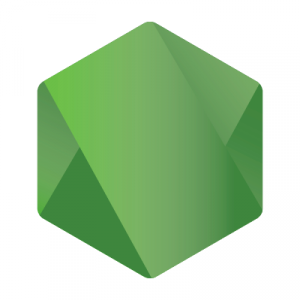
Environment variables are a powerful way to configure and control Node.js applications without hardcoding values in the code. They allow you to provide settings and sensitive information like API keys, database credentials, or configuration options externally.
Setting Environment Variables
Before reading environment variables, you need to set them. On different platforms, the method of setting environment variables can vary.
Windows
On Windows, you can set environment variables using the set
command in the Command Prompt or PowerShell:
set ENV_VARIABLE_NAME=value
Unix/Linux/macOS
On Unix-like systems, you can set environment variables using the export
command in the terminal:
export ENV_VARIABLE_NAME=value
Alternatively, you can set environment variables in a specific shell configuration file like .bashrc
, .bash_profile
, or .zshrc
, depending on the shell you are using.
Accessing Environment Variables in Node.js
Node.js provides access to environment variables through the process.env
object. To read an environment variable, simply access its value using process.env.VARIABLE_NAME
.
For example, if you have an environment variable named DATABASE_URL
, you can access it in your Node.js code like this:
const databaseUrl = process.env.DATABASE_URL;
console.log('Database URL:', databaseUrl);
Default Values for Environment Variables
In some cases, you may want to provide default values for environment variables to handle situations where they are not set. You can achieve this using the logical OR operator (||
).
const port = process.env.PORT || 3000;
console.log('Server Port:', port);
In this example, if the PORT
environment variable is not set, the port
variable will be assigned a default value of 3000
.
Using .env Files
For local development or testing, it is common to use a .env
file to set environment variables. Create a file named .env
in the root of your project and define your environment variables in this format:
ENV_VARIABLE_NAME=value
ANOTHER_ENV_VARIABLE=value2
To use the .env
file, you can utilize a package like dotenv
. Install it via npm:
npm install dotenv
Then, require and load it at the beginning of your entry file (e.g., index.js
):
require('dotenv').config();
Now, the variables defined in the .env
file will be available through process.env
in your Node.js application.
0 Comment