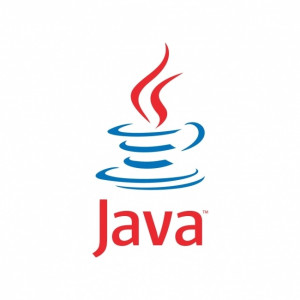
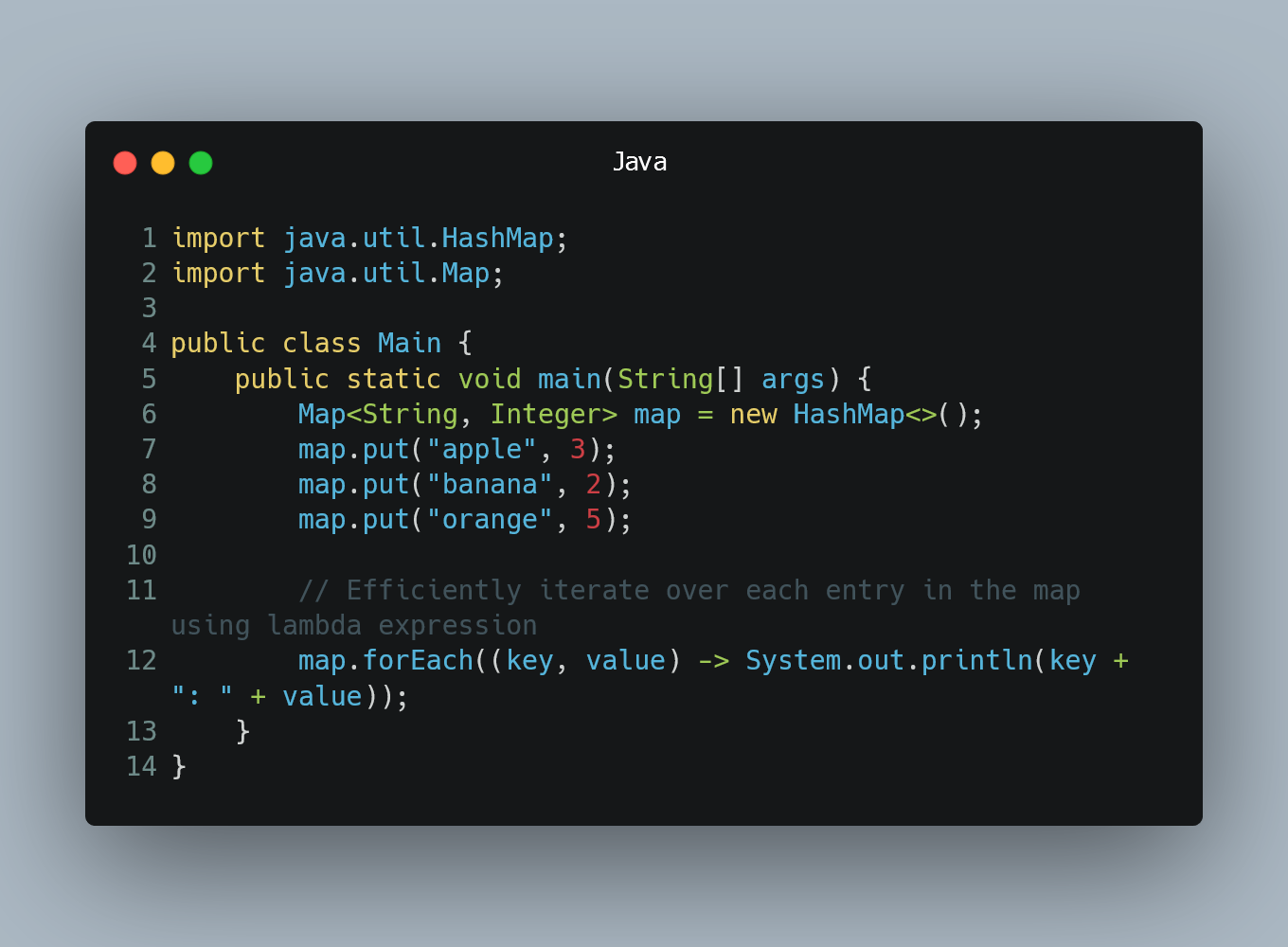
In Java, iterating over the entries of a map is a common task. While there are multiple ways to achieve this, some approaches are more efficient than others.
Using EntrySet
The most efficient and recommended way to iterate over a map is by using the entrySet()
method of the map. It returns a set of map entries, where each entry contains both the key and the corresponding value.
Example:
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("apple", 3);
map.put("banana", 2);
map.put("orange", 5);
// Efficiently iterate over each entry in the map
for (Map.Entry<String, Integer> entry : map.entrySet()) {
String key = entry.getKey();
Integer value = entry.getValue();
System.out.println(key + ": " + value);
}
}
}
In this example, we use the entrySet()
method to get a set of map entries. Then, we iterate over each entry in the map and extract the key and value using the getKey()
and getValue()
methods of the Map.Entry
interface.
Efficiency Advantages
Using entrySet()
for iteration is more efficient because it avoids redundant lookups. When iterating through the map, each call to getKey()
or getValue()
directly accesses the key or value associated with the current entry without the need for an additional lookup.
Enhanced For-Loop (Java 8+)
If you are using Java 8 or later, you can further simplify the iteration using the enhanced for-loop and lambda expressions.
Example:
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("apple", 3);
map.put("banana", 2);
map.put("orange", 5);
// Efficiently iterate over each entry in the map using lambda expression
map.forEach((key, value) -> System.out.println(key + ": " + value));
}
}
In this Java 8 example, we use the forEach()
method of the map to iterate over each entry and directly print the key-value pair using a lambda expression.
0 Comment