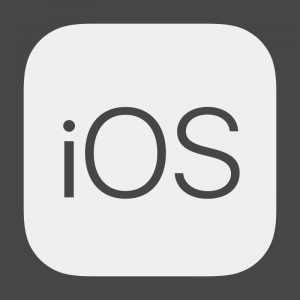
Switch/Case statements provide a clean and concise way to handle multiple conditions and are often used in place of long chains of if/else statements.
Basic Switch/Case Syntax
The basic structure of a Switch/Case statement in Swift looks like this:
switch expression {
case value1:
// Code block executed when expression matches value1
case value2:
// Code block executed when expression matches value2
// Add more cases as needed...
default:
// Code block executed when none of the cases match the expression
}
Matching on Constants
You can use a variety of data types as the expression, including integers, characters, strings, and enumerations. For instance, to match on constants:
let dayOfWeek = 3
switch dayOfWeek {
case 1:
print("Sunday")
case 2:
print("Monday")
case 3:
print("Tuesday")
// Add more cases for other days...
default:
print("Invalid day")
}
Matching on Ranges
Switch/Case statements are not limited to matching single values; they can also match ranges of values:
let score = 85
switch score {
case 0..<60:
print("You failed.")
case 60..<70:
print("You passed with a D.")
case 70..<80:
print("You passed with a C.")
case 80..<90:
print("You passed with a B.")
case 90...100:
print("Congratulations! You got an A!")
default:
print("Invalid score.")
}
Pattern Matching with Tuples
Tuples enable you to perform more complex pattern matching:
let point = (2, 2)
switch point {
case (0, 0):
print("Origin")
case (_, 0):
print("On the x-axis")
case (0, _):
print("On the y-axis")
default:
print("Somewhere in 2D space")
}
Where Clauses
You can use where
clauses to add additional conditions to your cases:
let age = 18
let isStudent = true
switch age {
case 0..<18:
print("You are a minor.")
case 18..<65 where isStudent == false:
print("You are an adult.")
case 18..<65 where isStudent == true:
print("You are an adult student.")
default:
print("You are a senior citizen.")
}
0 Comment