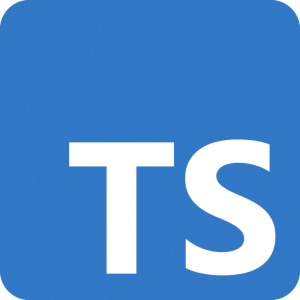
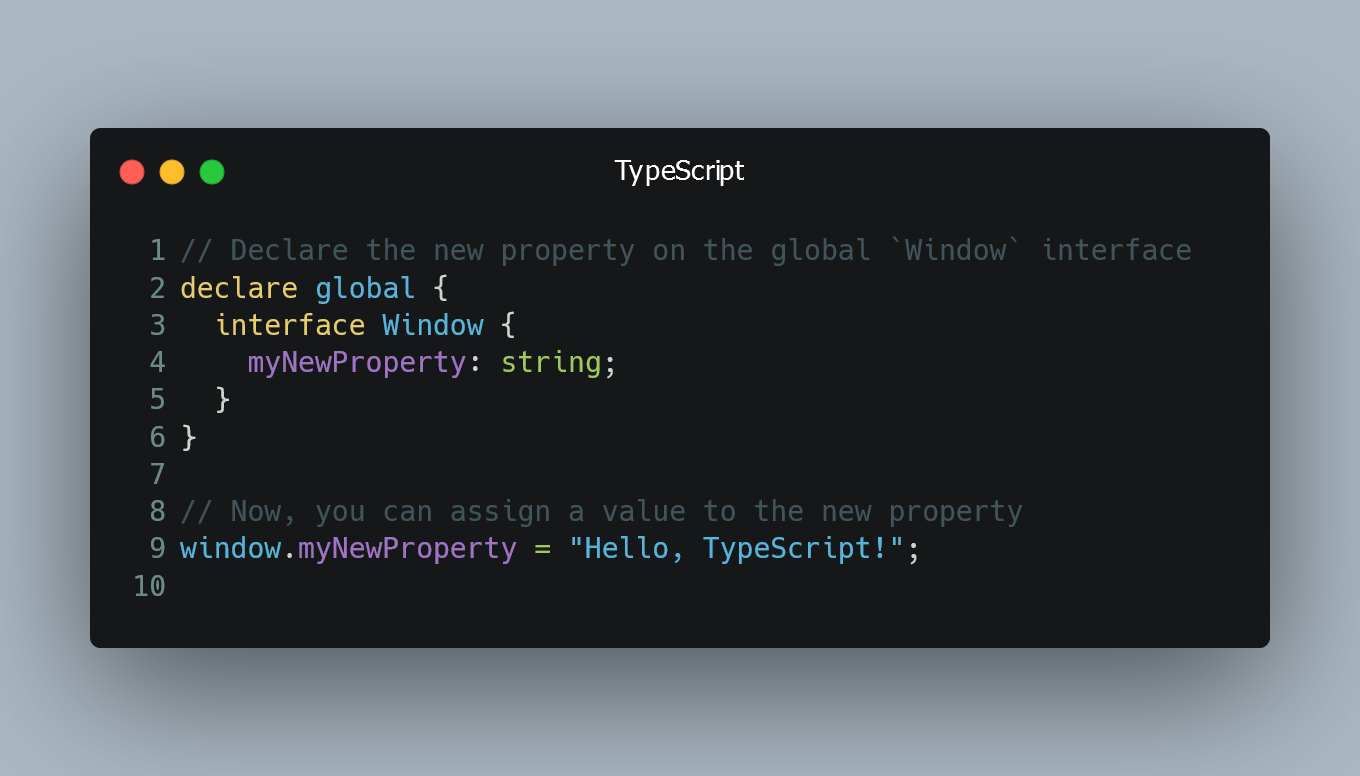
In TypeScript, when you want to add a new property to the window
object (the global object in the browser), you need to ensure that TypeScript recognizes the new property and its type.
#1. Using Declaration Merging
To explicitly set a new property on window
, you can use declaration merging in TypeScript. Declaration merging allows you to extend existing interfaces or types to include additional properties.
// Declare the new property on the global `Window` interface
declare global {
interface Window {
myNewProperty: string;
}
}
// Now, you can assign a value to the new property
window.myNewProperty = "Hello, TypeScript!";
In this example, we use the declare global
block to augment the Window
interface and add a new property called myNewProperty
, which is of type string
. After declaring the new property, you can freely assign values to it on the window
object.
#2. Using Type Assertion
Alternatively, you can use a type assertion to inform TypeScript about the new property.
// Use type assertion to add the new property
(window as any).myNewProperty = "Hello, TypeScript!";
In this approach, we use the (window as any)
type assertion to cast the window
object to the any
type, effectively disabling TypeScript's type checking for the object. This allows us to add the new property without any type errors.
#3. Avoid any
Type
While using a type assertion with any
is a quick way to add a new property to window
, it is not recommended in production code. The use of any
bypasses type checking, which can lead to potential runtime errors or hinder the benefits of using TypeScript.
0 Comment