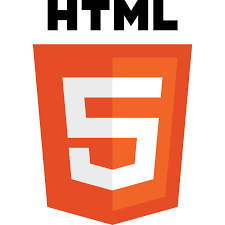
To prevent a <div>
from expanding beyond the size of its content, you can use CSS to control the dimensions and behavior of the container.
#1. Using "display: inline-block;"
One way to make a <div>
not larger than its contents is by setting its display property to inline-block
. This allows the <div>
to shrink-wrap around its content.
<!DOCTYPE html>
<html>
<head>
<title>Div Not Larger Than Contents</title>
<style>
/* Set the div to display as inline-block */
.inline-block-div {
display: inline-block;
border: 1px solid black; /* Just for illustration */
}
</style>
</head>
<body>
<div class="inline-block-div">
This div will not be larger than its contents.
</div>
</body>
</html>
In this example, the .inline-block-div
is styled with display: inline-block;
. This allows the <div>
to take up only the space required by its content and not expand beyond that.
#2. Using "display: inline;"
An alternative approach is to use the display: inline;
property. This behaves similarly to inline-block
, but with a subtle difference in how it handles vertical margins.
<!DOCTYPE html>
<html>
<head>
<title>Div Not Larger Than Contents</title>
<style>
/* Set the div to display as inline */
.inline-div {
display: inline;
border: 1px solid black; /* Just for illustration */
}
</style>
</head>
<body>
<div class="inline-div">
This div will not be larger than its contents.
</div>
</body>
</html>
In this example, the .inline-div
is styled with display: inline;
. This ensures that the <div>
shrinks to fit the content without expanding beyond the content's boundaries.
#3. Using "display: contents;"
If you want to keep the content of the <div>
flowing as if it were not wrapped by the <div>
, you can use display: contents;
. This property allows the content to be treated as if it were direct children of the parent's container, effectively removing the <div>
from the layout.
<!DOCTYPE html>
<html>
<head>
<title>Div Not Larger Than Contents</title>
<style>
/* Set the div to display as contents */
.contents-div {
display: contents;
}
</style>
</head>
<body>
<div class="contents-div">
This div will not affect the layout of its contents.
<p>Paragraph 1</p>
<p>Paragraph 2</p>
</div>
</body>
</html>
In this example, the .contents-div
is styled with display: contents;
, and the <div>
itself does not participate in the layout, allowing its contents to flow as if it were not there.
0 Comment