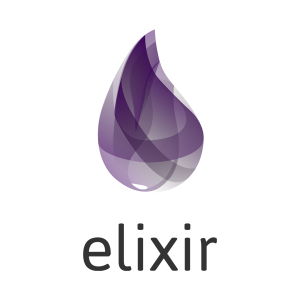
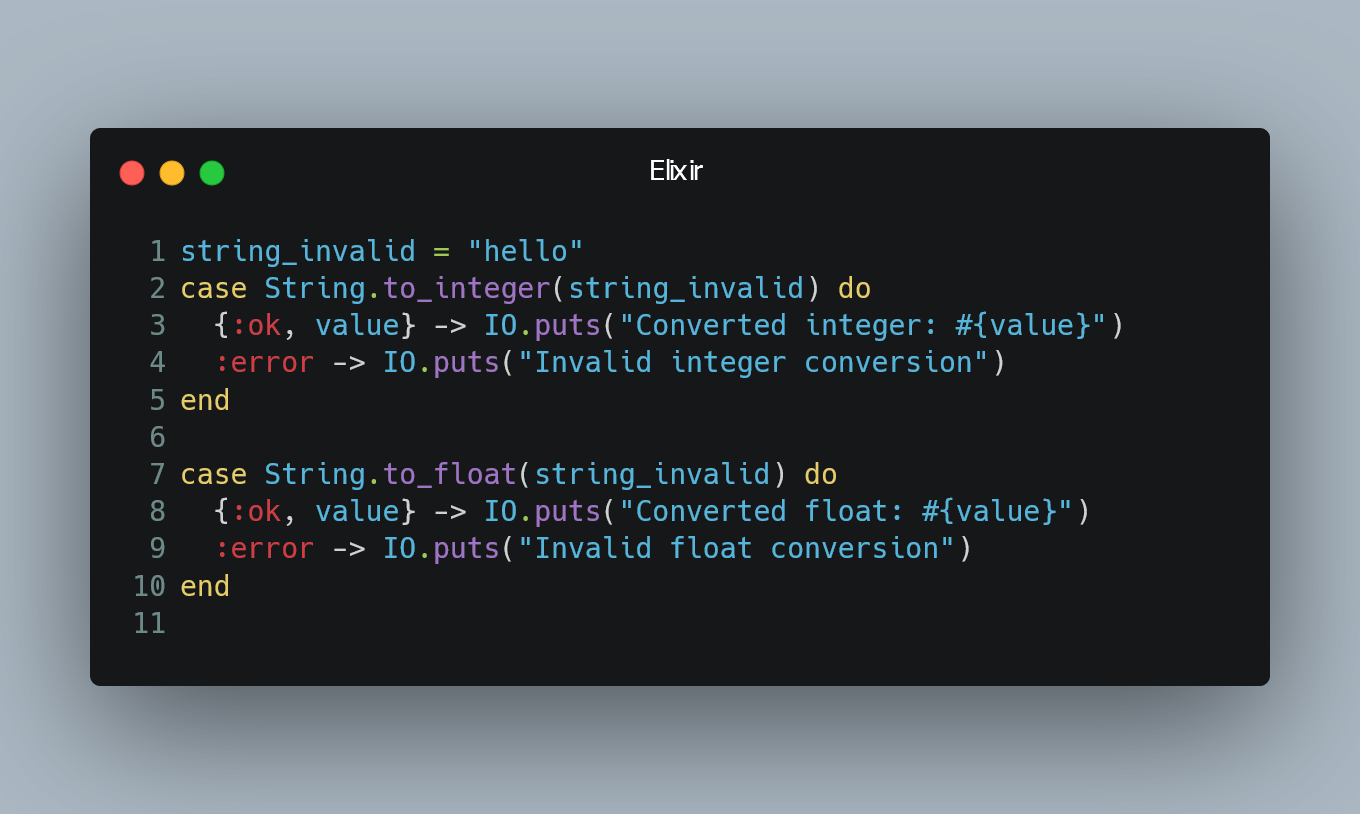
In Elixir, you can convert a string to an integer or a float using built-in functions that handle the conversion process.
Using String.to_integer()
The String.to_integer/1
function is used to convert a string to an integer.
Example:
string_int = "42"
integer_value = String.to_integer(string_int)
IO.puts(integer_value) # Output: 42
In this example, we use String.to_integer/1
to convert the string "42"
to an integer with the value 42
.
Using String.to_float()
The String.to_float/1
function is used to convert a string to a floating-point number (float).
Example:
string_float = "3.14"
float_value = String.to_float(string_float)
IO.puts(float_value) # Output: 3.14
In this example, we use String.to_float/1
to convert the string "3.14"
to a floating-point number with the value 3.14
.
Handling Invalid Conversions
When converting a string to an integer or a float, it's essential to handle cases where the string cannot be converted to a valid number.
Example:
string_invalid = "hello"
case String.to_integer(string_invalid) do
{:ok, value} -> IO.puts("Converted integer: #{value}")
:error -> IO.puts("Invalid integer conversion")
end
case String.to_float(string_invalid) do
{:ok, value} -> IO.puts("Converted float: #{value}")
:error -> IO.puts("Invalid float conversion")
end
In this example, we use a case statement to handle potential invalid conversions. If the conversion is successful, the value is printed; otherwise, an error message is displayed.
0 Comment