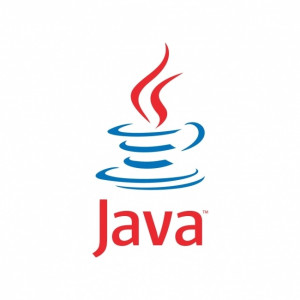
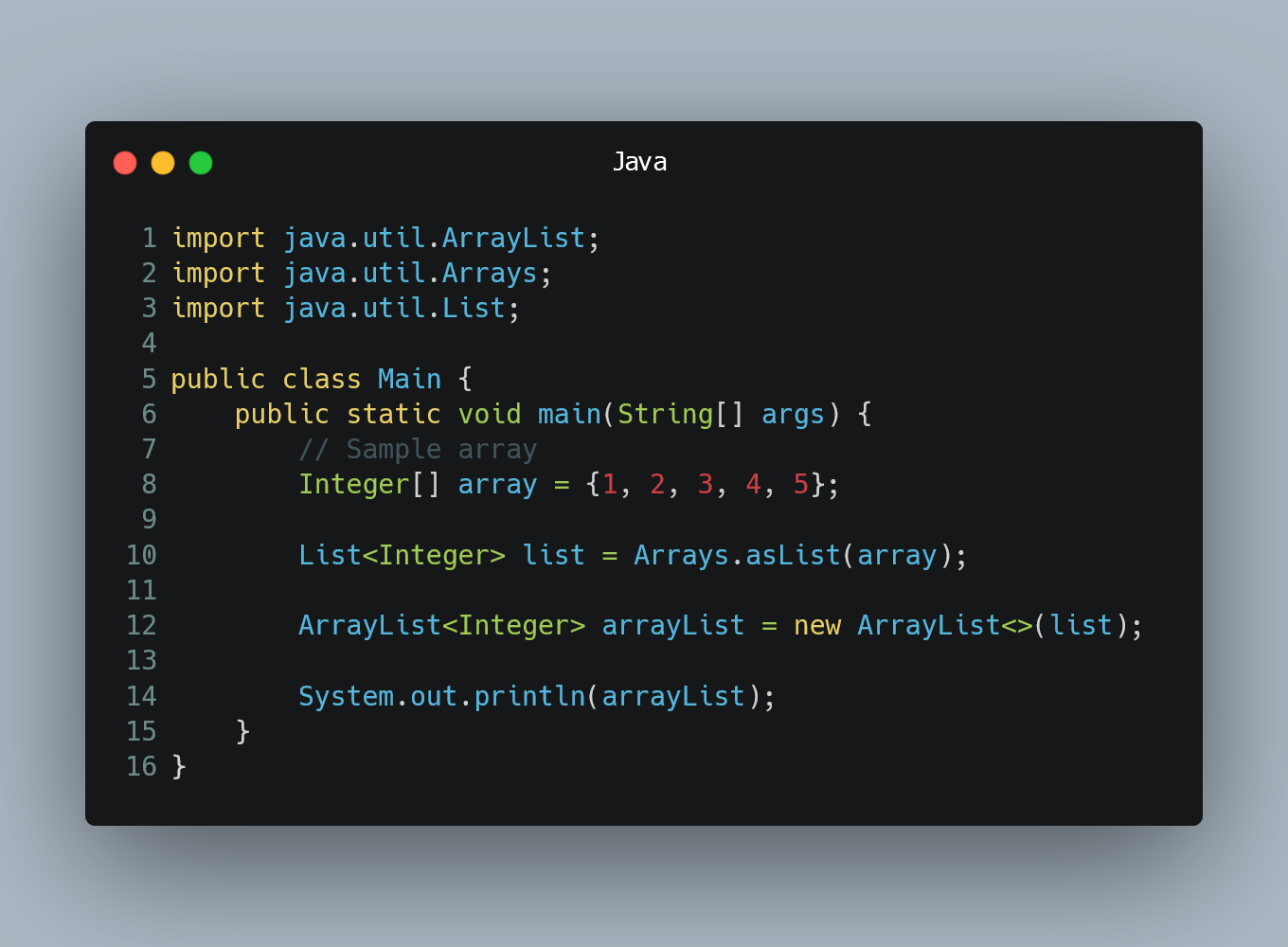
In Java, you can easily convert an array into an ArrayList to take advantage of the dynamic resizing and additional methods provided by ArrayList.
Using Arrays.asList()
The Arrays.asList()
method is a convenient way to convert an array into a List, which can then be easily transformed into an ArrayList.
Example:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Sample array
Integer[] array = {1, 2, 3, 4, 5};
// Convert array to List
List<Integer> list = Arrays.asList(array);
// Convert List to ArrayList
ArrayList<Integer> arrayList = new ArrayList<>(list);
// Print ArrayList
System.out.println(arrayList);
}
}
In this example, we start with an array of integers. First, we use Arrays.asList(array)
to convert the array into a List. Then, we pass this List to the ArrayList constructor, which creates a new ArrayList containing the elements of the List. Finally, we print the ArrayList to verify the conversion.
Modifying the ArrayList
After creating the ArrayList from the array, you can modify the ArrayList independently of the original array.
Example:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Sample array
String[] array = {"apple", "banana", "orange"};
// Convert array to List
List<String> list = Arrays.asList(array);
// Convert List to ArrayList
ArrayList<String> arrayList = new ArrayList<>(list);
// Add a new element to the ArrayList
arrayList.add("grape");
// Print the modified ArrayList
System.out.println(arrayList);
}
}
In this example, we start with an array of strings. After converting the array to an ArrayList, we add a new element, "grape," to the ArrayList. This demonstrates that the ArrayList is separate from the original array and can be modified independently.
0 Comment