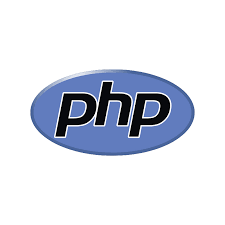
In PHP, you can easily check whether a string contains a specific word using various built-in functions.
Using strpos() Function
What is strpos()?
The strpos()
function is a PHP string function that finds the position of the first occurrence of a substring in a string. It can be used to check if a specific word exists within a string.
Syntax of strpos()
strpos($haystack, $needle);
Here, $haystack
is the original string in which you want to search, and $needle
is the word you want to find.
Example
$text = "The quick brown fox jumps over the lazy dog.";
$word = "fox";
if (strpos($text, $word) !== false) {
echo "The word '$word' is found in the text.";
} else {
echo "The word '$word' is not found in the text.";
}
In this example, the strpos()
function is used to check if the word "fox" exists in the $text
variable. If the word is found, it will print "The word 'fox' is found in the text."; otherwise, it will print "The word 'fox' is not found in the text.".
Using strstr() Function
What is strstr()?
The strstr()
function is another PHP string function that checks if a substring exists in a string. It is similar to strpos()
but returns the part of the haystack string from the first occurrence of the needle to the end.
Syntax of strstr()
strstr($haystack, $needle);
Here, $haystack
is the original string in which you want to search, and $needle
is the word you want to find.
Example
$text = "The quick brown fox jumps over the lazy dog.";
$word = "fox";
if (strstr($text, $word)) {
echo "The word '$word' is found in the text.";
} else {
echo "The word '$word' is not found in the text.";
}
In this example, the strstr()
function is used to check if the word "fox" exists in the $text
variable. If the word is found, it will print "The word 'fox' is found in the text."; otherwise, it will print "The word 'fox' is not found in the text.".
0 Comment