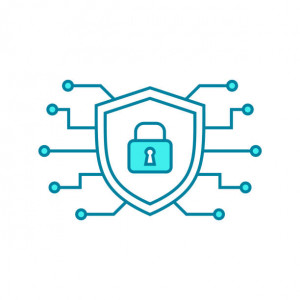
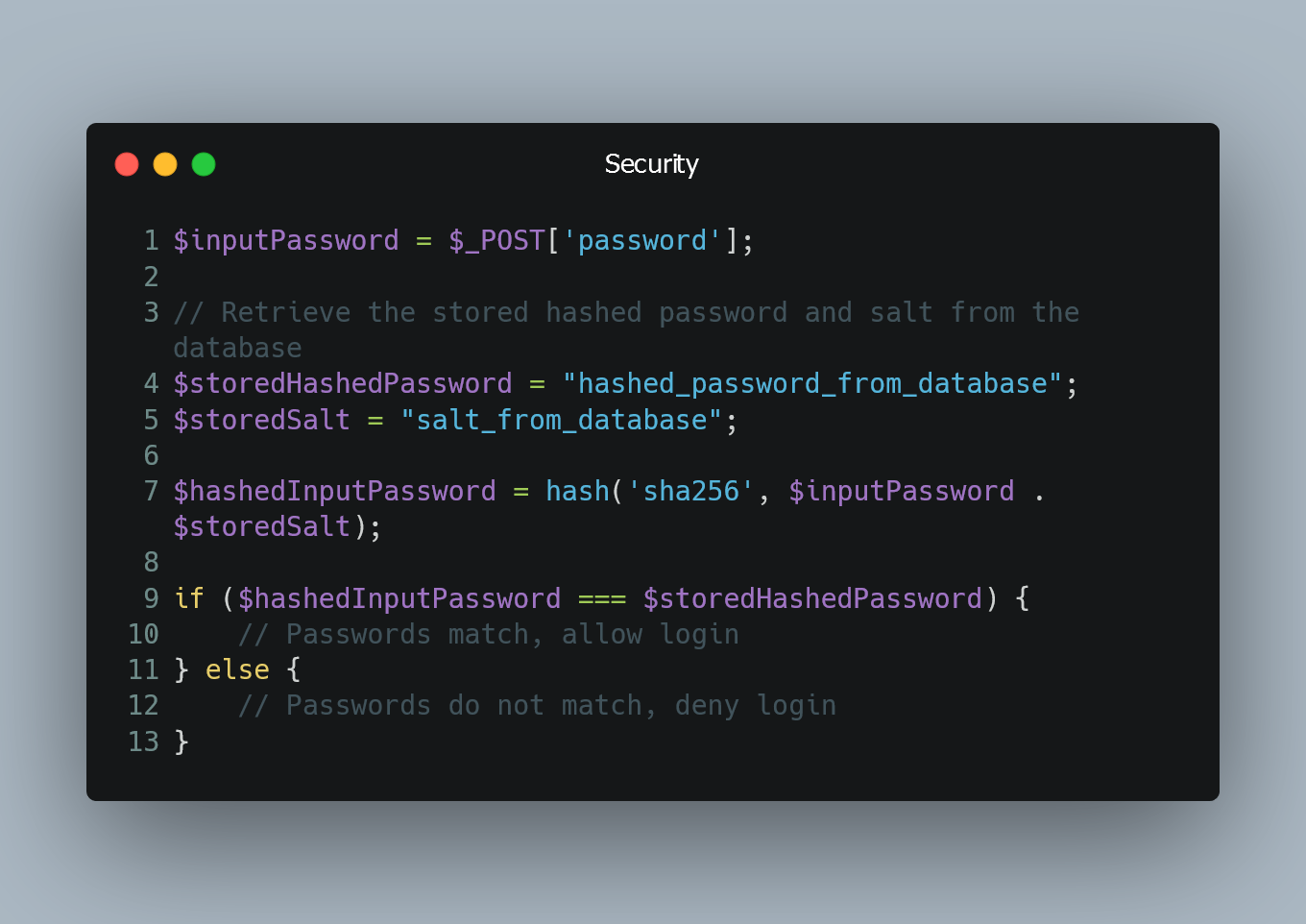
Securing user passwords is critical to protect user accounts from unauthorized access and data breaches. In PHP, using a strong hash function and salt is essential to enhance password security.
Hashing Passwords
Always hash user passwords before storing them in the database. Hashing is a one-way cryptographic function that converts the password into a fixed-length string of characters. PHP provides various hashing functions like password_hash()
for this purpose.
Example:
// Hashing Passwords
$password = $_POST['password'];
// Hash the password
$hashedPassword = password_hash($password, PASSWORD_DEFAULT);
// Store $hashedPassword in the database
Salting Passwords
A salt is random data added to the password before hashing. Salting prevents attackers from using precomputed tables (rainbow tables) to crack passwords. Each user should have a unique salt for added security.
Example:
// Salting Passwords
$password = $_POST['password'];
// Generate a random salt
$salt = bin2hex(random_bytes(16));
// Concatenate the salt with the password and hash it
$hashedPassword = hash('sha256', $password . $salt);
// Store $hashedPassword and $salt in the database
Using bcrypt
The bcrypt hashing algorithm is considered more secure for password hashing due to its adaptive nature and the ability to define the cost factor (work factor). PHP provides the password_hash()
function with the PASSWORD_BCRYPT
algorithm for bcrypt hashing.
Example:
// Using bcrypt
$password = $_POST['password'];
// Hash the password using bcrypt
$hashedPassword = password_hash($password, PASSWORD_BCRYPT);
// Store $hashedPassword in the database
Verifying Passwords
When verifying passwords during the login process, use the appropriate function (e.g., password_verify()
for bcrypt) to compare the stored hashed password with the user's input.
Example:
// Verifying Passwords
$inputPassword = $_POST['password'];
// Retrieve the stored hashed password and salt from the database
$storedHashedPassword = "hashed_password_from_database";
$storedSalt = "salt_from_database";
// Concatenate the salt with the input password and hash it for verification
$hashedInputPassword = hash('sha256', $inputPassword . $storedSalt);
// Compare the hashed input password with the stored hashed password
if ($hashedInputPassword === $storedHashedPassword) {
// Passwords match, allow login
} else {
// Passwords do not match, deny login
}
0 Comment