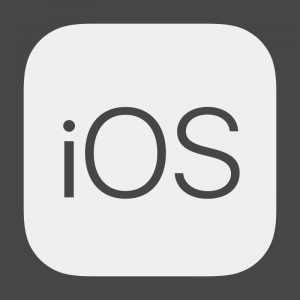
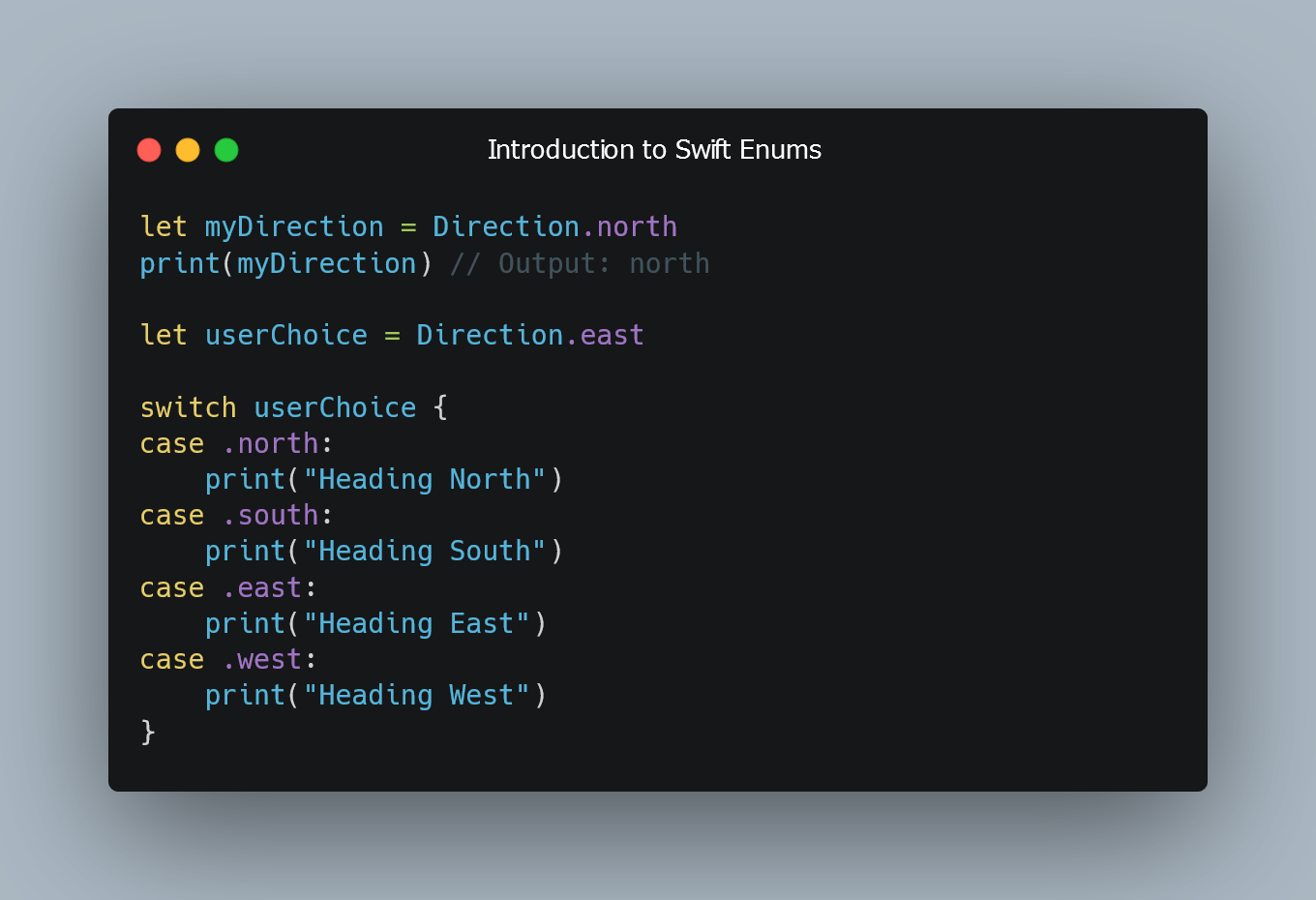
Swift Enums (Enumerations) are a powerful and flexible way to define a group of related values in a type-safe manner. They allow you to organize your code and represent data with distinct cases, making your code more readable and maintainable.
Defining an Enum
To create an enum, you define it with the enum
keyword followed by the name and a list of cases. Each case represents a distinct value the enum can hold.
enum Direction {
case north
case south
case east
case west
}
Using Enums
Accessing Enum Cases
You can access the individual cases of an enum using dot notation:
let myDirection = Direction.north
print(myDirection) // Output: north
Switch Statements with Enums
Switch statements are particularly useful with enums to handle different cases:
let userChoice = Direction.east
switch userChoice {
case .north:
print("Heading North")
case .south:
print("Heading South")
case .east:
print("Heading East")
case .west:
print("Heading West")
}
Associated Values
Enums can hold associated values, allowing them to carry additional data:
enum Weather {
case sunny
case rainy(Int) // The associated value is the rainfall in millimeters
case cloudy(WeatherType) // The associated value is another enum
}
enum WeatherType {
case cumulus
case stratus
case cirrus
}
let todayWeather = Weather.rainy(15)
Raw Values
You can assign raw values of the same type to each case, enabling easy conversion between raw values and enum values:
enum DayOfWeek: Int {
case sunday = 1
case monday
case tuesday
case wednesday
case thursday
case friday
case saturday
}
let today = DayOfWeek.wednesday
print(today.rawValue) // Output: 4
Enum Methods and Properties
You can also add methods and properties to enums, just like with other Swift types:
enum TrafficLight {
case red
case yellow
case green
var description: String {
switch self {
case .red:
return "Stop"
case .yellow:
return "Prepare to go"
case .green:
return "Go"
}
}
}
let currentLight = TrafficLight.green
print(currentLight.description) // Output: "Go"
0 Comment