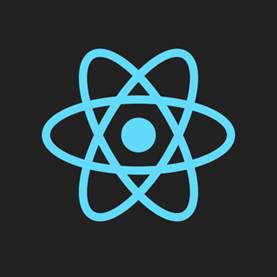
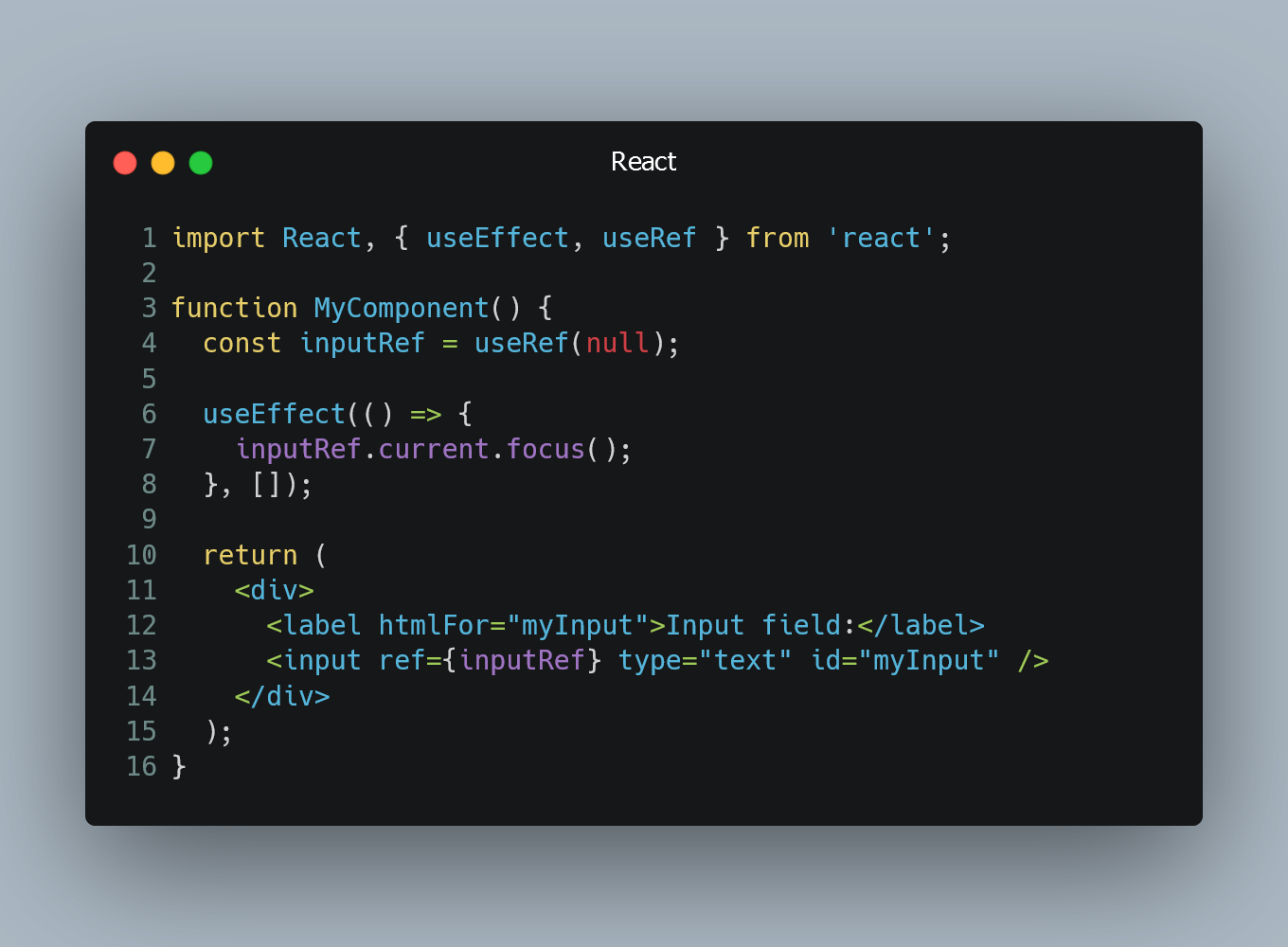
In React, you can programmatically set the focus on an input field after it has been rendered on the screen.
#1. Using the useRef
Hook
The useRef
hook allows you to create a reference to a DOM element. By using this reference, you can access the DOM element and set focus on it after rendering.
import React, { useEffect, useRef } from 'react';
function MyComponent() {
const inputRef = useRef(null);
useEffect(() => {
// Focus on the input field after rendering
inputRef.current.focus();
}, []);
return (
<div>
<label htmlFor="myInput">Input field:</label>
<input ref={inputRef} type="text" id="myInput" />
</div>
);
}
In this example, we use the useRef
hook to create a reference called inputRef
. The useEffect
hook is used with an empty dependency array ([]
) to run the focus logic only once after the component has rendered. Within the useEffect
, we use inputRef.current.focus()
to set focus on the input field.
#2. Conditional Focus
You can also conditionally set focus based on certain conditions. For example, you might want to set focus on the input field only if a specific condition is met.
import React, { useEffect, useRef, useState } from 'react';
function MyComponent() {
const [showInput, setShowInput] = useState(false);
const inputRef = useRef(null);
useEffect(() => {
if (showInput) {
inputRef.current.focus();
}
}, [showInput]);
return (
<div>
<button onClick={() => setShowInput(true)}>Show Input Field</button>
{showInput && (
<div>
<label htmlFor="myInput">Input field:</label>
<input ref={inputRef} type="text" id="myInput" />
</div>
)}
</div>
);
}
In this example, we use the state variable showInput
to control whether the input field should be displayed. The focus will be set on the input field only when the showInput
state is true
.
0 Comment