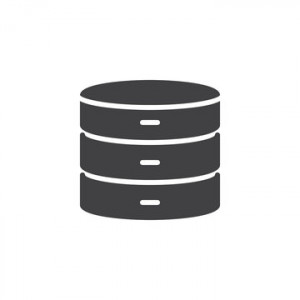
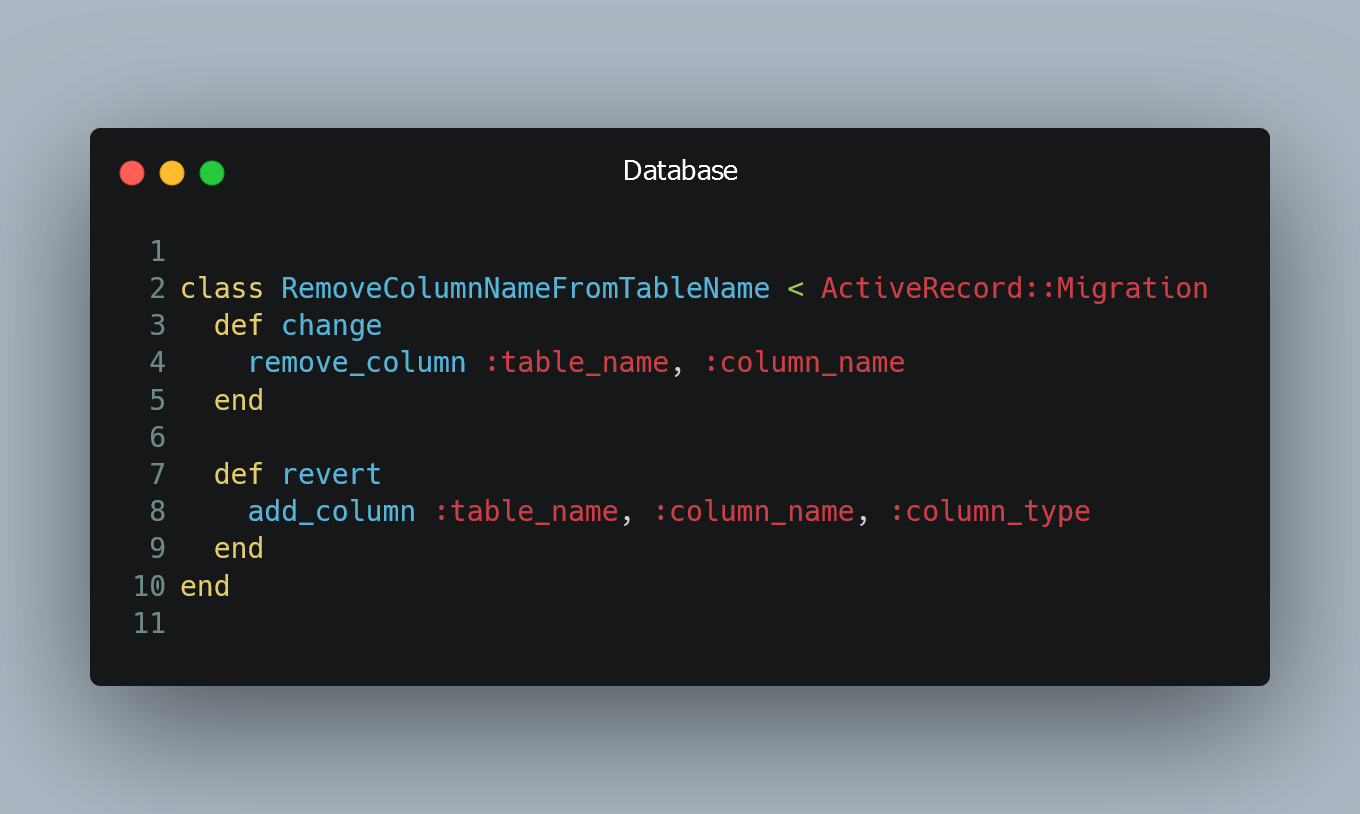
To drop columns from a database table using Rails migration, follow these steps:
Generate a Migration File
The first step is to generate a migration file that will contain the necessary instructions to drop the column. In your terminal, run the following command:
rails generate migration RemoveColumnNameFromTableName
Replace ColumnName
with the name of the column you want to drop, and TableName
with the name of the table that contains the column.
Edit the Generated Migration File
After running the generator command, you'll find the migration file in the db/migrate
directory. Open the file with your preferred text editor.
Inside the migration file, you'll see a class that inherits from ActiveRecord::Migration
. The change
method is where you'll define the changes to the database.
Define the Column Removal
In the change
method, use the remove_column
method to specify the table name and the column you want to drop. Here's the syntax:
class RemoveColumnNameFromTableName < ActiveRecord::Migration
def change
remove_column :table_name, :column_name
end
end
Replace table_name
with the name of the table containing the column, and column_name
with the name of the column you want to drop.
Running the Migration
Save the changes to the migration file, and then run the migration with the following command:
rails db:migrate
Rails will execute the migration, and the specified column will be removed from the database table.
Rolling Back (Optional)
If you need to undo the migration and restore the dropped column, you can define a revert
method in the same migration file:
class RemoveColumnNameFromTableName < ActiveRecord::Migration
def change
remove_column :table_name, :column_name
end
def revert
add_column :table_name, :column_name, :column_type
end
end
Replace column_type
with the appropriate data type for the column you are restoring.
To roll back the migration, use the following command:
rails db:rollback
Please note that rolling back migrations can lead to data loss, so be cautious when using this command in a production environment.
Congratulations! You've learned how to drop columns from a database table using Rails migration.
0 Comment