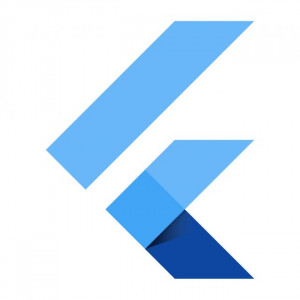
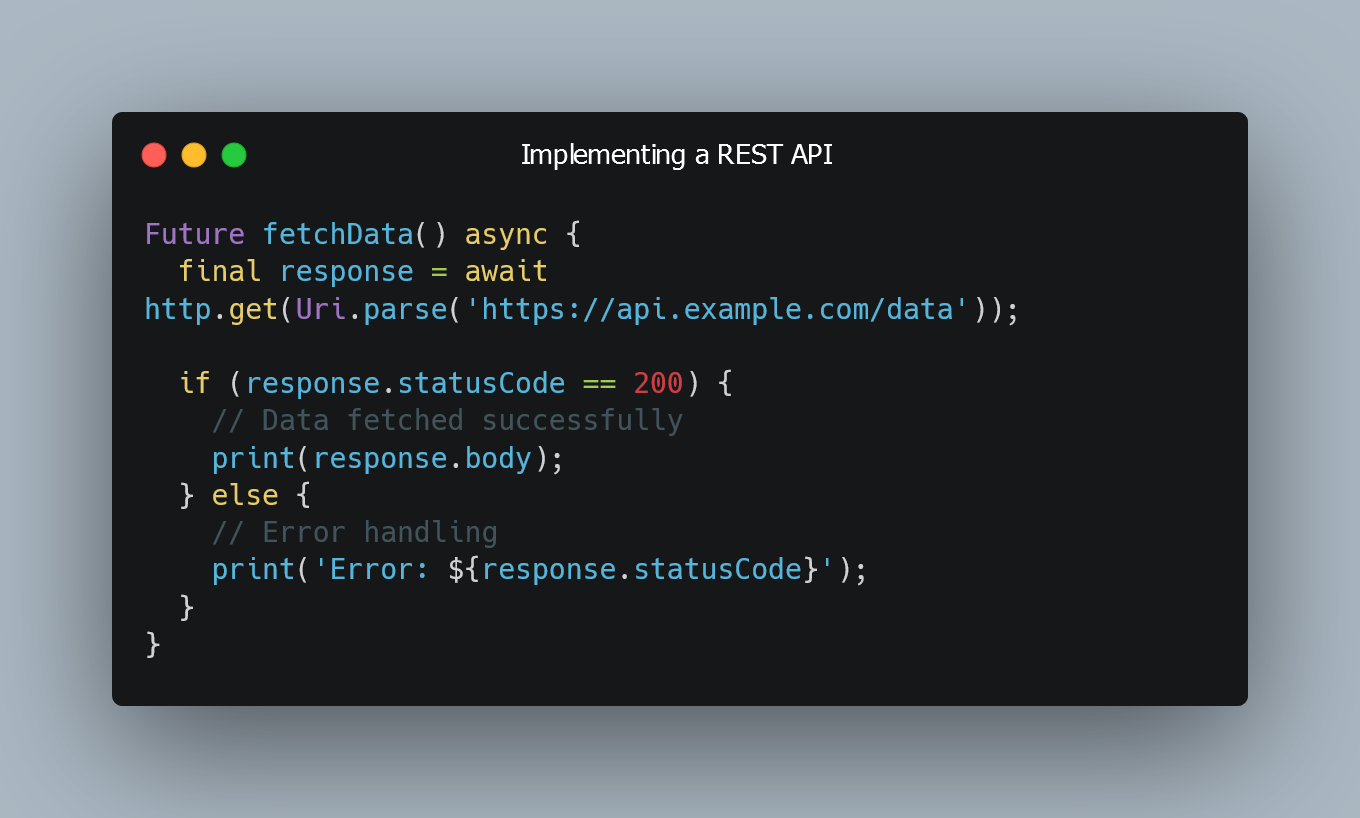
A REST API allows your Flutter app to communicate with a server and perform various operations like fetching data, posting data, or updating data.
Set Up the Server
Before implementing the REST API in your Flutter app, you'll need a server to interact with. You can create a server using any backend technology like Node.js, Python, or Ruby. The server should expose RESTful endpoints to handle HTTP requests.
Add the HTTP Package
In your pubspec.yaml
file, add the http
package to your dependencies:
dependencies:
flutter:
sdk: flutter
http: ^0.14.0
Import the package in your Dart file:
import 'package:http/http.dart' as http;
Make HTTP Requests
Use the http
package to make HTTP requests to your server's RESTful endpoints. For example, to perform a GET request:
Future fetchData() async {
final response = await http.get(Uri.parse('https://api.example.com/data'));
if (response.statusCode == 200) {
// Data fetched successfully
print(response.body);
} else {
// Error handling
print('Error: ${response.statusCode}');
}
}
Send Data to the Server
To send data to the server, use a POST request. For example:
Future sendData() async {
final response = await http.post(Uri.parse('https://api.example.com/data'),
body: {'key': 'value'});
if (response.statusCode == 201) {
// Data sent successfully
print('Data sent successfully');
} else {
// Error handling
print('Error: ${response.statusCode}');
}
}
Handle the Response
In your Flutter app, handle the response received from the server according to your app's requirements. You can parse the JSON data received from the server using the json
package, if necessary.
Error Handling
Always implement error handling to handle network-related issues, server errors, or any other unexpected situations. Use try-catch blocks or implement error handlers based on the response status codes.
Additional Operations
You can implement other RESTful operations like updating data (PUT request) or deleting data (DELETE request) based on your app's requirements. Use the appropriate HTTP methods and handle the responses accordingly.
0 Comment