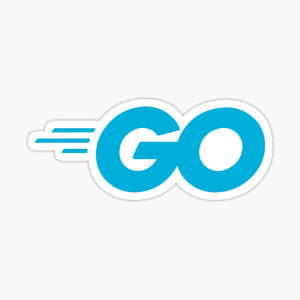
Go does not have a traditional foreach loop like some other programming languages. Instead, it uses a range-based iteration method to loop over elements in collections such as arrays, slices, maps, and strings.
Iterating Over Arrays and Slices
To iterate over elements in an array or slice, you can use the range
keyword followed by the array or slice variable. The range
keyword returns the index and the value of each element in the collection.
Example
package main
import "fmt"
func main() {
numbers := []int{1, 2, 3, 4, 5}
for index, value := range numbers {
fmt.Printf("Index: %d, Value: %d\n", index, value)
}
}
Iterating Over Maps
For maps, the range
keyword iterates over key-value pairs in the map. The range
keyword returns the key and the value of each key-value pair.
Example
package main
import "fmt"
func main() {
fruits := map[string]int{
"apple": 1,
"banana": 2,
"orange": 3,
}
for key, value := range fruits {
fmt.Printf("Key: %s, Value: %d\n", key, value)
}
}
Ignoring the Index or Key
If you are not interested in the index or key, you can use the blank identifier (_
) to ignore them during iteration.
Example
package main
import "fmt"
func main() {
colors := []string{"red", "green", "blue"}
for _, color := range colors {
fmt.Println("Color:", color)
}
}
0 Comment