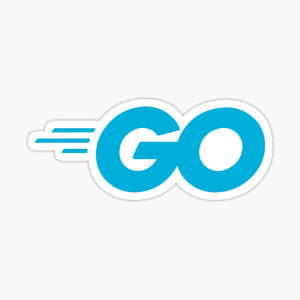
Published in
GO
To print the variables of a struct in the console in Go, you can use the fmt.Println()
or fmt.Printf()
functions from the fmt
package.
Using fmt.Println()
The fmt.Println()
function is a simple way to print the struct variables to the console.
Example
package main
import "fmt"
type Person struct {
Name string
Age int
Country string
}
func main() {
person := Person{
Name: "John",
Age: 30,
Country: "USA",
}
fmt.Println("Person:", person)
}
Using fmt.Printf() with %+v
If you need more control over the output or want to display the field names along with their values, you can use fmt.Printf()
with the %+v
verb.
Example
package main
import "fmt"
type Person struct {
Name string
Age int
Country string
}
func main() {
person := Person{
Name: "John",
Age: 30,
Country: "USA",
}
fmt.Printf("Name: %s, Age: %d, Country: %s\n", person.Name, person.Age, person.Country)
}
Using spew.Dump()
If you want a more detailed and formatted output, you can use a third-party package like spew
to print the struct variables.
Example
package main
import (
"github.com/davecgh/go-spew/spew"
)
type Person struct {
Name string
Age int
Country string
}
func main() {
person := Person{
Name: "John",
Age: 30,
Country: "USA",
}
spew.Dump(person)
}
0 Comment