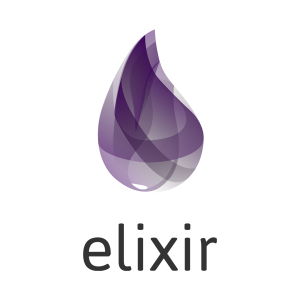
In Elixir, you can determine the type of a variable using various functions and operators. Checking the type of a variable can be useful for conditional branching and ensuring proper handling of data.
Using is_* Functions
Elixir provides a set of is_*
functions that allow you to check if a variable belongs to a specific type. These functions return true
or false
based on the type evaluation.
Example:
value = 42
IO.puts(is_integer(value)) # Output: true
IO.puts(is_float(value)) # Output: false
IO.puts(is_list(value)) # Output: false
In this example, we use is_integer/1
, is_float/1
, and is_list/1
to check if the value
variable is an integer, a float, or a list, respectively.
Using the is_map Function
To check if a variable is a map, you can use the is_map/1
function.
Example:
data = %{"name" => "John", "age" => 30}
IO.puts(is_map(data)) # Output: true
In this example, we use is_map/1
to check if the data
variable is a map.
Using the is_binary Function
To check if a variable is a binary (a sequence of bytes), you can use the is_binary/1
function.
Example:
binary_data = <<1, 2, 3>>
IO.puts(is_binary(binary_data)) # Output: true
In this example, we use is_binary/1
to check if the binary_data
variable is a binary.
Using Pattern Matching
Pattern matching is another powerful way to determine the type of a variable. You can use pattern matching with case statements or function clauses to handle different types of variables separately.
Example:
defmodule TypeChecker do
def check_type(value) when is_integer(value) do
IO.puts("It's an integer.")
end
def check_type(value) when is_list(value) do
IO.puts("It's a list.")
end
def check_type(_) do
IO.puts("Unknown type.")
end
end
TypeChecker.check_type(42) # Output: It's an integer.
TypeChecker.check_type([1,2]) # Output: It's a list.
TypeChecker.check_type(:atom) # Output: Unknown type.
In this example, we define a module TypeChecker
with multiple function clauses that use pattern matching to handle different types of variables.
0 Comment