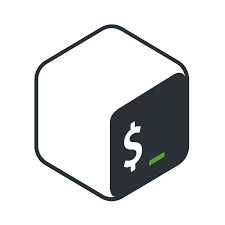
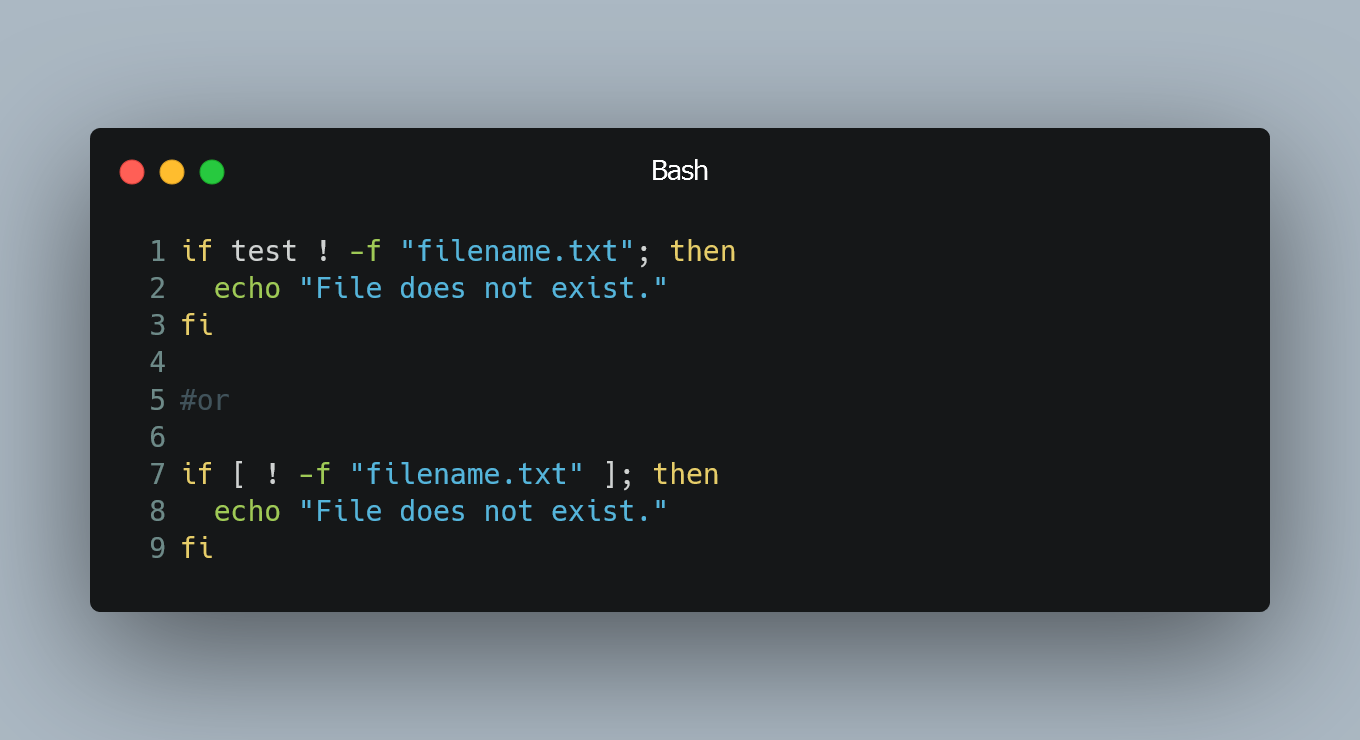
To determine whether a file does not exist in Bash, you can use various commands and operators to perform the check.
Using the test
command or [ ]
brackets
The test
command, or its equivalent [ ]
brackets, allow you to check for file existence.
if test ! -f "filename.txt"; then
echo "File does not exist."
fi
or
if [ ! -f "filename.txt" ]; then
echo "File does not exist."
fi
In both cases, the -f
flag checks whether the given file exists and is a regular file. The !
operator negates the result, so the condition is true when the file does not exist.
Using [[ ]]
double brackets (preferred method)
The double brackets [[ ]]
provide extended test capabilities and are generally preferred in Bash scripts.
if [[ ! -f "filename.txt" ]]; then
echo "File does not exist."
fi
The [[ ]]
approach works similarly to the test
command but offers better readability and additional features.
Using !
with test -e
or [[ -e ]]
The -e
flag checks for the existence of any type of file, not just regular files.
if ! test -e "filename.txt"; then
echo "File does not exist."
fi
or
if ! [[ -e "filename.txt" ]]; then
echo "File does not exist."
fi
The !
operator negates the result, making the condition true if the file does not exist.
Using !
with stat
command
The stat
command allows you to get detailed information about a file, and it can be used to check for file existence.
if ! stat "filename.txt" &> /dev/null; then
echo "File does not exist."
fi
The &> /dev/null
part redirects the output and error messages to /dev/null
, ensuring a silent operation.
Using find
command
You can also utilize the find
command to search for the file and take action accordingly.
if ! find "filename.txt" -type f -print -quit | grep -q .; then
echo "File does not exist."
fi
The -type f
flag ensures that only regular files are considered, and -print -quit
makes the search stop after finding the first match.
0 Comment