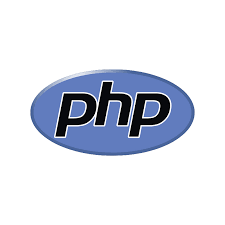
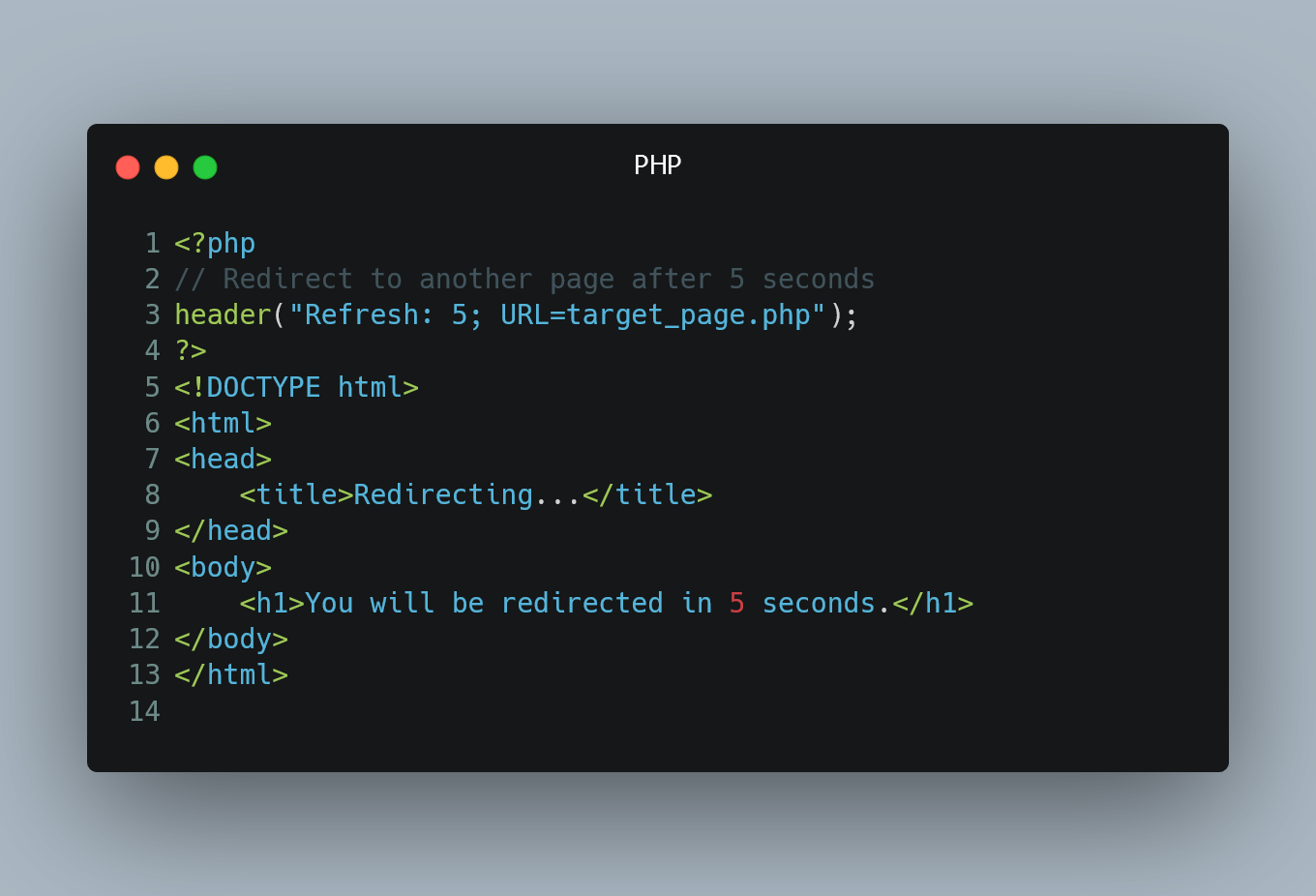
In PHP, you can use the header() function to perform a redirect to another page or URL.
Using the header() Function
The header() Function
The header() function is a built-in PHP function used to send HTTP headers to the browser. By setting the "Location" header, you can initiate a redirect.
Syntax of header()
header("Location: target_page.php");
exit;
The "Location" header specifies the target URL or page to which the user will be redirected.
Example
<?php
// Redirect to another page after 5 seconds
header("Refresh: 5; URL=target_page.php");
?>
<!DOCTYPE html>
<html>
<head>
<title>Redirecting...</title>
</head>
<body>
<h1>You will be redirected in 5 seconds.</h1>
</body>
</html>
In this example, the header() function is used with the "Refresh" header to redirect the user to "target_page.php" after 5 seconds. While waiting, the user will see the message "You will be redirected in 5 seconds." in the HTML page.
Redirecting immediately
If you want to redirect the user immediately without any delay, you can use the header() function as follows:
<?php
// Redirect immediately to another page
header("Location: target_page.php");
exit;
?>
In this case, the user will be redirected to "target_page.php" without any delay.
Important Note
- Make sure there is no output sent to the browser before using the header() function, as headers must be sent before any content.
- It is a good practice to include an exit; statement after the header() function to prevent further execution of the script.
0 Comment