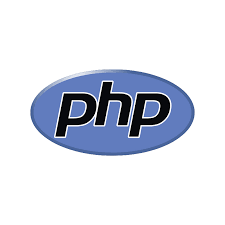
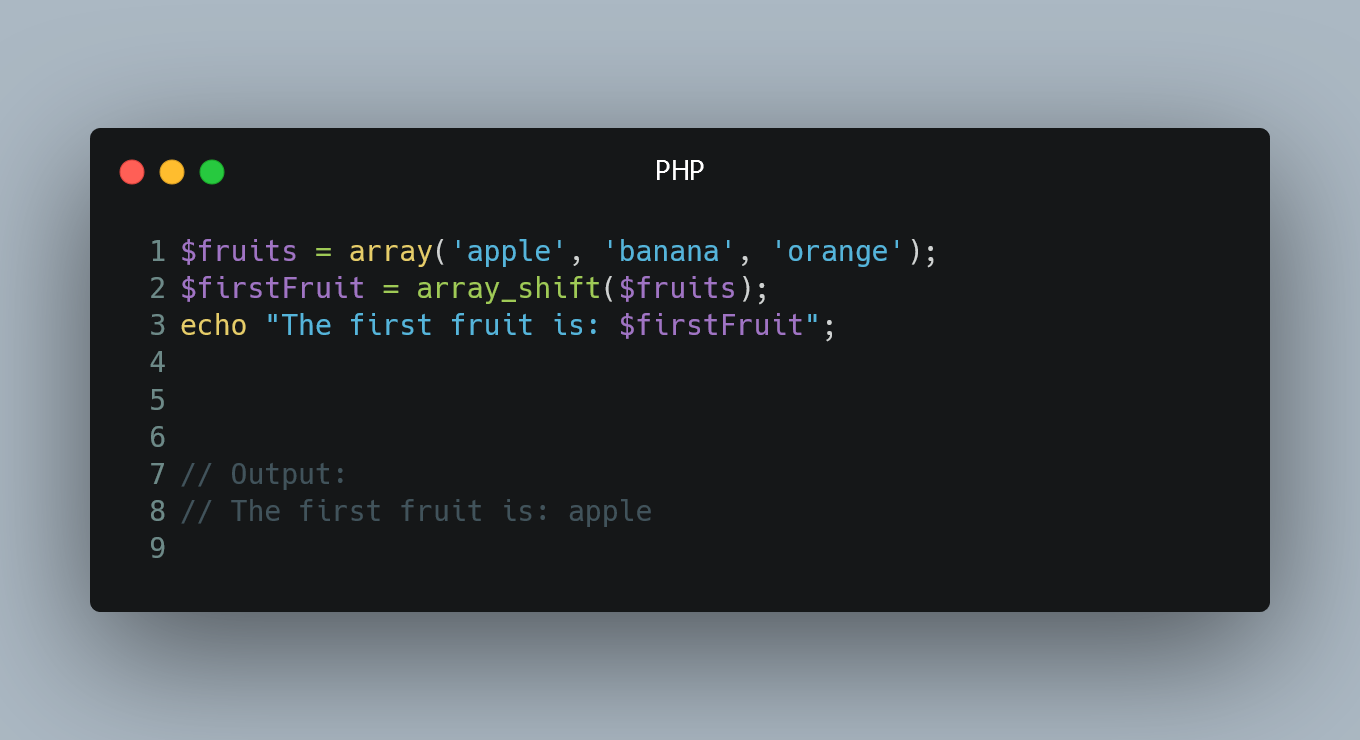
In PHP, you can retrieve the first element of an array using several methods.
Using array_shift() Function
The array_shift() Function
The array_shift() function is a built-in PHP function used to remove and return the first element of an array.
Syntax of array_shift()
$firstElement = array_shift($array);
Example
$fruits = array('apple', 'banana', 'orange');
$firstFruit = array_shift($fruits);
echo "The first fruit is: $firstFruit";
Output:
The first fruit is: apple
In this example, array_shift() is used to extract and return the first element of the '$fruits' array.
Using reset() Function
The reset() Function
The reset() function is a built-in PHP function that moves the internal pointer of an array to its first element and returns that element.
Syntax of reset()
$firstElement = reset($array);
Example
$colors = array('red', 'green', 'blue');
$firstColor = reset($colors);
echo "The first color is: $firstColor";
Output:
The first color is: red
In this example, reset() is used to move the pointer to the first element of the '$colors' array and retrieve the value of that element.
Using array_slice() Function
The array_slice() Function
The array_slice() function allows you to extract a portion of an array. By specifying the start index as 0 and the length as 1, you can get the first element of the array.
Syntax of array_slice()
$firstElement = array_slice($array, 0, 1)[0];
Example
$numbers = array(10, 20, 30, 40, 50);
$firstNumber = array_slice($numbers, 0, 1)[0];
echo "The first number is: $firstNumber";
Output:
The first number is: 10
In this example, array_slice() is used to get a one-element slice from the '$numbers' array, and the first element of that slice is the first element of the original array.
0 Comment