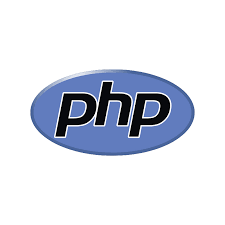
In PHP, you can remove an element from an array using built-in array manipulation functions.
Using unset() Function
What is unset()?
The unset()
function is a PHP construct used to remove a specific element from an array. It modifies the original array directly.
Syntax of unset()
unset($array[$index]);
Here, $array
is the array from which you want to delete an element, and $index
is the index of the element you want to remove.
Example
$fruits = array('apple', 'banana', 'orange', 'grape');
unset($fruits[1]); // Remove 'banana' from the array
print_r($fruits);
In this example, the unset()
function is used to delete the element at index 1 from the $fruits
array. The output will be Array ( [0] => apple [2] => orange [3] => grape )
.
Using array_splice() Function
What is array_splice()?
The array_splice()
function is used to remove a portion of the array and replace it with new elements if needed. It can also be used to delete a single element from an array.
Syntax of array_splice()
array_splice($array, $index, 1);
Here, $array
is the array from which you want to delete an element, $index
is the index of the element you want to remove, and 1
represents the number of elements to remove.
Example
$fruits = array('apple', 'banana', 'orange', 'grape');
array_splice($fruits, 1, 1); // Remove 'banana' from the array
print_r($fruits);
In this example, the array_splice()
function is used to delete the element at index 1 from the $fruits
array. The output will be Array ( [0] => apple [2] => orange [3] => grape )
.
0 Comment