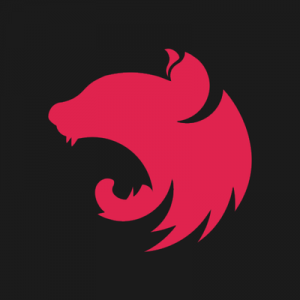
In NestJS, services are an essential part of the application, and they can be injected and used across different modules. To inject a NestJS service from another module, you need to ensure that the service is exported and that the module where it is provided is imported into the module where you want to use the service.
Creating the Service
First, you need to create the service that you want to inject. For example, let's create a simple service called ExampleService
.
// example.service.ts
import { Injectable } from '@nestjs/common';
@Injectable()
export class ExampleService {
getHello(): string {
return 'Hello from ExampleService!';
}
}
Providing the Service
Next, you need to provide the service within a module. This is typically done in the module's providers
array. Let's assume the service is provided in the ExampleModule
.
// example.module.ts
import { Module } from '@nestjs/common';
import { ExampleService } from './example.service';
@Module({
providers: [ExampleService],
exports: [ExampleService],
})
export class ExampleModule {}
In this example, we import the ExampleService
and add it to the providers
array. We also use the exports
array to make the service available for injection in other modules.
Importing the Module with the Service
To inject the ExampleService
from another module, you need to import the ExampleModule
that provides the service. Let's assume we want to use the ExampleService
in the AppModule
.
// app.module.ts
import { Module } from '@nestjs/common';
import { ExampleModule } from './example/example.module';
import { AppController } from './app.controller';
import { AppService } from './app.service';
@Module({
imports: [ExampleModule], // Import the ExampleModule here
controllers: [AppController],
providers: [AppService],
})
export class AppModule {}
Injecting the Service
Now that the ExampleService
is provided and exported from the ExampleModule
, you can inject it into any constructor in the AppModule
or any other module that imports ExampleModule
.
// app.service.ts
import { Injectable } from '@nestjs/common';
import { ExampleService } from './example/example.service';
@Injectable()
export class AppService {
constructor(private readonly exampleService: ExampleService) {}
getHello(): string {
return this.exampleService.getHello();
}
}
In this example, we import the ExampleService
from the ExampleModule
and inject it into the AppService
constructor. Now, we can use the methods provided by the ExampleService
within the AppService
.
0 Comment