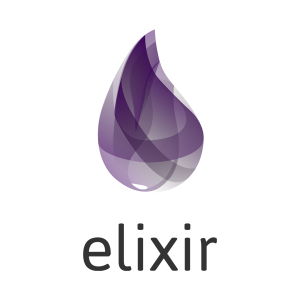
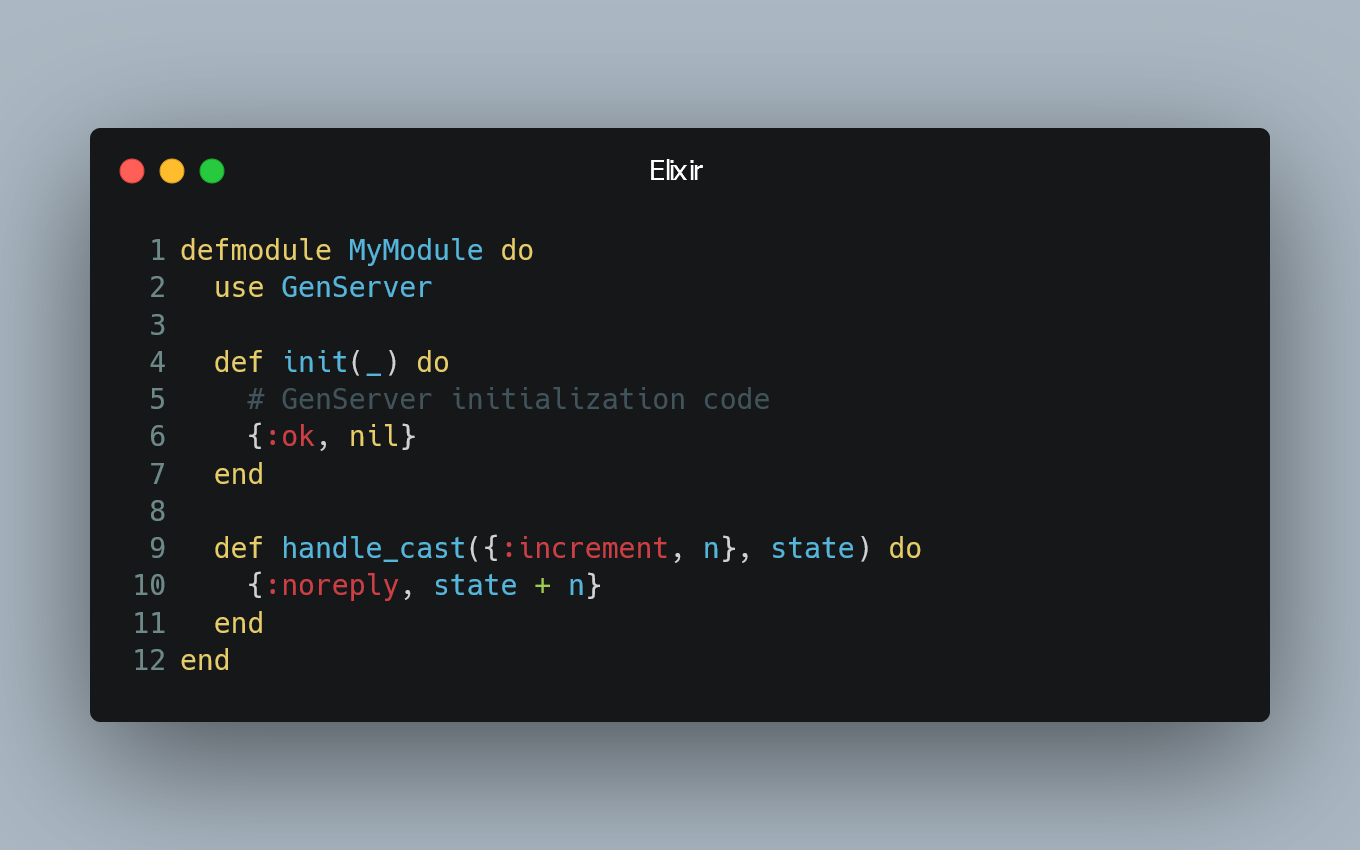
In Elixir, both use
and import
are used to include external modules or functionality into the current module. However, they serve different purposes and have distinct use cases.
Use Macro with Use
The use
macro is used to invoke module macros and apply transformations to the current module. It is commonly used for adding functionalities, defining behaviours, and setting up configurations.
Example:
defmodule MyModule do
use GenServer
def init(_) do
# GenServer initialization code
{:ok, nil}
end
def handle_cast({:increment, n}, state) do
{:noreply, state + n}
end
end
In this example, we use use GenServer
to include the GenServer
module's macros and behavior into the MyModule
module. This allows us to define the init/1
and handle_cast/2
functions as part of the GenServer
behavior.
Importing Functions with Import
The import
statement is used to bring functions from an external module into the current module's scope. It allows you to call the imported functions directly without having to prefix them with the module name.
Example:
defmodule MyModule do
import List, only: [duplicate: 2]
def get_duplicates(list) do
duplicate(list, 3)
end
end
In this example, we use import List, only: [duplicate: 2]
to import the duplicate/2
function from the List
module. Now, we can call duplicate/2
directly in the get_duplicates/1
function without the need to specify List.duplicate/2
.
Use vs Import
-
use
is typically used for setting up the behavior of the current module, applying transformations, or adding functionalities. It is often used with behaviors likeGenServer
,Agent
, or when defining custom behaviors. -
import
, on the other hand, is used for bringing specific functions into the current module's scope, making them available without the need to prefix the module name. It is useful when you want to use a few functions from a module frequently. -
It is essential to use
use
andimport
judiciously and only when necessary to avoid potential naming conflicts and to keep the code clean and maintainable.
0 Comment