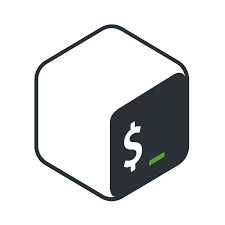
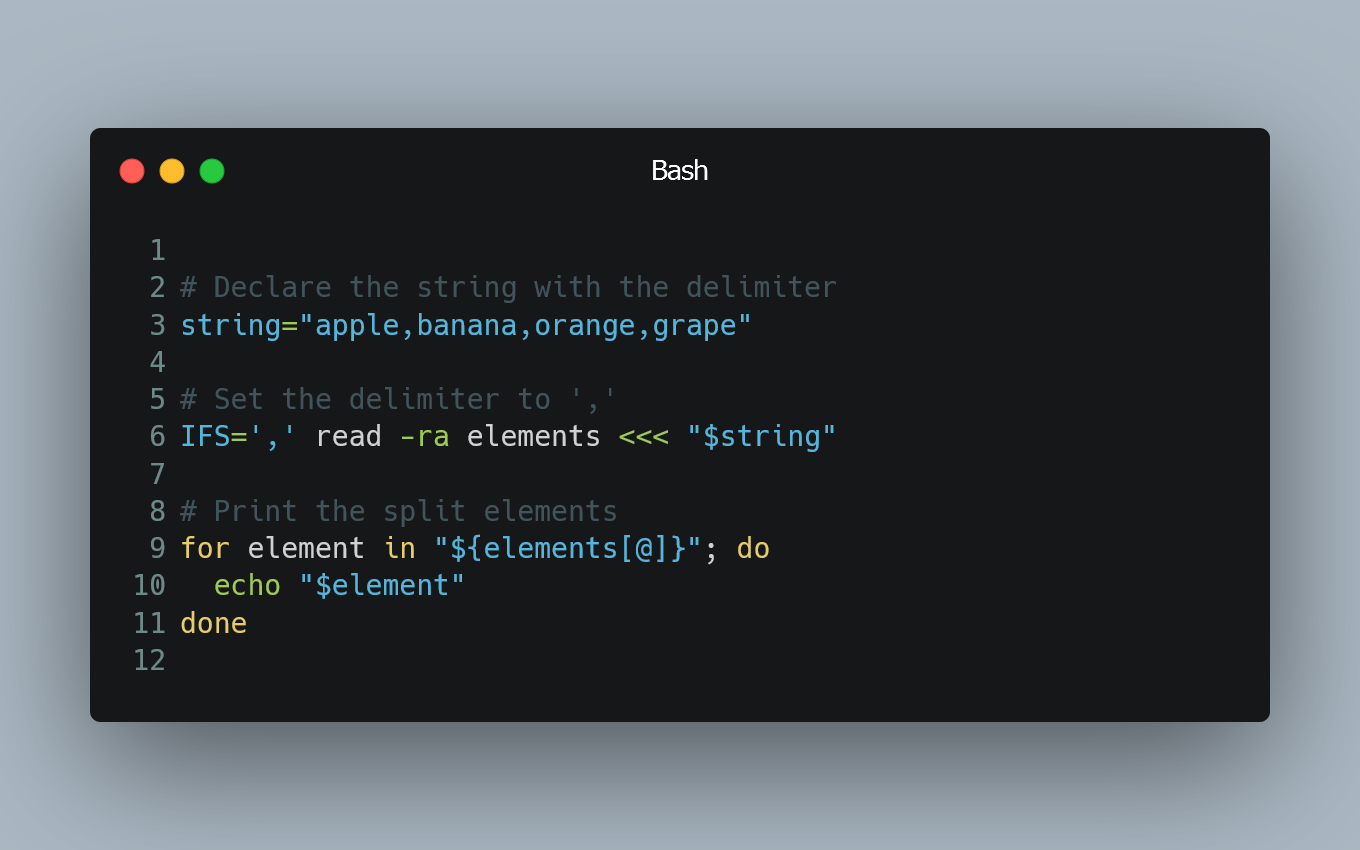
To split a string on a delimiter in Bash, you can use various approaches and built-in commands to achieve the desired result.
Using IFS
(Internal Field Separator)
The IFS
variable in Bash is the internal field separator, used by the shell to determine how to split strings into words. By modifying the value of IFS
, you can split a string on a specific delimiter.
# Declare the string with the delimiter
string="apple,banana,orange,grape"
# Set the delimiter to ','
IFS=',' read -ra elements <<< "$string"
# Print the split elements
for element in "${elements[@]}"; do
echo "$element"
done
Using cut
command
The cut
command is specifically designed to extract sections from lines of text.
# Declare the string with the delimiter
string="apple,banana,orange,grape"
# Use cut with ',' as the delimiter
echo "$string" | cut -d ',' -f 1
echo "$string" | cut -d ',' -f 2
echo "$string" | cut -d ',' -f 3
echo "$string" | cut -d ',' -f 4
Using awk
command
The awk
command is a versatile text processing tool that can split strings based on delimiters.
# Declare the string with the delimiter
string="apple,banana,orange,grape"
# Use awk with ',' as the delimiter
echo "$string" | awk -F ',' '{print $1}'
echo "$string" | awk -F ',' '{print $2}'
echo "$string" | awk -F ',' '{print $3}'
echo "$string" | awk -F ',' '{print $4}'
Using read
command
The read
command can be utilized to split a string into variables.
# Declare the string with the delimiter
string="apple,banana,orange,grape"
# Use read with ',' as the delimiter
IFS=',' read fruit1 fruit2 fruit3 fruit4 <<< "$string"
# Print the split elements
echo "$fruit1"
echo "$fruit2"
echo "$fruit3"
echo "$fruit4"
Using parameter expansion (substring removal)
You can also use parameter expansion to split a string based on a delimiter.
# Declare the string with the delimiter
string="apple,banana,orange,grape"
# Remove the suffix after each delimiter
fruit1="${string%%,*}"
string="${string#*,}"
fruit2="${string%%,*}"
string="${string#*,}"
fruit3="${string%%,*}"
string="${string#*,}"
fruit4="$string"
# Print the split elements
echo "$fruit1"
echo "$fruit2"
echo "$fruit3"
echo "$fruit4"
0 Comment