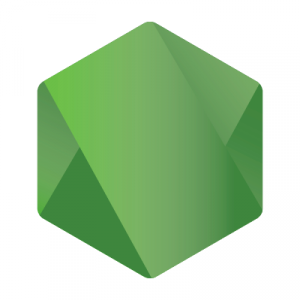
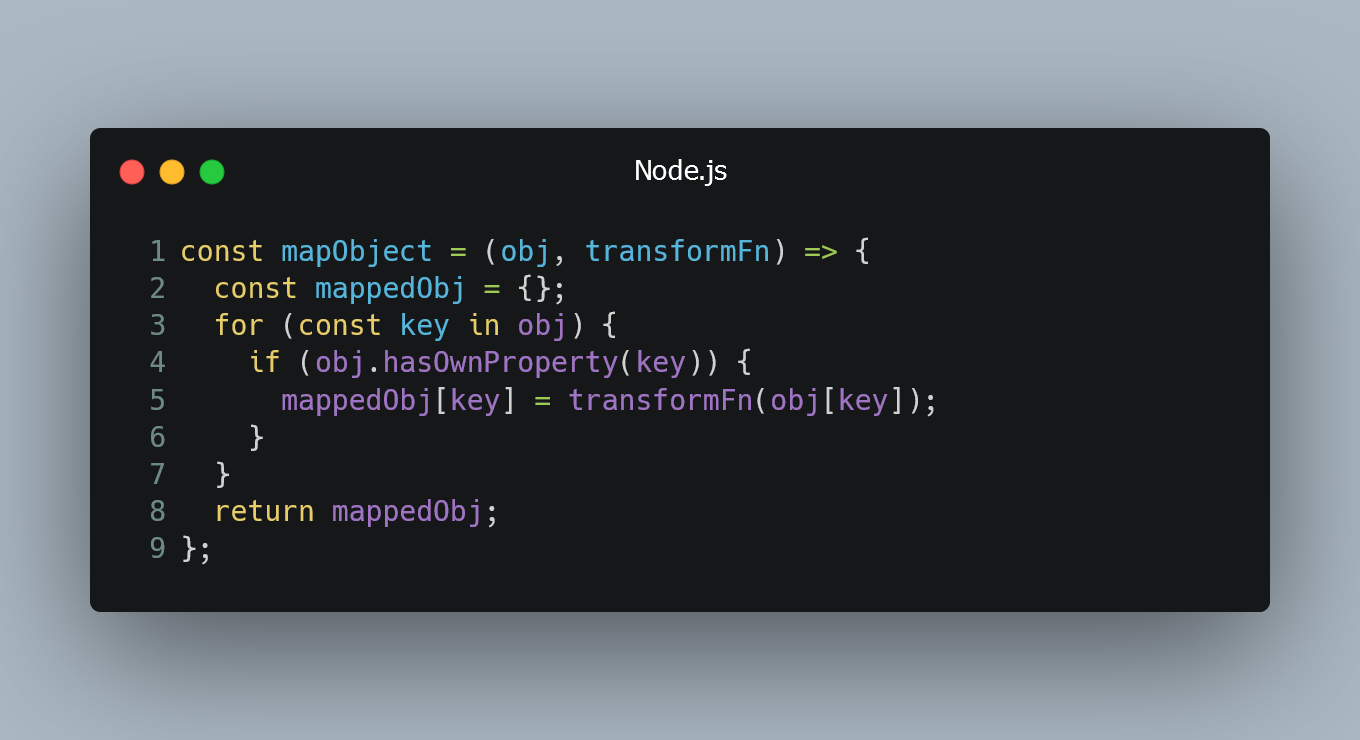
In JavaScript, the map
function is commonly used with arrays to transform elements and create new arrays based on the existing ones. However, there is no built-in map
function for objects.
Creating a Map Function for Objects
To create a map
function for objects, we can define a custom function that iterates over the object properties and applies a transformation function to each value. The resulting output will be a new object with the transformed properties.
const mapObject = (obj, transformFn) => {
const mappedObj = {};
for (const key in obj) {
if (obj.hasOwnProperty(key)) {
mappedObj[key] = transformFn(obj[key]);
}
}
return mappedObj;
};
In this implementation, the mapObject
function takes two parameters: the obj
(input object) and transformFn
(a function to transform each property value). It iterates over the keys of the input object using a for...in
loop and applies the transformFn
to each value, storing the result in the mappedObj
. Finally, it returns the new object with the transformed properties.
Using the Map Function for Objects
Let's see an example of using our custom mapObject
function to transform an object:
const originalObject = {
a: 1,
b: 2,
c: 3,
};
const transformFunction = (value) => value * 2;
const transformedObject = mapObject(originalObject, transformFunction);
console.log(transformedObject);
In this example, we have an originalObject
with three properties: a
, b
, and c
. We define a transformFunction
that doubles the value of each property. By calling mapObject(originalObject, transformFunction)
, we obtain a new transformedObject
where each property's value is doubled.
The output will be:
{
a: 2,
b: 4,
c: 6,
}
This demonstrates how we can use the custom map
function for objects to transform the values of an object using a specified transformation function.
0 Comment