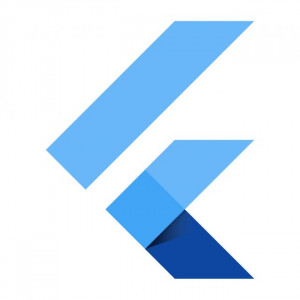
Published in
Flutter
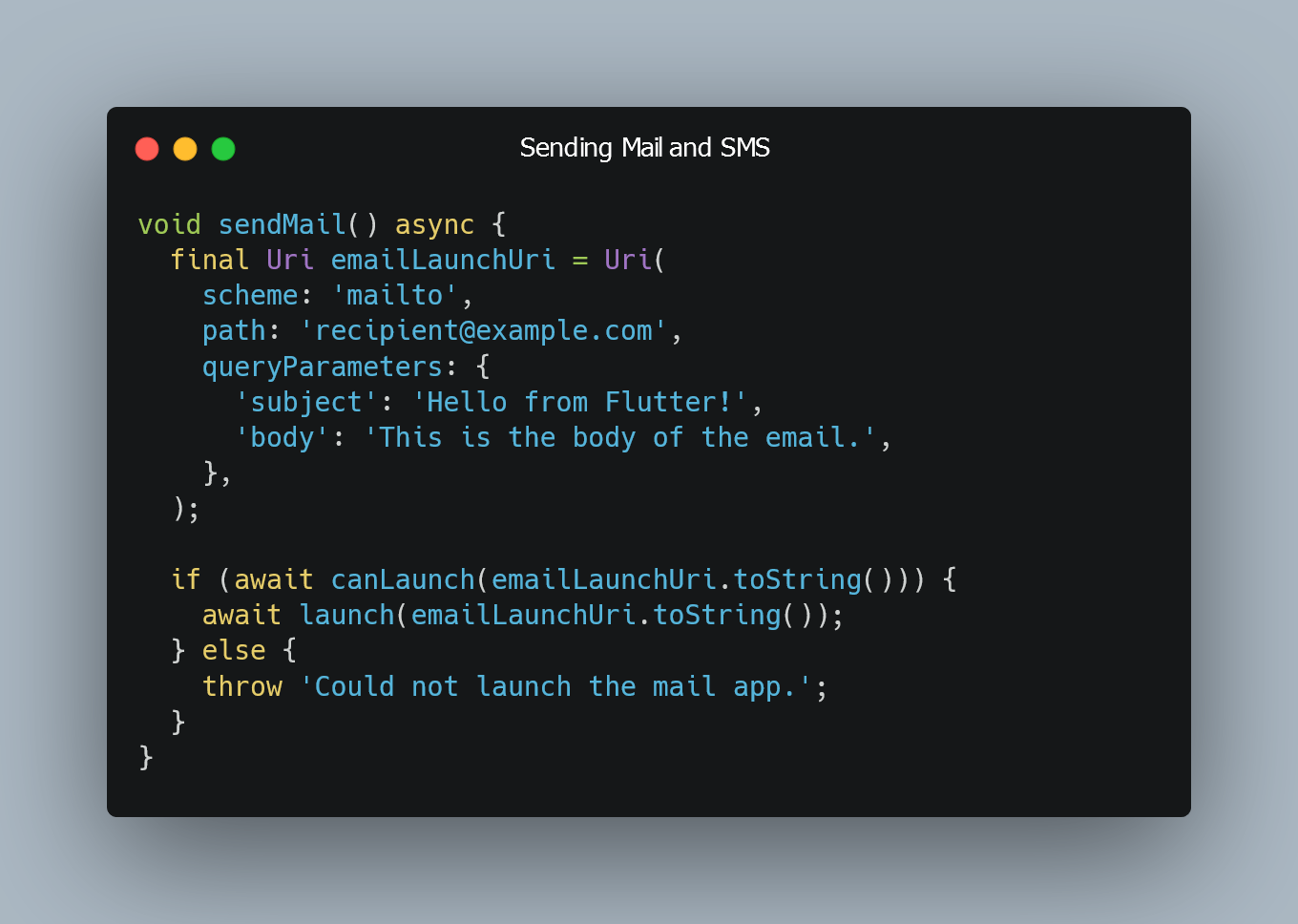
Flutter provides plugins that allow you to easily integrate mail and SMS functionalities into your app.
Mail Integration
Add the Dependency
In your pubspec.yaml
file, add the url_launcher
package to your dependencies:
dependencies:
flutter:
sdk: flutter
url_launcher: ^6.0.0
Import the Package
Import the url_launcher
package in your Dart file:
import 'package:url_launcher/url_launcher.dart';
Launch the Mail App
Use the launch
method from the url_launcher
package to open the mail app with pre-filled data:
void sendMail() async {
final Uri emailLaunchUri = Uri(
scheme: 'mailto',
path: 'recipient@example.com',
queryParameters: {
'subject': 'Hello from Flutter!',
'body': 'This is the body of the email.',
},
);
if (await canLaunch(emailLaunchUri.toString())) {
await launch(emailLaunchUri.toString());
} else {
throw 'Could not launch the mail app.';
}
}
SMS Integration
Add the Dependency
In your pubspec.yaml
file, add the flutter_sms
package to your dependencies:
dependencies:
flutter:
sdk: flutter
flutter_sms: ^2.1.0
Import the Package
Import the flutter_sms
package in your Dart file:
import 'package:flutter_sms/flutter_sms.dart';
Send the SMS
Use the sendSMS
method from the flutter_sms
package to send an SMS:
void sendSMS() async {
List recipients = ['1234567890', '9876543210'];
String message = 'This is the message from Flutter!';
String _result = await sendSMS(message: message, recipients: recipients)
.catchError((onError) {
print(onError);
});
}
0 Comment