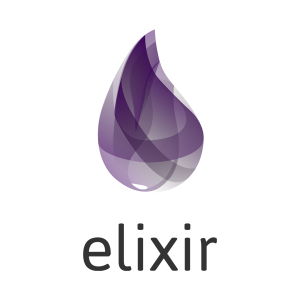
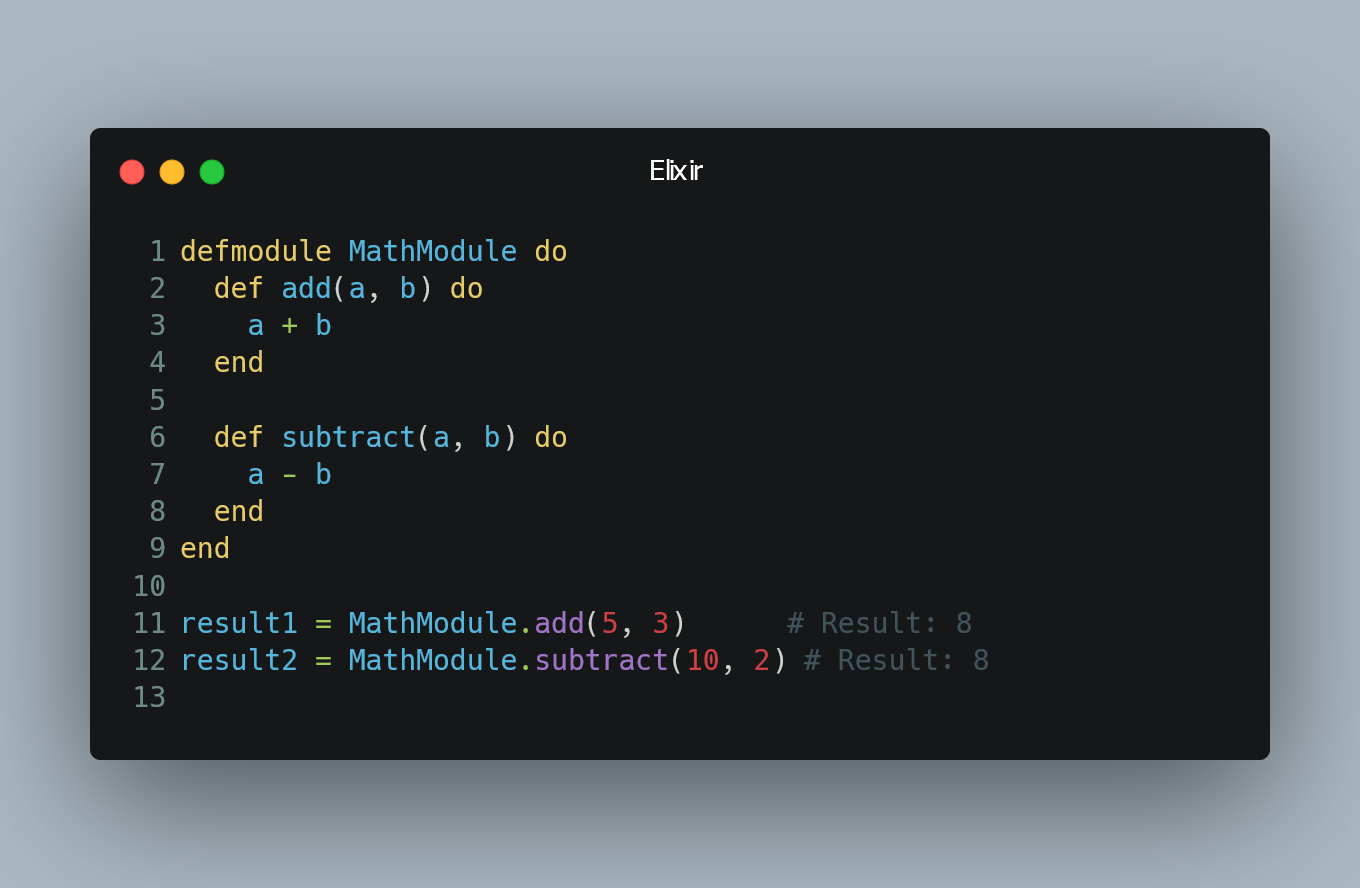
In Elixir, there are two main kinds of functions: named functions and anonymous functions.
Named Functions
Named functions in Elixir are defined with a name and can be called by that name from anywhere within the module where they are defined. They are typically used for tasks that need to be repeated or called multiple times in the code.
Example:
defmodule MathModule do
def add(a, b) do
a + b
end
def subtract(a, b) do
a - b
end
end
result1 = MathModule.add(5, 3) # Result: 8
result2 = MathModule.subtract(10, 2) # Result: 8
In this example, we define two named functions, add/2
and subtract/2
, inside the MathModule
module. These functions can be called by their names with the appropriate arguments to perform addition and subtraction operations.
Anonymous Functions
Anonymous functions in Elixir are defined using the fn
keyword and do not have a name. They are also known as "lambda functions" or "function literals." Anonymous functions are often used for more complex or specialized tasks, and they can be assigned to variables, passed as arguments to other functions, or returned from functions.
Example:
add = fn(a, b) -> a + b end
subtract = fn(a, b) -> a - b end
result1 = add.(5, 3) # Result: 8
result2 = subtract.(10, 2) # Result: 8
In this example, we define two anonymous functions, add
and subtract
, using the fn
keyword. These functions can be called using the dot notation and passing the required arguments, just like named functions.
Choosing Between Named and Anonymous Functions
When to use named functions or anonymous functions depends on the complexity and reusability of the code. Named functions are suitable for code blocks that need to be called multiple times and can help improve code readability. On the other hand, anonymous functions are more flexible and useful when you need to pass functions as arguments to other functions or return functions from functions.
0 Comment