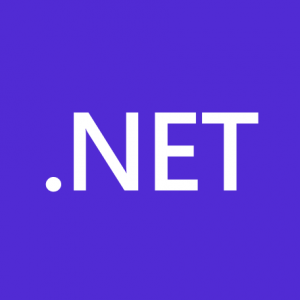
In .NET, decimal, float, and double are three different data types used to represent floating-point numbers. Each of these types has specific characteristics, making them suitable for different scenarios.
Decimal
The decimal data type in .NET is a 128-bit data type designed for precise decimal arithmetic. It is suitable for financial calculations, where precision is critical to avoid rounding errors. The decimal type has a higher precision but a smaller range compared to float and double.
Example:
decimal myDecimal = 123.45m;
In the example above, the m
suffix is used to indicate a decimal literal.
Float
The float data type in .NET is a 32-bit single-precision floating-point type. It is suitable for scenarios that require a wider range of values but can tolerate some loss of precision. Floats are generally used when memory is a concern, and the calculations do not require a high level of precision.
Example:
float myFloat = 3.14f;
In the example above, the f
suffix is used to indicate a float literal.
Double
The double data type in .NET is a 64-bit double-precision floating-point type. It provides a larger range and higher precision compared to float, making it a good choice for most general-purpose floating-point calculations.
Example:
double myDouble = 2.71828;
In the example above, the double variable myDouble
is assigned the value of Euler's number.
Precision and Range Comparison
Data Type | Precision | Range |
---|---|---|
decimal | 28-29 significant digits | ±1.0 x 10^-28 to ±7.9 x 10^28 |
float | 7 significant digits | ±1.5 x 10^-45 to ±3.4 x 10^38 |
double | 15-16 significant digits | ±5.0 x 10^-324 to ±1.7 x 10^308 |
Choosing the Right Type
When deciding which data type to use, consider the specific requirements of your application. If precision is crucial, especially for financial calculations, the decimal type is the most suitable choice. For general-purpose calculations with a wide range of values, double is often sufficient. Use float when memory constraints are significant and the loss of precision is acceptable.
0 Comment