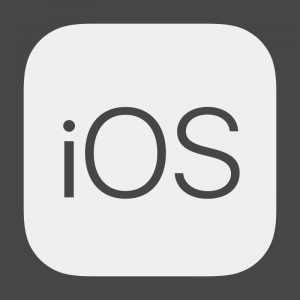
Published in
iOS
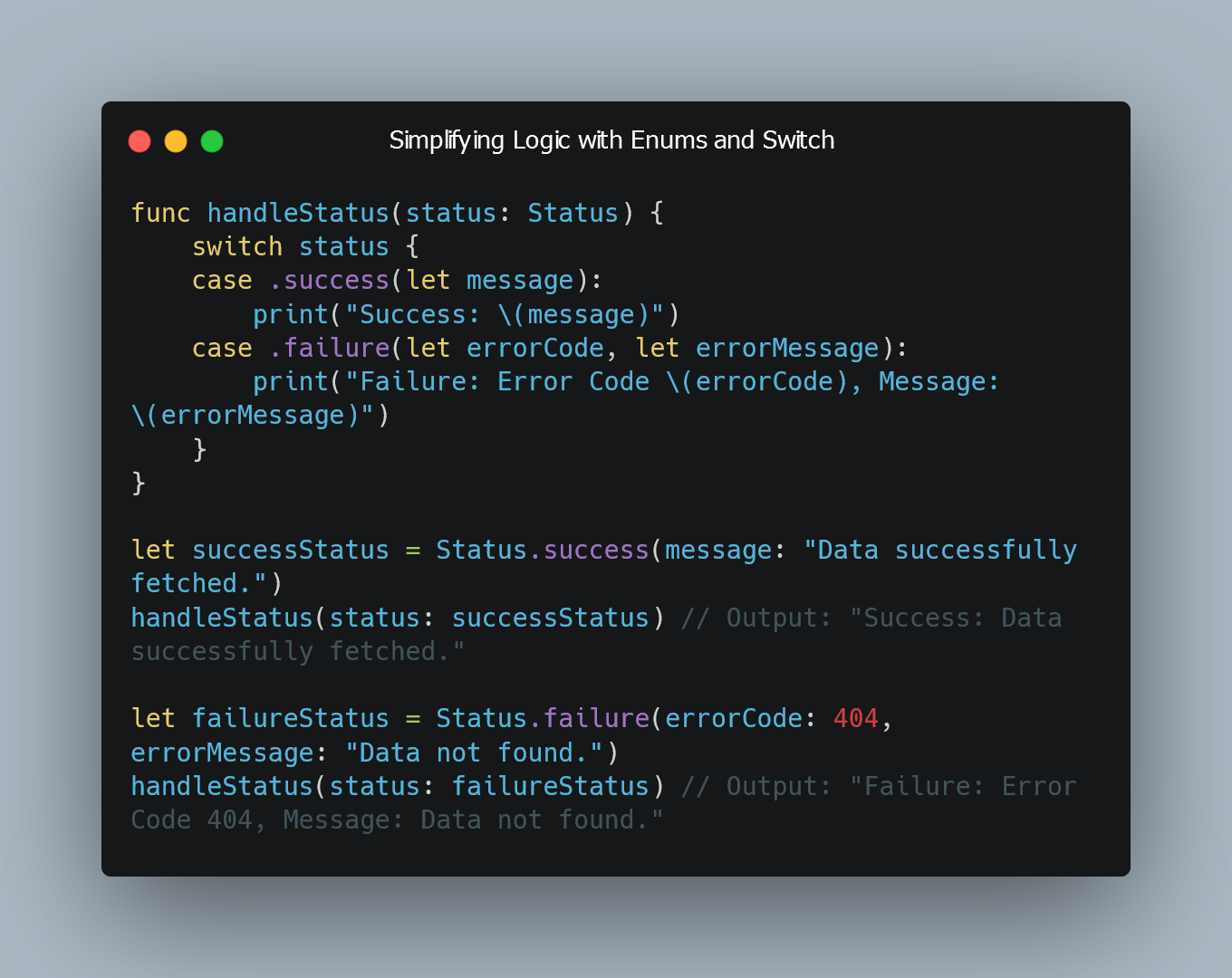
Enums are a powerful feature in Swift that allow you to define a group of related values. When combined with switch/case statements, enums can lead to cleaner, more organized, and more readable code.
Enum Definition
To define an enum in Swift, use the enum
keyword followed by the cases enclosed in curly braces.
enum Direction {
case north
case south
case east
case west
}
Switch/Case with Enums
The switch/case statement in Swift is an excellent fit for working with enums. It allows you to handle different cases based on the enum's value.
Basic Switch/Case with Enums
enum Direction {
case north
case south
case east
case west
}
func getDirectionMessage(direction: Direction) -> String {
switch direction {
case .north:
return "You are heading north."
case .south:
return "You are heading south."
case .east:
return "You are heading east."
case .west:
return "You are heading west."
}
}
let currentDirection = Direction.north
let message = getDirectionMessage(direction: currentDirection)
print(message) // Output: "You are heading north."
Associated Values with Enums
Enums can also have associated values, making them even more versatile.
enum Status {
case success(message: String)
case failure(errorCode: Int, errorMessage: String)
}
func handleStatus(status: Status) {
switch status {
case .success(let message):
print("Success: \(message)")
case .failure(let errorCode, let errorMessage):
print("Failure: Error Code \(errorCode), Message: \(errorMessage)")
}
}
let successStatus = Status.success(message: "Data successfully fetched.")
handleStatus(status: successStatus) // Output: "Success: Data successfully fetched."
let failureStatus = Status.failure(errorCode: 404, errorMessage: "Data not found.")
handleStatus(status: failureStatus) // Output: "Failure: Error Code 404, Message: Data not found."
0 Comment