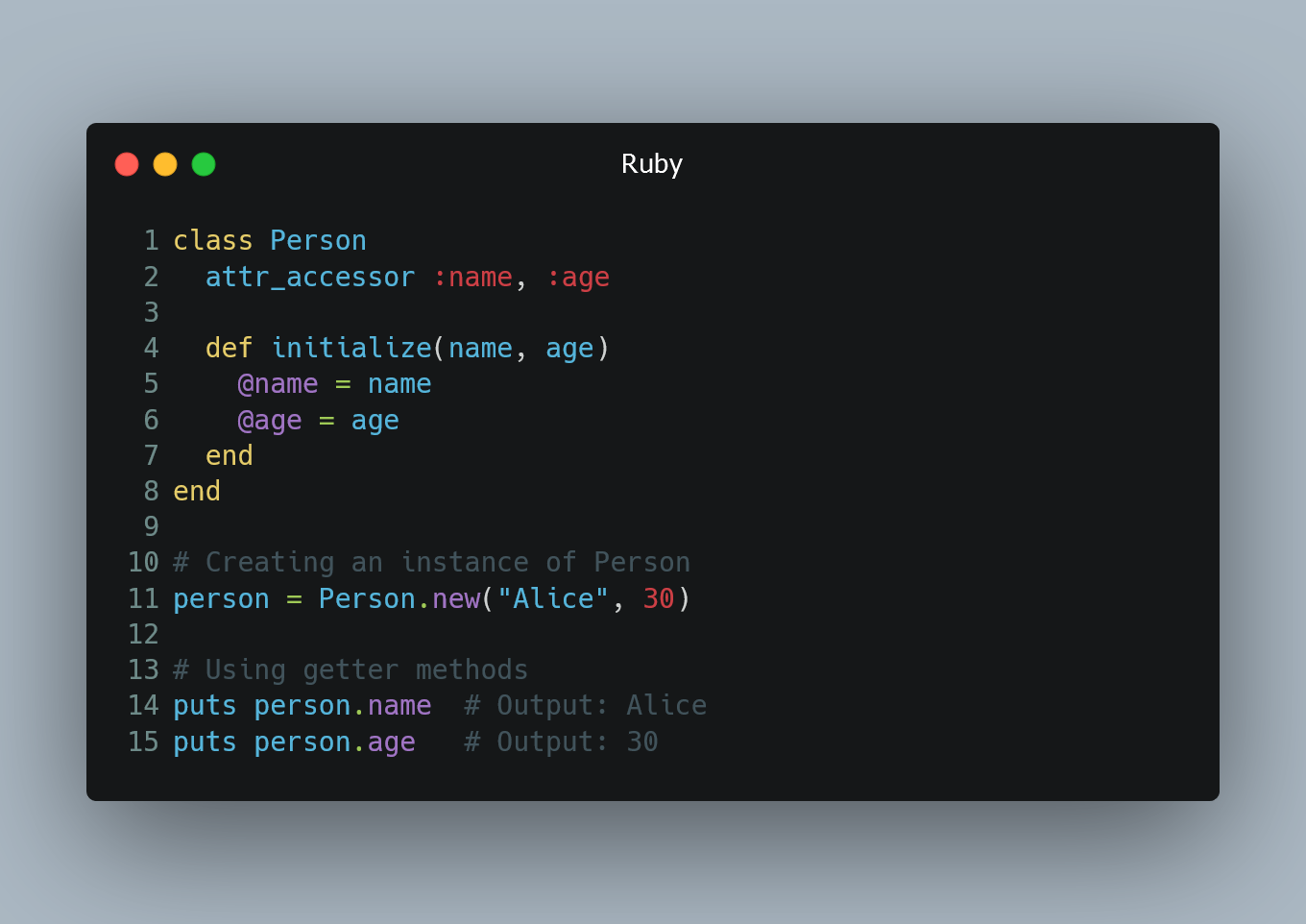
In Ruby, attr_accessor
is a convenient and commonly used method that provides a shortcut for defining getter and setter methods for instance variables in a class.
What is attr_accessor?
Overview
attr_accessor
is a Ruby method that automatically creates getter and setter methods for instance variables of a class. With attr_accessor
, you can read and write the values of instance variables without having to define explicit methods for each one.
Syntax
The syntax for using attr_accessor
is as follows:
class MyClass
attr_accessor :variable_name
end
Here, variable_name
is the name of the instance variable for which you want to create the getter and setter methods.
Example
class Person
attr_accessor :name, :age
def initialize(name, age)
@name = name
@age = age
end
end
# Creating an instance of Person
person = Person.new("Alice", 30)
# Using getter methods
puts person.name # Output: Alice
puts person.age # Output: 30
# Using setter methods
person.name = "Bob"
person.age = 25
puts person.name # Output: Bob
puts person.age # Output: 25
In this example, we define a class Person
with two instance variables name
and age
. By using attr_accessor
, we create getter and setter methods for these variables. We can then access and modify their values using the dot notation.
Benefits of Using attr_accessor
Using attr_accessor
offers several advantages:
- Conciseness: It reduces the amount of boilerplate code required to define getter and setter methods.
- Readability: It makes the code more readable by clearly indicating which instance variables are accessible from outside the class.
- Flexibility: It allows you to control read and write access to instance variables by using
attr_reader
(for only getters) orattr_writer
(for only setters) if needed.
0 Comment