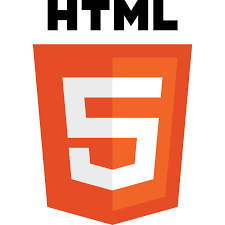
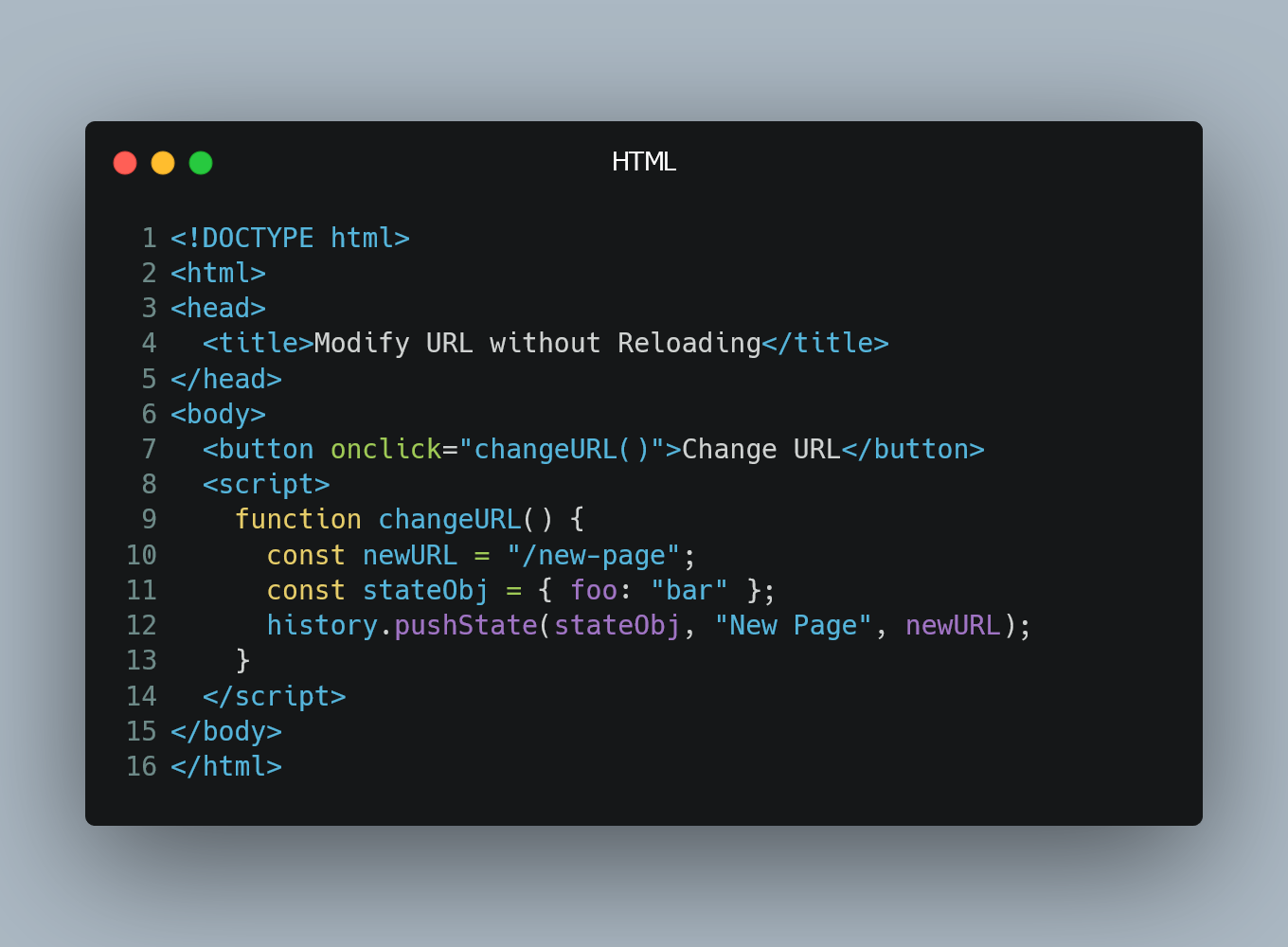
You can modify the URL of a web page without triggering a full page reload by using the HTML5 History API.
#1. Using pushState()
The pushState()
method of the History API allows you to add a new entry to the browser's history stack and modify the URL without reloading the page.
<!DOCTYPE html>
<html>
<head>
<title>Modify URL without Reloading</title>
</head>
<body>
<button onclick="changeURL()">Change URL</button>
<script>
// Function to modify the URL
function changeURL() {
const newURL = "/new-page"; // New URL to set
const stateObj = { foo: "bar" }; // Optional state object
// Use pushState() to modify the URL
history.pushState(stateObj, "New Page", newURL);
}
</script>
</body>
</html>
In this example, we have a button that triggers the JavaScript function changeURL()
when clicked. Inside the function, we use history.pushState()
to modify the URL without reloading the page. The new URL is "/new-page"
, and we can also provide an optional state object as the second parameter.
#2. Using replaceState()
The replaceState()
method of the History API is similar to pushState()
but replaces the current entry in the history stack instead of adding a new one.
<!DOCTYPE html>
<html>
<head>
<title>Modify URL without Reloading</title>
</head>
<body>
<button onclick="changeURL()">Change URL</button>
<script>
// Function to modify the URL
function changeURL() {
const newURL = "/new-page"; // New URL to set
const stateObj = { foo: "bar" }; // Optional state object
// Use replaceState() to modify the URL
history.replaceState(stateObj, "New Page", newURL);
}
</script>
</body>
</html>
In this example, the changeURL()
function uses history.replaceState()
to modify the URL, effectively replacing the current history entry with the new URL.
#3. Handling Popstate Event
When you modify the URL using the History API, you also need to handle the popstate
event to update the content of the page based on the new URL.
<!DOCTYPE html>
<html>
<head>
<title>Modify URL without Reloading</title>
</head>
<body>
<button onclick="changeURL()">Change URL</button>
<script>
// Function to modify the URL
function changeURL() {
const newURL = "/new-page"; // New URL to set
const stateObj = { foo: "bar" }; // Optional state object
// Use pushState() to modify the URL
history.pushState(stateObj, "New Page", newURL);
// Call a function to update the content based on the new URL
updateContent();
}
// Function to handle the popstate event
window.onpopstate = function(event) {
// Call a function to update the content based on the current URL
updateContent();
}
// Function to update the content based on the current URL
function updateContent() {
const currentURL = window.location.pathname;
// Add logic here to update the content based on the current URL
console.log("Updated content for URL: " + currentURL);
}
</script>
</body>
</html>
In this example, we add a function updateContent()
to handle updating the content of the page based on the current URL. We call this function both when the URL is changed using pushState()
and when the user navigates back or forward using the browser's navigation buttons.
0 Comment