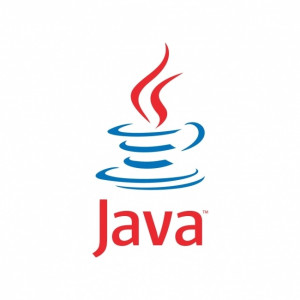
In Java, you can easily generate random integers within a specific range using the java.util.Random
class.
Creating a Random Object
To generate random integers, you need to create an instance of the Random
class.
Example:
import java.util.Random;
public class Main {
public static void main(String[] args) {
Random random = new Random();
// Your code to generate random integers within a specific range goes here
}
}
In this example, we create a Random
object named random
, which we will use to generate random integers.
Generating Random Integers within a Range
To generate random integers within a specific range, you can use the nextInt(int bound)
method of the Random
class. The nextInt(int bound)
method returns a random integer between 0 (inclusive) and the specified bound (exclusive).
Example:
import java.util.Random;
public class Main {
public static void main(String[] args) {
Random random = new Random();
// Generate a random integer between 1 and 100 (inclusive)
int randomNumber = random.nextInt(100) + 1;
System.out.println("Random Number: " + randomNumber);
}
}
In this example, random.nextInt(100)
generates a random integer between 0 and 99. To obtain a random number between 1 and 100 (inclusive), we add 1 to the result.
Generating Random Integers within a Custom Range
To generate random integers within a custom range, you can use the formula random.nextInt((max - min) + 1) + min
.
Example:
import java.util.Random;
public class Main {
public static void main(String[] args) {
Random random = new Random();
int min = 10;
int max = 20;
// Generate a random integer between min and max (inclusive)
int randomNumber = random.nextInt((max - min) + 1) + min;
System.out.println("Random Number: " + randomNumber);
}
}
In this example, random.nextInt((max - min) + 1) + min
generates a random integer between min
and max
(inclusive).
0 Comment