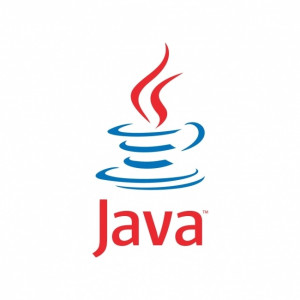
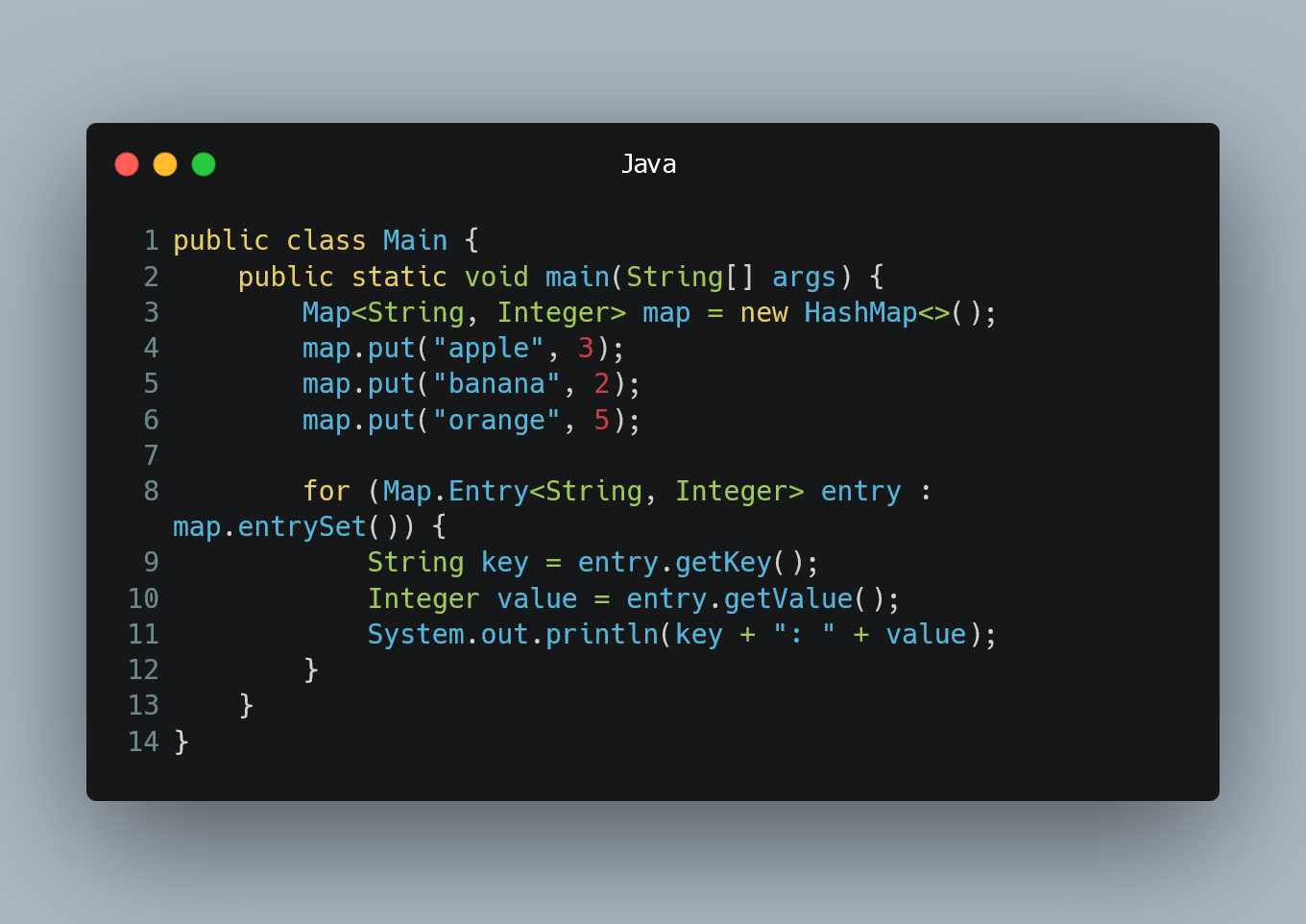
In Java, iterating through a HashMap allows you to access and process each key-value pair present in the map. Iterating is a common operation when working with maps, and there are various approaches you can take to achieve this.
Using entrySet()
One of the most efficient ways to iterate through a HashMap is by using the entrySet()
method, which returns a set of map entries, each containing both the key and the corresponding value.
Example:
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("apple", 3);
map.put("banana", 2);
map.put("orange", 5);
// Iterate through the HashMap using entrySet()
for (Map.Entry<String, Integer> entry : map.entrySet()) {
String key = entry.getKey();
Integer value = entry.getValue();
System.out.println(key + ": " + value);
}
}
}
In this example, we use the entrySet()
method to obtain a set of map entries, and then we use an enhanced for-loop to iterate through each entry and access the key and value using getKey()
and getValue()
methods.
Using keySet()
Alternatively, you can use the keySet()
method to obtain a set of keys and then access the corresponding values from the HashMap.
Example:
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("apple", 3);
map.put("banana", 2);
map.put("orange", 5);
// Iterate through the HashMap using keySet()
for (String key : map.keySet()) {
Integer value = map.get(key);
System.out.println(key + ": " + value);
}
}
}
In this example, we use the keySet()
method to obtain a set of keys, and then we use an enhanced for-loop to iterate through each key and access the corresponding value using get()
method.
Using forEach() (Java 8+)
If you are using Java 8 or later, you can utilize the forEach()
method with lambda expressions for a more concise way of iterating through the HashMap.
Example:
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("apple", 3);
map.put("banana", 2);
map.put("orange", 5);
// Iterate through the HashMap using forEach() and lambda expression
map.forEach((key, value) -> System.out.println(key + ": " + value));
}
}
In this Java 8 example, we use the forEach()
method with a lambda expression to directly print each key-value pair.
0 Comment