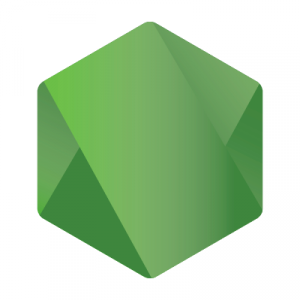
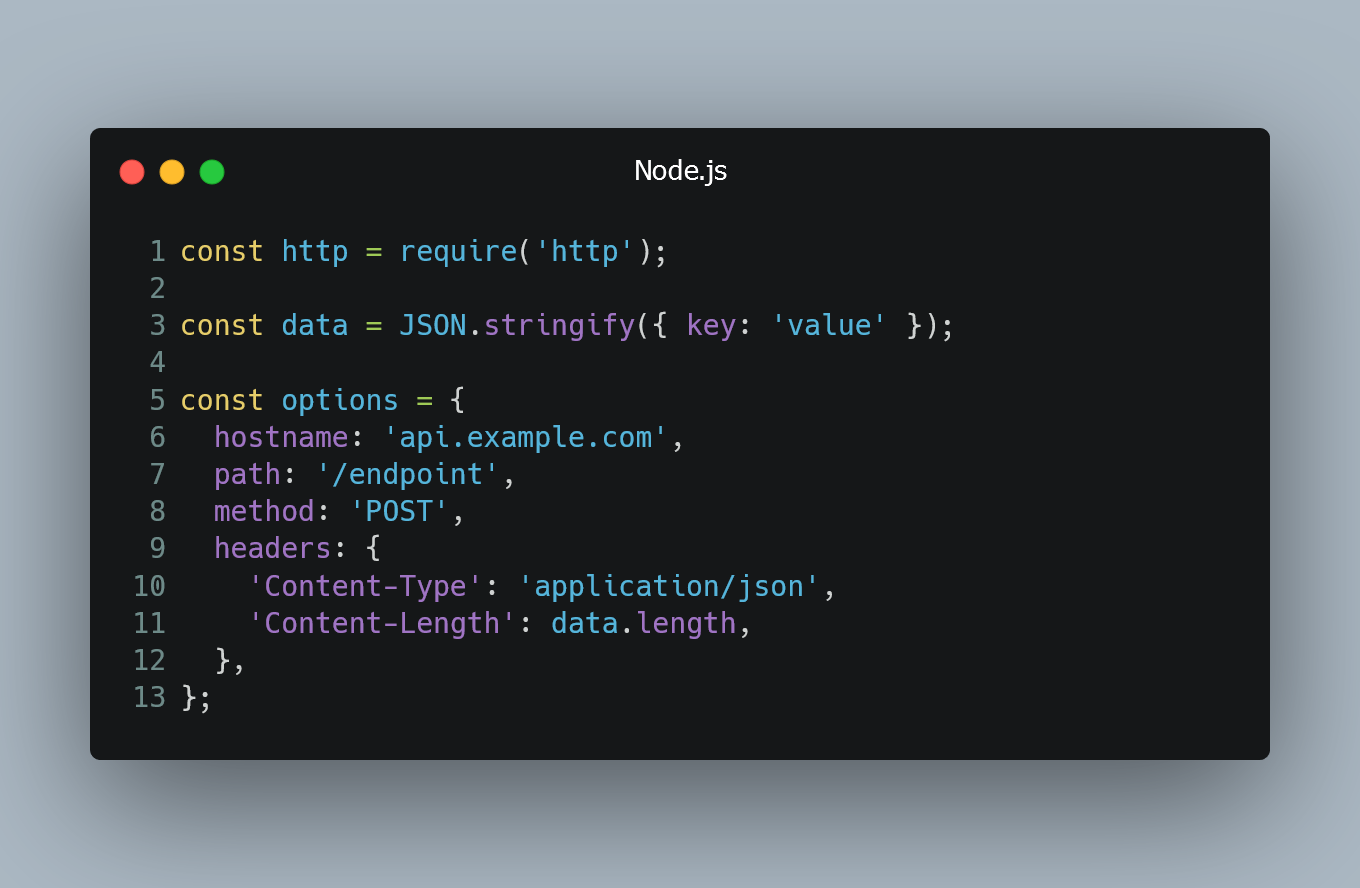
In Node.js, you can make an HTTP POST request to interact with APIs or send data to a server. HTTP POST requests are commonly used to create or update resources on the server.
Using the http
Module
The http
module in Node.js provides functionality for making HTTP requests. To make an HTTP POST request, follow these steps:
const http = require('http');
const data = JSON.stringify({ key: 'value' });
const options = {
hostname: 'api.example.com',
path: '/endpoint',
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Content-Length': data.length,
},
};
const req = http.request(options, (res) => {
let responseData = '';
res.on('data', (chunk) => {
responseData += chunk;
});
res.on('end', () => {
console.log('Response:', responseData);
});
});
req.on('error', (error) => {
console.error('Error:', error);
});
req.write(data);
req.end();
In this example, we first require the http
module and create an object, data
, containing the data to be sent in the request body. Next, we define the request options, including the target hostname
, path
, method
(which is 'POST'
for an HTTP POST request), and headers specifying the content type and content length.
We then use http.request()
to create the request. The response data is collected and concatenated in the responseData
variable. Once the response is complete, the data is logged to the console.
Using the https
Module
For secure communication over HTTPS, you can use the https
module in Node.js. The process is similar to making an HTTP POST request but with a few changes:
const https = require('https');
// Same data and options as the previous example
const req = https.request(options, (res) => {
let responseData = '';
res.on('data', (chunk) => {
responseData += chunk;
});
res.on('end', () => {
console.log('Response:', responseData);
});
});
req.on('error', (error) => {
console.error('Error:', error);
});
req.write(data);
req.end();
The code structure and request options remain the same as the http
example, but we have replaced the http
module with the https
module.
0 Comment