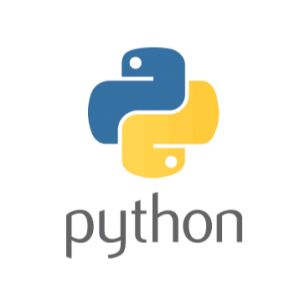
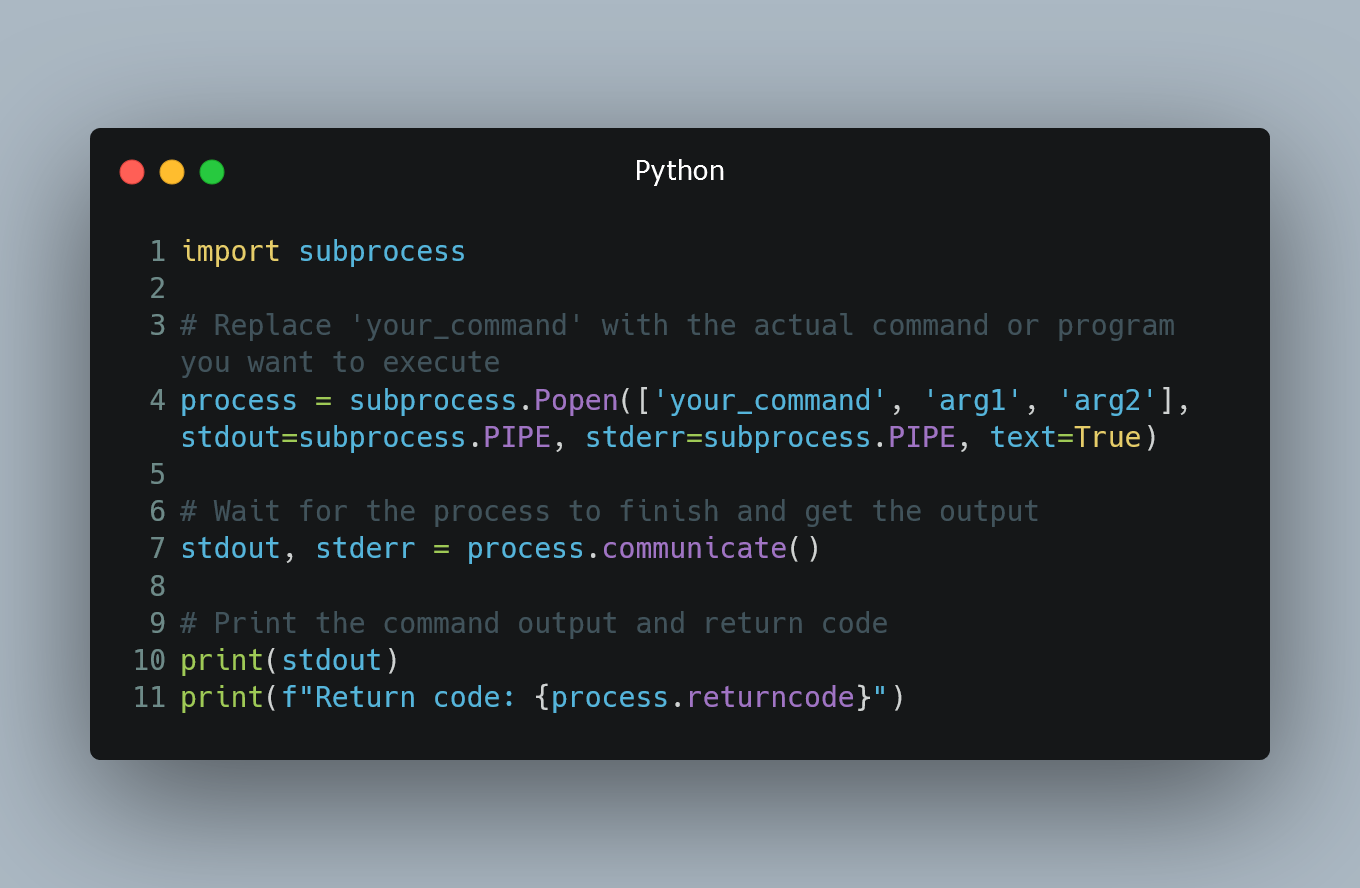
In Python, you can execute an external program or call a system command using various methods provided by the subprocess
module.
#1. Using subprocess.run()
The subprocess.run()
function is the simplest and recommended way to execute a program or system command. It is available in Python 3.5 and later versions.
import subprocess
# Replace 'your_command' with the actual command or program you want to execute
result = subprocess.run(['your_command', 'arg1', 'arg2'], capture_output=True, text=True)
# Print the command output and return code
print(result.stdout)
print(f"Return code: {result.returncode}")
The subprocess.run()
function runs the specified command with its arguments and returns a CompletedProcess
object that contains information about the execution, including the command's output and return code.
#2. Using subprocess.Popen()
For more advanced control and interaction with the process, you can use subprocess.Popen()
. It allows you to communicate with the process interactively and read its output and errors.
import subprocess
# Replace 'your_command' with the actual command or program you want to execute
process = subprocess.Popen(['your_command', 'arg1', 'arg2'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
# Wait for the process to finish and get the output
stdout, stderr = process.communicate()
# Print the command output and return code
print(stdout)
print(f"Return code: {process.returncode}")
#3. Using Shell Commands
You can also run shell commands using the shell=True
argument. However, using shell commands can be a security risk if the input is not properly validated, as it allows arbitrary code execution.
import subprocess
# Replace 'your_command' with the actual shell command you want to execute
result = subprocess.run('your_command', capture_output=True, text=True, shell=True)
# Print the command output and return code
print(result.stdout)
print(f"Return code: {result.returncode}")
#4. Handling Paths with Spaces (Windows)
If your command or program path contains spaces, you need to handle it properly, especially on Windows. Enclose the command or program path in double quotes.
import subprocess
# Example on Windows: Running 'C:\Program Files\your_program.exe'
result = subprocess.run(['"C:\\Program Files\\your_program.exe"'], capture_output=True, text=True)
#5. Handling User Input
Be cautious when using user input to construct the command, as it can lead to security vulnerabilities such as command injection. Always validate and sanitize user input before using it in subprocess calls.
0 Comment