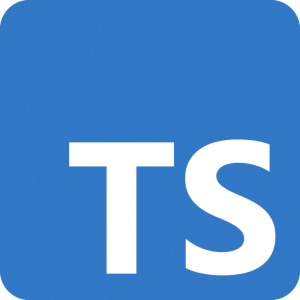
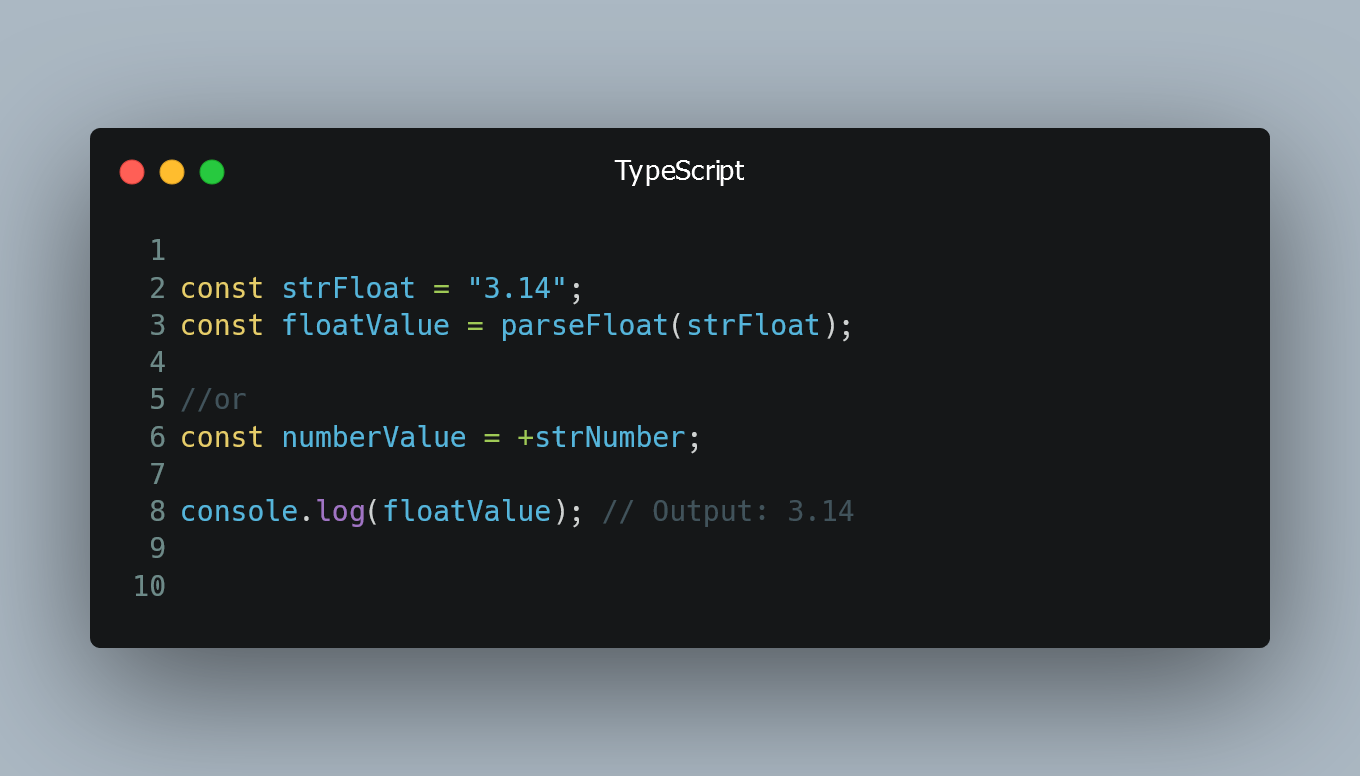
In TypeScript, you may need to convert a string to a number for various reasons, such as performing mathematical operations or working with numeric data.
#1. Using parseInt()
Function
The parseInt()
function is a built-in JavaScript method that converts a string to an integer number.
const strNumber = "42";
const numberValue = parseInt(strNumber);
console.log(numberValue); // Output: 42
In this example, we have a string strNumber
containing the value "42". The parseInt()
function converts the string to an integer, and the variable numberValue
now holds the numeric value 42.
#2. Using parseFloat()
Function
Similarly, if you want to convert a string to a floating-point number, you can use the parseFloat()
function.
const strFloat = "3.14";
const floatValue = parseFloat(strFloat);
console.log(floatValue); // Output: 3.14
In this example, the string strFloat
contains the value "3.14". The parseFloat()
function converts the string to a floating-point number, and the variable floatValue
now holds the numeric value 3.14.
#3. Using Unary Plus Operator
Another simple way to convert a string to a number is by using the unary plus operator (+
).
const strNumber = "42";
const numberValue = +strNumber;
console.log(numberValue); // Output: 42
In this example, the unary plus operator is applied to the string strNumber
, converting it to a numeric value, which is then assigned to the variable numberValue
.
#4. Using Number()
Constructor
The Number()
constructor can also be used to convert a string to a number.
const strNumber = "42";
const numberValue = Number(strNumber);
console.log(numberValue); // Output: 42
In this example, the Number()
constructor converts the string strNumber
to a numeric value, which is stored in the variable numberValue
.
Keep in mind that when converting a string to a number, TypeScript may throw a runtime error if the string cannot be parsed as a valid number. To handle such scenarios, consider using error handling mechanisms like isNaN()
to check if the result is NaN (Not-a-Number).
0 Comment