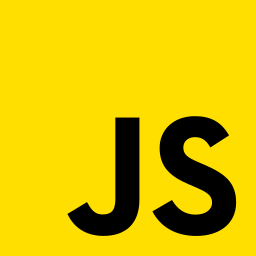
In JavaScript, you can easily remove a property from an object using different methods.
Using the delete Keyword
The simplest and most common way to remove a property from an object is by using the delete
keyword followed by the object's property name.
Example
const person = {
name: "John",
age: 30,
country: "USA"
};
delete person.age;
console.log(person); // Output: { name: "John", country: "USA" }
Using Object.assign()
Another method to remove a property from an object is by using Object.assign()
to create a new object without the unwanted property.
Example
const person = {
name: "John",
age: 30,
country: "USA"
};
const { age, ...newPerson } = person;
console.log(newPerson); // Output: { name: "John", country: "USA" }
In this example, we use object destructuring along with the spread operator (...
) to create a new object newPerson
without the age
property.
Using ES6 Destructuring
If you want to remove a property from an object and modify the object in-place, you can use ES6 destructuring assignment.
Example
const person = {
name: "John",
age: 30,
country: "USA"
};
const { age, ...newPerson } = person;
Object.assign(person, newPerson);
console.log(person); // Output: { name: "John", country: "USA" }
Using Lodash omit() Function
If you are using the popular utility library Lodash, you can use the omit()
function to remove specific properties from an object.
Example
const _ = require('lodash');
const person = {
name: "John",
age: 30,
country: "USA"
};
const newPerson = _.omit(person, 'age');
console.log(newPerson); // Output: { name: "John", country: "USA" }
0 Comment