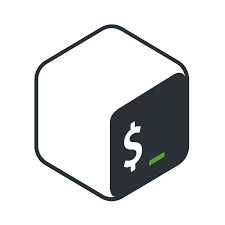
To determine whether a variable is set or not in Bash, you can use various methods and conditional checks.
Using test
command or [ ]
brackets
The test
command, or its equivalent [ ]
brackets, can be used to check if a variable is set.
# Declare the variable
variable="Hello"
# Check if the variable is set
if test -n "$variable"; then
echo "Variable is set."
fi
or
# Declare the variable
variable="Hello"
# Check if the variable is set
if [ -n "$variable" ]; then
echo "Variable is set."
fi
In both cases, the -n
flag checks if the length of the variable is greater than zero, which indicates that the variable is set.
Using [[ ]]
double brackets (preferred method)
The double brackets [[ ]]
provide extended test capabilities and are generally preferred in Bash scripts.
# Declare the variable
variable="Hello"
# Check if the variable is set
if [[ -n "$variable" ]]; then
echo "Variable is set."
fi
The [[ ]]
approach works similarly to the test
command but offers better readability and additional features.
Using isset
function (custom function)
You can create a custom function to check if a variable is set.
# Define the isset function
isset() {
[[ ${!1+x} ]]
}
# Declare the variable
variable="Hello"
# Check if the variable is set
if isset variable; then
echo "Variable is set."
fi
The isset
function uses indirect expansion (${!1+x}
) to check if the variable exists.
Using :-
operator for default value
You can use the :-
operator to assign a default value if the variable is not set.
# Unset variable
# variable=""
# Check if the variable is set, otherwise assign a default value
echo "Value: ${variable:-default_value}"
If the variable
is not set, it will print default_value
; otherwise, it will print the value of the variable
.
Using declare
command
The declare
command can be used to check if a variable is set.
# Declare the variable
variable="Hello"
# Check if the variable is set
if declare -p variable &> /dev/null; then
echo "Variable is set."
fi
The declare -p
command prints the attributes and values of variables. By redirecting the output to /dev/null
, we suppress the output and only use the return status to check if the variable is set.
0 Comment