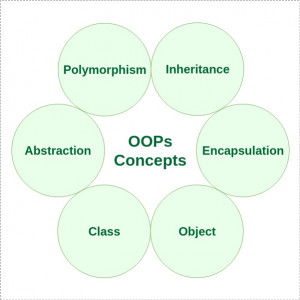
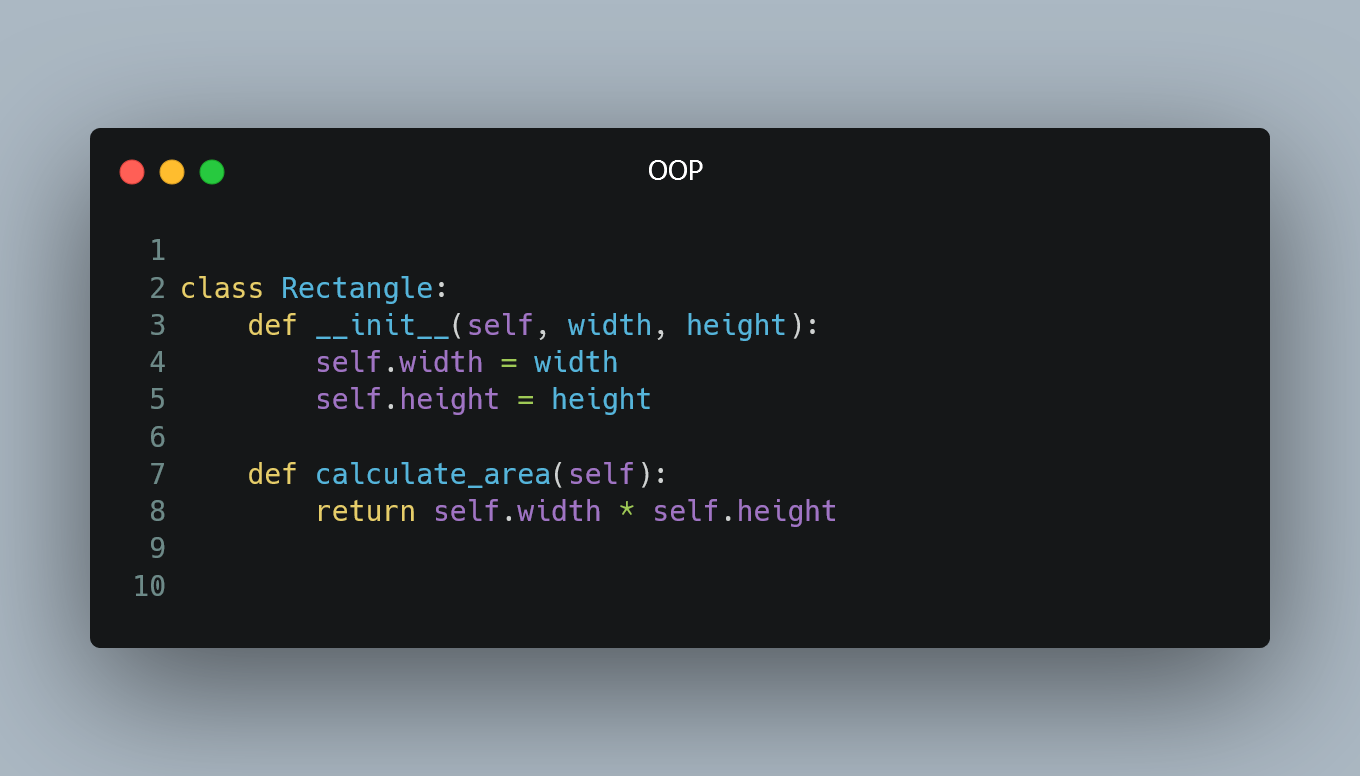
In programming, the terms "method" and "function" are often used to describe blocks of code that perform a specific task. While they share similarities, there are fundamental differences between them.
Functions
A function is a self-contained block of code that performs a particular task or calculation. It takes input, processes it, and produces an output. Functions are typically independent and can be used in various parts of a program.
Example of a Function (Python):
def add_numbers(a, b):
return a + b
In this example, add_numbers
is a function that takes two arguments a
and b
and returns their sum.
Methods
A method is similar to a function, but it is associated with an object or a class in object-oriented programming. Methods are defined within a class and operate on the data (attributes) of that class.
Example of a Method (Python):
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def calculate_area(self):
return self.width * self.height
In this example, calculate_area
is a method defined within the Rectangle
class. It operates on the attributes width
and height
of the class to calculate the area of the rectangle.
Key Differences
-
Association: Functions are standalone and can be used anywhere in the program. Methods, on the other hand, are associated with objects or classes and operate on their attributes.
-
Invocation: Functions are invoked by their names, and their arguments are passed explicitly. Methods are invoked on objects or instances of a class, and the object itself is implicitly passed as the first argument (often referred to as
self
in Python). -
Scope: Functions have their own scope, and they do not have access to attributes or data of specific objects. Methods, being associated with a class, have access to the data (attributes) of that class through the
self
parameter.
0 Comment