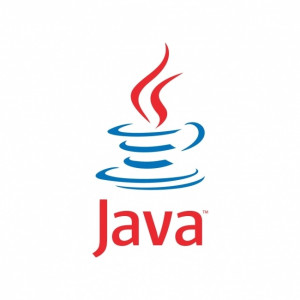
In Java, you may need to convert a String to an int when dealing with user inputs, configuration settings, or any other scenario where numeric values are represented as strings.
Using Integer.parseInt()
The simplest and most common way to convert a String to an int is by using the Integer.parseInt()
method, which parses the string representation of an integer and returns the corresponding int value.
Example:
public class Main {
public static void main(String[] args) {
String strNumber = "42";
int intValue = Integer.parseInt(strNumber);
System.out.println("Converted int value: " + intValue);
}
}
In this example, we use Integer.parseInt()
to convert the String "42" to the int value 42
. The converted int value is then printed.
Handling NumberFormatException
When using Integer.parseInt()
, you must handle the NumberFormatException
that can occur if the input String does not represent a valid integer.
Example:
public class Main {
public static void main(String[] args) {
String strNumber = "invalid";
try {
int intValue = Integer.parseInt(strNumber);
System.out.println("Converted int value: " + intValue);
} catch (NumberFormatException e) {
System.out.println("Invalid input: " + strNumber + " is not a valid integer.");
}
}
}
In this example, we attempt to convert the String "invalid" to an int using Integer.parseInt()
. Since "invalid" is not a valid integer, a NumberFormatException
will be thrown. We handle this exception using a try-catch block to gracefully handle the invalid input.
Using Integer.valueOf()
Another option for converting a String to an int is by using the Integer.valueOf()
method. It works similarly to Integer.parseInt()
but returns an Integer
object instead of a primitive int.
Example:
public class Main {
public static void main(String[] args) {
String strNumber = "123";
Integer integerValue = Integer.valueOf(strNumber);
int intValue = integerValue.intValue();
System.out.println("Converted int value: " + intValue);
}
}
In this example, we use Integer.valueOf()
to convert the String "123" to an Integer
object, which we then convert to the int value 123
using intValue()
.
0 Comment