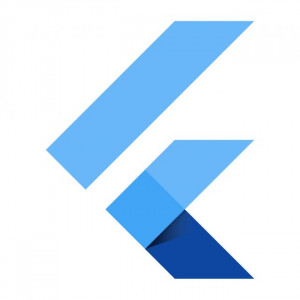
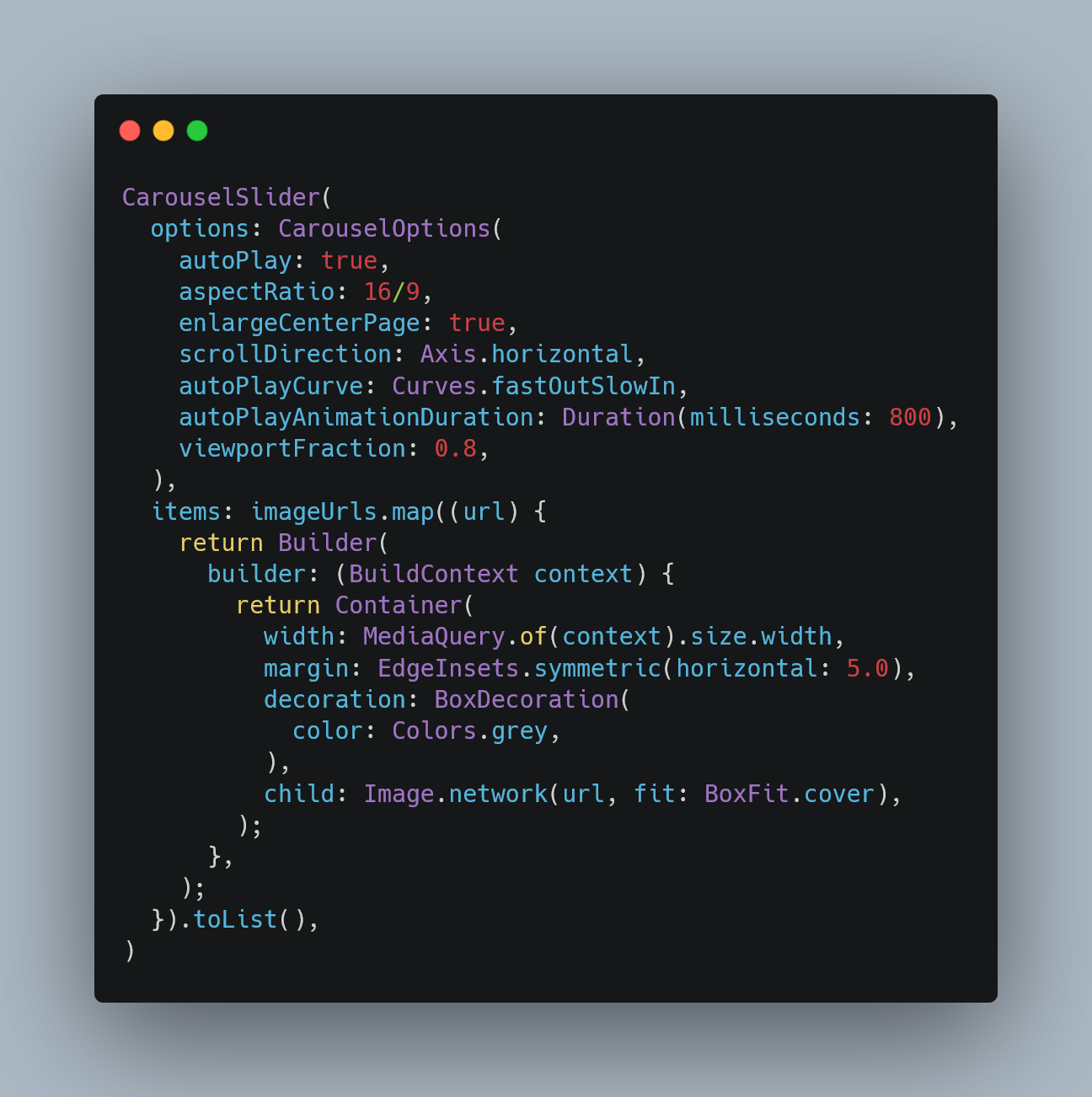
A carousel slider is a common UI element in mobile app development that allows users to swipe through a series of images or content in a carousel-like manner.
Setting Up the Project:
Before we begin, make sure you have Flutter installed and set up on your system. Create a new Flutter project using the following command:
flutter create carousel_slider_demo
Adding Dependencies:
In your pubspec.yaml
file, add the carousel_slider
package to your dependencies:
dependencies:
flutter:
sdk: flutter
carousel_slider: ^4.0.0
Import the package:
import 'package:carousel_slider/carousel_slider.dart';
Creating the Carousel Slider:
Step 1: Prepare the List of Images or Content:
Create a list of images or content that you want to display in the carousel slider. For this example, we'll use images.
List imageUrls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg',
];
Step 2: Build the Carousel Slider Widget:
In the build
method of your widget, create the carousel slider using the CarouselSlider
widget.
CarouselSlider(
options: CarouselOptions(
autoPlay: true,
aspectRatio: 16/9,
enlargeCenterPage: true,
scrollDirection: Axis.horizontal,
autoPlayCurve: Curves.fastOutSlowIn,
autoPlayAnimationDuration: Duration(milliseconds: 800),
viewportFraction: 0.8,
),
items: imageUrls.map((url) {
return Builder(
builder: (BuildContext context) {
return Container(
width: MediaQuery.of(context).size.width,
margin: EdgeInsets.symmetric(horizontal: 5.0),
decoration: BoxDecoration(
color: Colors.grey,
),
child: Image.network(url, fit: BoxFit.cover),
);
},
);
}).toList(),
)
0 Comment