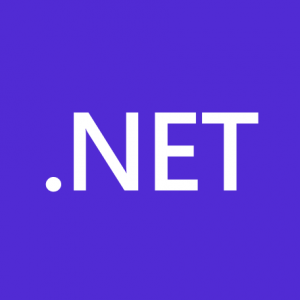
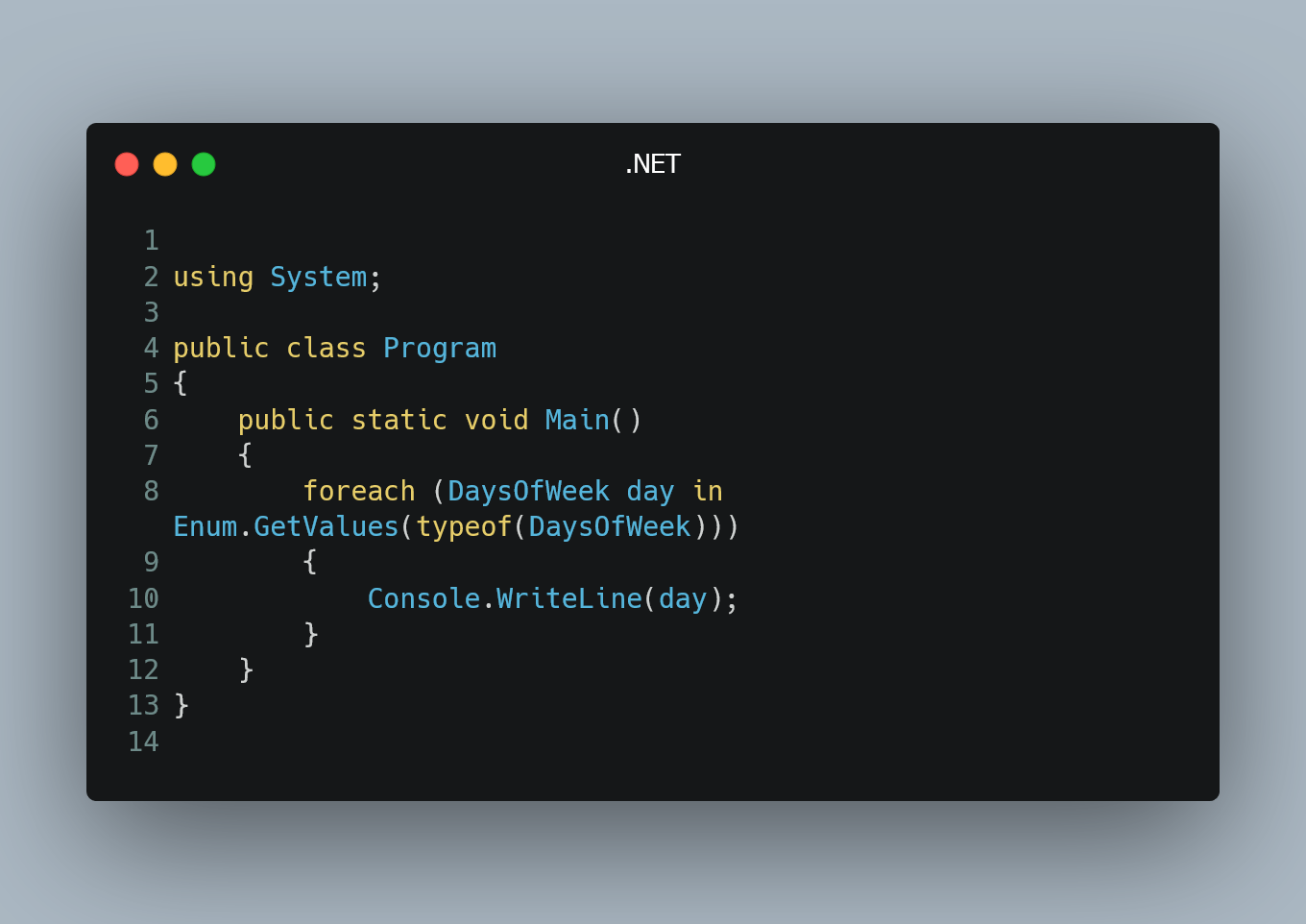
In C#, enumerations, or enums, are special value types that define a set of named constants. Enumerations make it easier to work with a predefined set of related values and improve code readability.
Enum Declaration
To begin, let's define an example enum. For this tutorial, we'll create an enum representing days of the week.
public enum DaysOfWeek
{
Sunday,
Monday,
Tuesday,
Wednesday,
Thursday,
Friday,
Saturday
}
Enumerating Enum Values
Enumerating an enum involves iterating over all its defined values. In C#, you can use the Enum.GetValues()
method to achieve this.
using System;
public class Program
{
public static void Main()
{
foreach (DaysOfWeek day in Enum.GetValues(typeof(DaysOfWeek)))
{
Console.WriteLine(day);
}
}
}
In the code above, we use a foreach
loop to iterate over each value of the DaysOfWeek
enum. The Enum.GetValues(typeof(DaysOfWeek))
call retrieves an array containing all the defined values of the enum, and the loop prints each value to the console.
Output
When you run the program, it will display the days of the week, one by one, in the console:
Sunday
Monday
Tuesday
Wednesday
Thursday
Friday
Saturday
Using Enumerated Values
Enumerating an enum is beneficial when you need to perform operations on all its possible values. For example, you might use this technique when mapping enum values to database entries, generating user interface elements, or implementing specific business logic based on the enum's values.
0 Comment