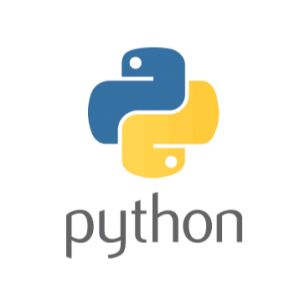
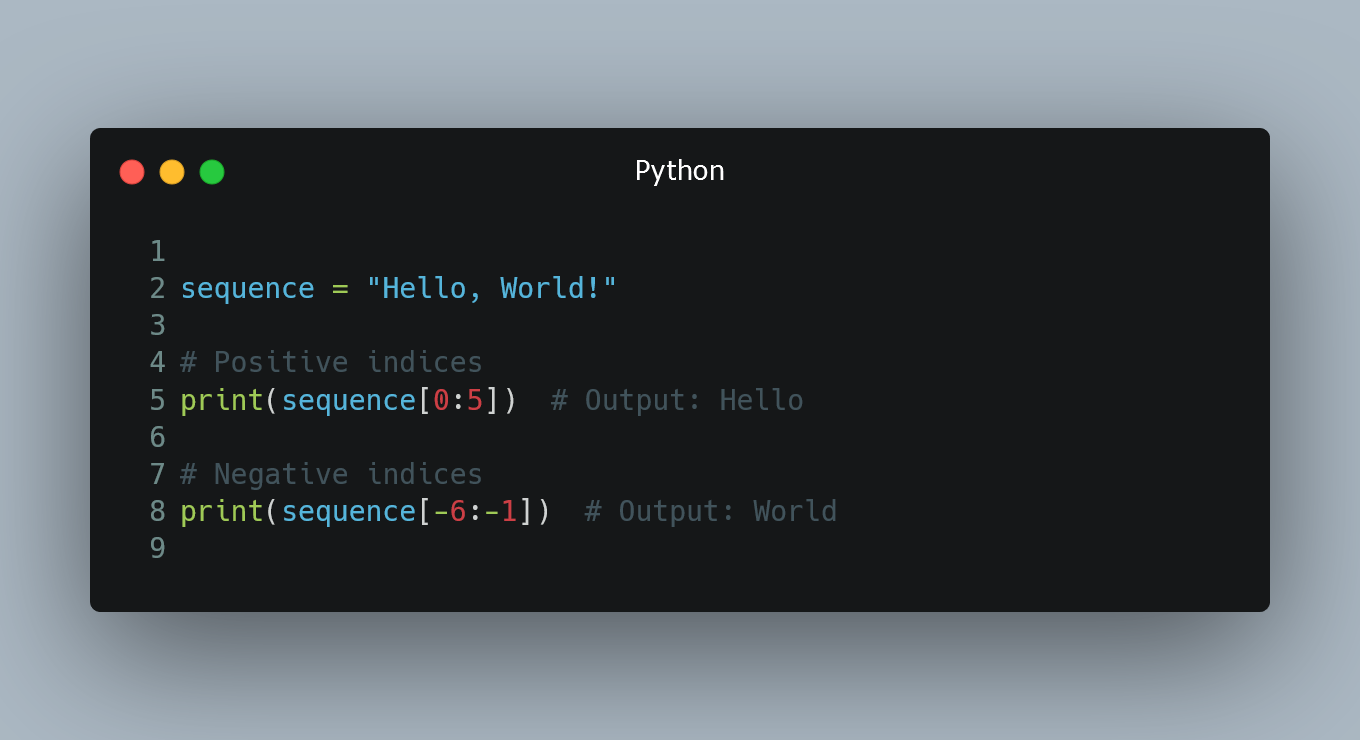
Slicing is a powerful and efficient way to extract specific elements from sequences like strings, lists, tuples, and other iterable objects in Python.
#1. Basic Syntax
The basic syntax of slicing involves using square brackets []
with a start index, stop index, and an optional step value:
sequence[start:stop:step]
- start: The index of the first element you want to include in the slice (inclusive).
- stop: The index of the first element you want to exclude from the slice (exclusive).
-
step: The interval between elements in the slice. It is optional, and the default value is
1
.
#2. Positive and Negative Indices
In Python, indices can be positive or negative. Positive indices start from the beginning of the sequence (0 being the first element), while negative indices start from the end of the sequence (-1 being the last element).
sequence = "Hello, World!"
# Positive indices
print(sequence[0:5]) # Output: Hello
# Negative indices
print(sequence[-6:-1]) # Output: World
#3. Slicing with Step
The step
value allows you to skip elements in the slice by specifying the interval between elements.
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
# Slice with step value 2 (every other element)
print(numbers[::2]) # Output: [0, 2, 4, 6, 8]
# Slice with step value -1 (reverse the list)
print(numbers[::-1]) # Output: [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
#4. Omitting Indices
If you omit the start
and stop
indices, the slice will include all elements from the beginning to the end of the sequence.
sequence = "Hello, World!"
# Slice all elements
print(sequence[:]) # Output: Hello, World!
#5. Modifying Slices
You can also use slicing to modify elements in the sequence.
numbers = [0, 1, 2, 3, 4, 5]
# Replace elements using slicing
numbers[2:4] = [10, 11]
print(numbers) # Output: [0, 1, 10, 11, 4, 5]
#6. Slicing Non-Continuous Sequences
Slicing allows you to extract non-continuous sequences by specifying a step value greater than 1.
sequence = "abcdefgh"
# Extract non-continuous sequence
print(sequence[::2]) # Output: aceg
0 Comment