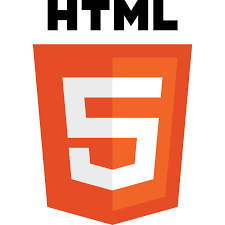
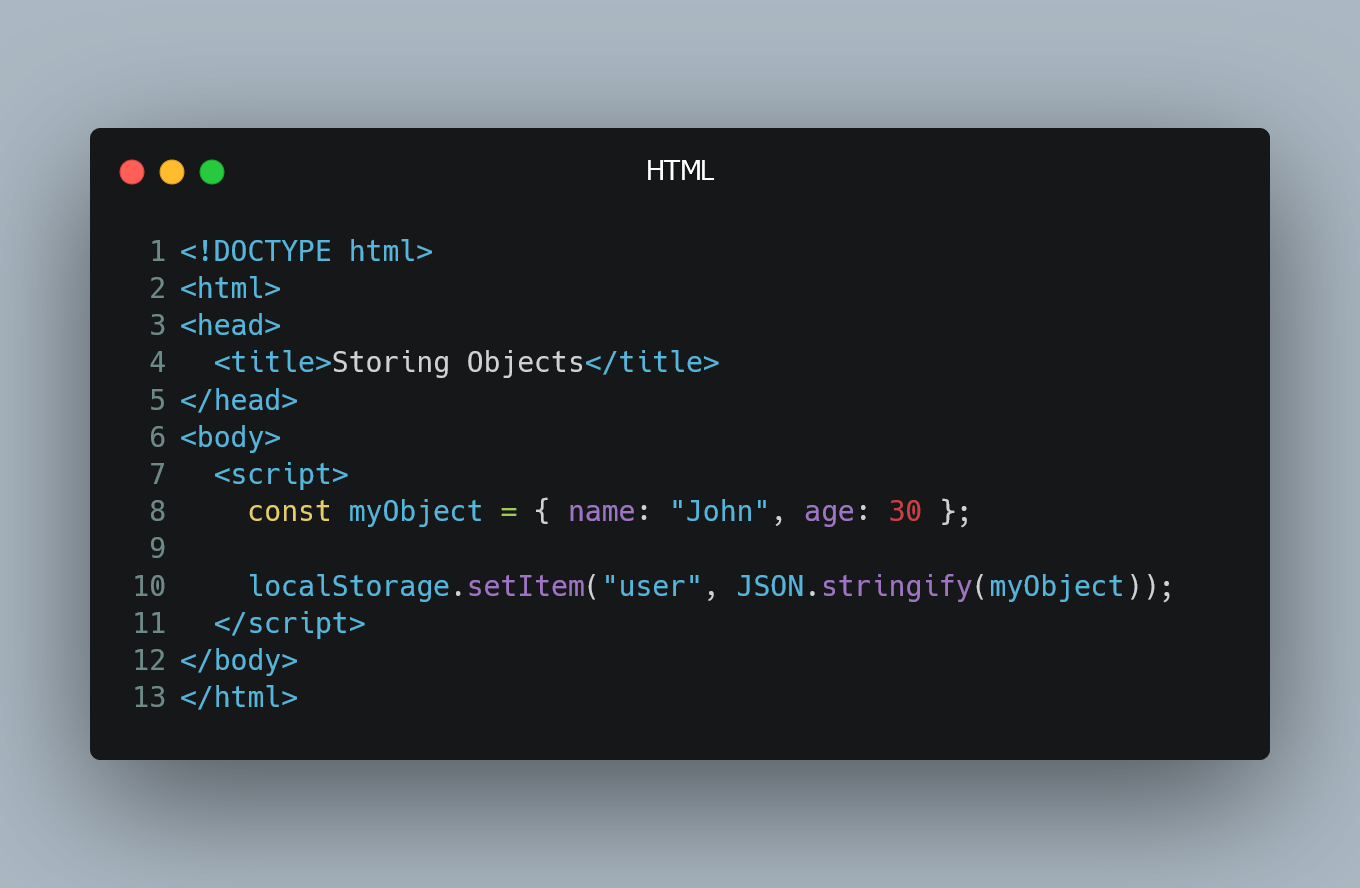
HTML5's localStorage
and sessionStorage
provide a convenient way to store key-value pairs in a web browser. While they are primarily designed to store strings, you can easily store and retrieve objects using JSON serialization.
#1. Storing an Object
To store an object in localStorage
or sessionStorage
, you need to convert the object into a JSON string using JSON.stringify()
.
<!DOCTYPE html>
<html>
<head>
<title>Storing Objects</title>
</head>
<body>
<script>
const myObject = { name: "John", age: 30 };
// Convert object to JSON string and store in localStorage
localStorage.setItem("user", JSON.stringify(myObject));
</script>
</body>
</html>
In this example, we create a JavaScript object myObject
with properties name
and age
. We then use JSON.stringify()
to convert the object to a JSON string and store it in localStorage
under the key "user"
.
#2. Retrieving an Object
To retrieve the stored object from localStorage
or sessionStorage
, you need to parse the JSON string back into a JavaScript object using JSON.parse()
.
<!DOCTYPE html>
<html>
<head>
<title>Retrieving Objects</title>
</head>
<body>
<script>
// Retrieve the JSON string from localStorage
const storedData = localStorage.getItem("user");
// Convert JSON string back to JavaScript object
const parsedData = JSON.parse(storedData);
// Access the properties of the object
console.log(parsedData.name); // Output: "John"
console.log(parsedData.age); // Output: 30
</script>
</body>
</html>
In this example, we first retrieve the JSON string from localStorage
using localStorage.getItem()
. We then use JSON.parse()
to convert the JSON string back to a JavaScript object, which we can access and use as normal.
#3. Using sessionStorage
The process is similar when using sessionStorage
, which works the same way as localStorage
, but with the key-value pairs only accessible within the same session or tab.
<!DOCTYPE html>
<html>
<head>
<title>Using sessionStorage</title>
</head>
<body>
<script>
const myObject = { name: "Jane", age: 25 };
// Convert object to JSON string and store in sessionStorage
sessionStorage.setItem("user", JSON.stringify(myObject));
// Retrieve and parse the object from sessionStorage
const storedData = sessionStorage.getItem("user");
const parsedData = JSON.parse(storedData);
// Access the properties of the object
console.log(parsedData.name); // Output: "Jane"
console.log(parsedData.age); // Output: 25
</script>
</body>
</html>
0 Comment