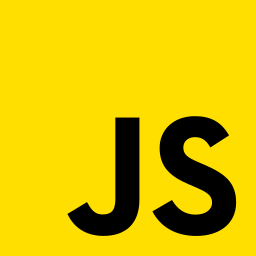
In JavaScript, you can easily check whether a string contains a substring using different methods and techniques.
Using String.includes()
The String.includes()
method is a straightforward way to check if a string contains a specific substring. It returns a boolean value indicating whether the substring is found within the original string.
Example
const sentence = "The quick brown fox jumps over the lazy dog";
const substring = "fox";
console.log(sentence.includes(substring)); // Output: true
Using String.indexOf()
The String.indexOf()
method can also be used to check for the presence of a substring in a string. It returns the index of the first occurrence of the substring or -1 if the substring is not found.
Example
const sentence = "The quick brown fox jumps over the lazy dog";
const substring = "fox";
console.log(sentence.indexOf(substring) !== -1); // Output: true
Using Regular Expressions
Regular expressions offer a more powerful way to check for substrings with more complex patterns.
Example
const sentence = "The quick brown fox jumps over the lazy dog";
const regex = /fox/;
console.log(regex.test(sentence)); // Output: true
Case Insensitive Check
If you need to perform a case-insensitive substring check, you can use either String.toLowerCase()
or the case-insensitive flag (i
) with regular expressions.
Example with String.toLowerCase()
const sentence = "The quick brown fox jumps over the lazy dog";
const substring = "FOX";
console.log(sentence.toLowerCase().includes(substring.toLowerCase())); // Output: true
Example with Regular Expression (case-insensitive flag)
const sentence = "The quick brown fox jumps over the lazy dog";
const regex = /fox/i;
console.log(regex.test(sentence)); // Output: true
0 Comment