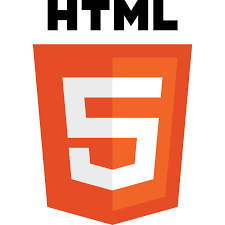
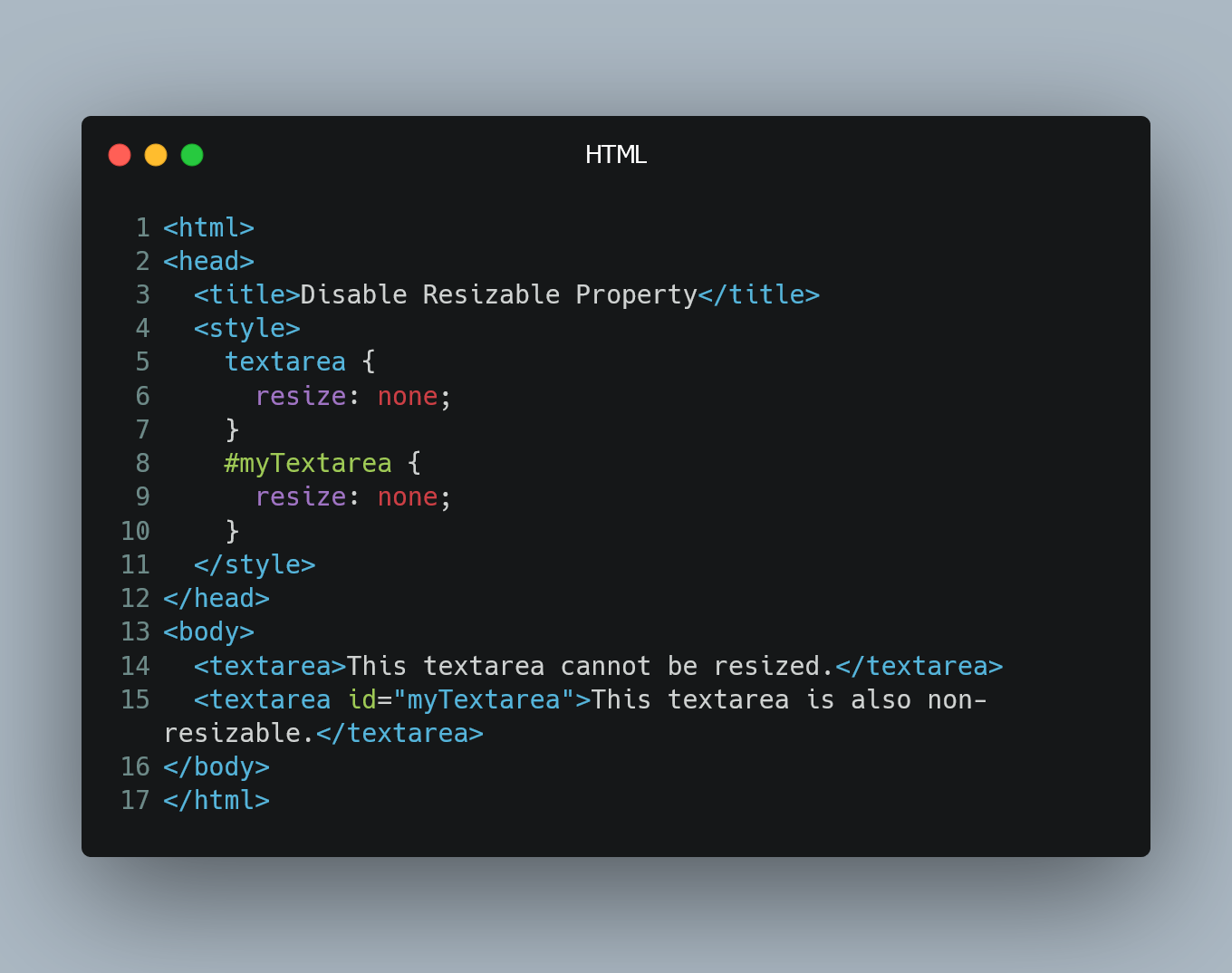
To prevent users from resizing a textarea element, you can use CSS to disable the resizable property.
#1. Using CSS Resize Property
You can use the resize
property in CSS to control the resizing behavior of a textarea.
<!DOCTYPE html>
<html>
<head>
<title>Disable Resizable Property</title>
<style>
/* Disable resizing for all textareas */
textarea {
resize: none;
}
/* Or target a specific textarea by its ID or class */
#myTextarea {
resize: none;
}
</style>
</head>
<body>
<textarea>This textarea cannot be resized.</textarea>
<textarea id="myTextarea">This textarea is also non-resizable.</textarea>
</body>
</html>
In this example, we use the CSS resize: none;
property to disable the resizing feature for all <textarea>
elements on the page. If you only want to target a specific textarea by its ID or class, you can add a more specific selector, as shown with #myTextarea
in the example.
#2. Using JavaScript
If you need to disable the resizing property dynamically or programmatically, you can achieve it using JavaScript.
<!DOCTYPE html>
<html>
<head>
<title>Disable Resizable Property</title>
<style>
/* Optional: Set a fixed height to ensure no resizing visually occurs */
textarea {
height: 100px;
}
</style>
</head>
<body>
<textarea id="myTextarea">This textarea can be resized.</textarea>
<button onclick="disableResizing()">Disable Resizing</button>
<script>
// Function to disable resizing
function disableResizing() {
const textarea = document.getElementById("myTextarea");
textarea.style.resize = "none";
}
</script>
</body>
</html>
In this example, we have a textarea with the ID "myTextarea"
and a button that triggers the JavaScript function disableResizing()
when clicked. Inside the function, we use the style.resize
property to set the textarea's resizing behavior to "none"
, effectively disabling the resizable property.
0 Comment