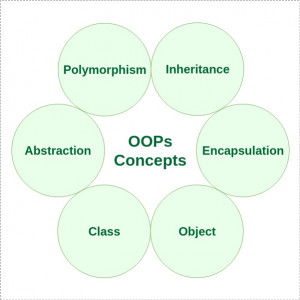
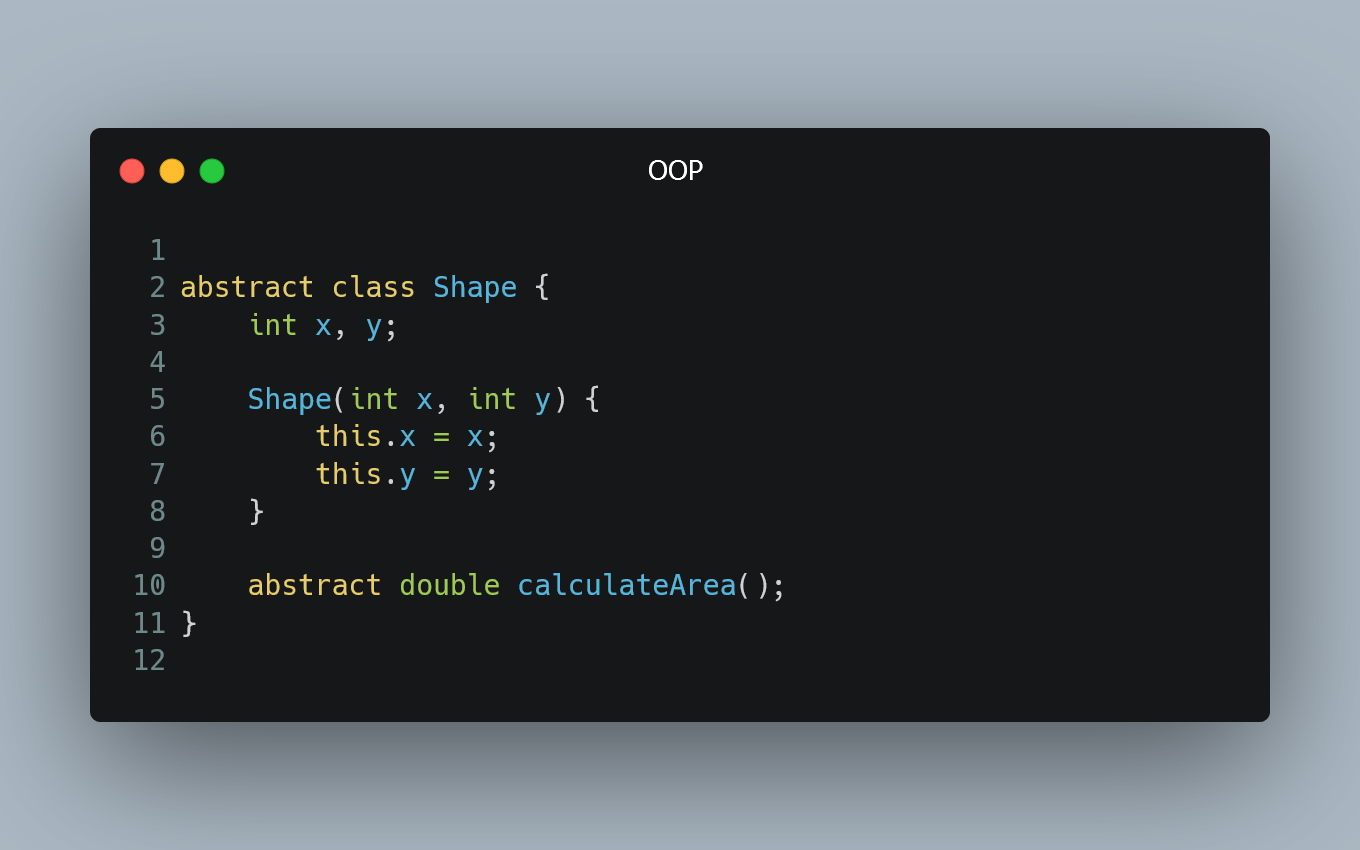
In object-oriented programming (OOP), both interfaces and abstract classes are used as blueprints for creating classes with common characteristics.
Abstract Class
An abstract class is a class that cannot be instantiated on its own and serves as a blueprint for other classes. It may contain both concrete methods (with implementations) and abstract methods (without implementations). Abstract methods act as placeholders that must be implemented by the classes that inherit from the abstract class.
Example of an Abstract Class (Java):
abstract class Shape {
int x, y;
Shape(int x, int y) {
this.x = x;
this.y = y;
}
abstract double calculateArea();
}
In this example, Shape
is an abstract class with an abstract method calculateArea()
. It cannot be instantiated, but other classes can inherit from it and provide their own implementation for calculateArea()
.
Interface
An interface is a collection of abstract methods that define a contract. It contains method signatures without any implementations. In OOP, a class can implement multiple interfaces, allowing it to adhere to the contracts defined by those interfaces.
Example of an Interface (C#):
interface ILoggable {
void LogInfo(string message);
void LogError(string error);
}
In this example, ILoggable
is an interface with two method signatures, LogInfo()
and LogError()
. Any class that implements this interface must provide concrete implementations for both methods.
Key Differences
-
Instantiation: Abstract classes cannot be instantiated directly, while interfaces cannot be instantiated at all. Classes can inherit from an abstract class, but they implement interfaces.
-
Method Implementation: Abstract classes can have concrete methods (with implementations) in addition to abstract methods, whereas interfaces only contain method signatures without implementations.
-
Multiple Inheritance: A class can inherit from only one abstract class, but it can implement multiple interfaces. This allows for a form of multiple inheritance using interfaces.
-
Usage: Abstract classes are used when you want to provide a common base for a group of related classes, with the flexibility to add both common and varying functionality. Interfaces are used when you want to define a contract that multiple unrelated classes can adhere to, promoting code reusability and polymorphism.
0 Comment