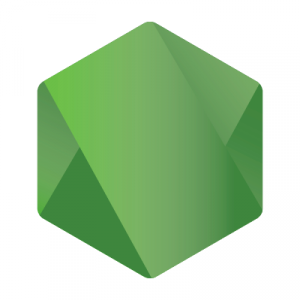
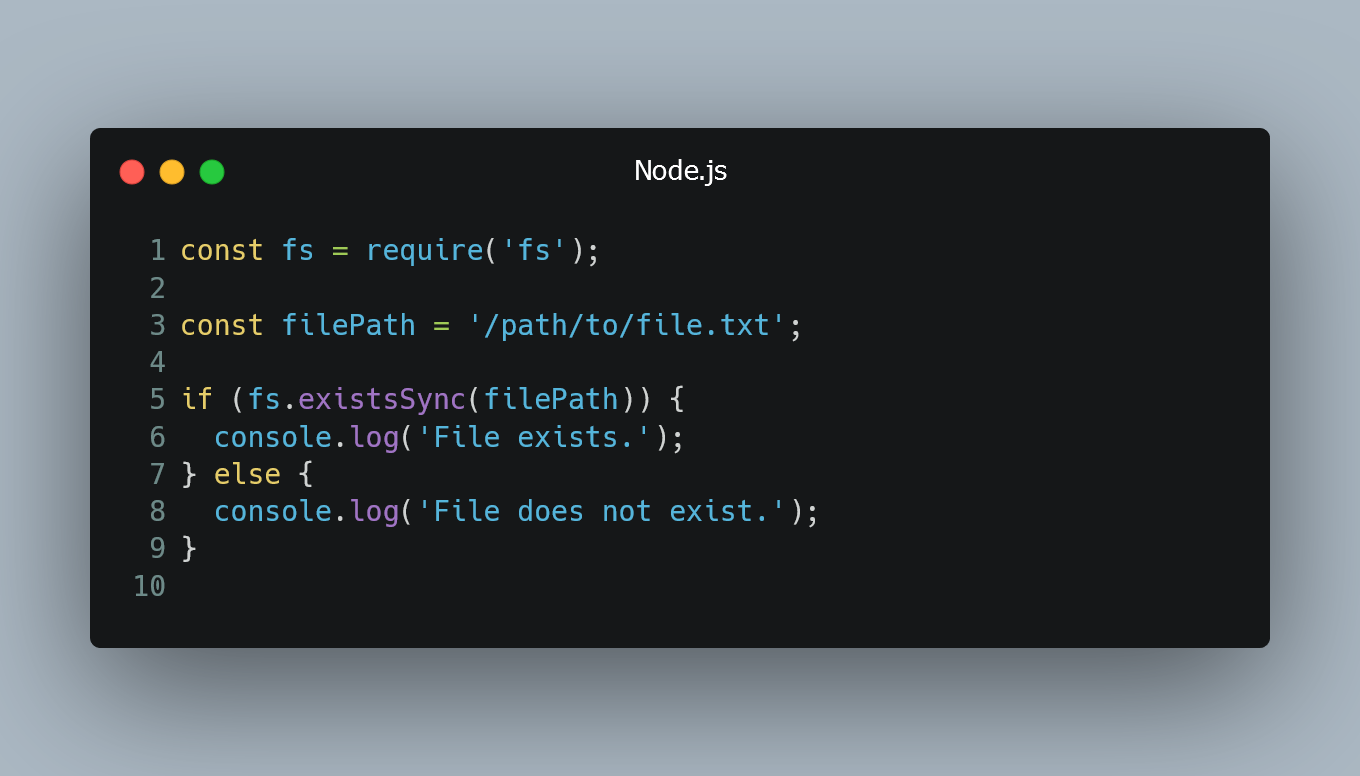
In Node.js, you can check synchronously if a file or directory exists using built-in functions from the fs
module. Synchronous file system operations are straightforward and can be useful in certain scenarios where you want to block the execution until the existence of the file or directory is determined.
Checking if a File Exists Synchronously
To check if a file exists synchronously, you can use the fs.existsSync()
function. It returns a boolean value, true
if the file exists and false
otherwise.
const fs = require('fs');
const filePath = '/path/to/file.txt';
if (fs.existsSync(filePath)) {
console.log('File exists.');
} else {
console.log('File does not exist.');
}
Replace /path/to/file.txt
with the actual file path you want to check. If the file exists, the message "File exists." will be printed; otherwise, "File does not exist." will be printed.
Checking if a Directory Exists Synchronously
To check if a directory exists synchronously, you can use the fs.existsSync()
function with the directory path. It behaves similarly to checking the file existence.
const fs = require('fs');
const directoryPath = '/path/to/directory';
if (fs.existsSync(directoryPath)) {
console.log('Directory exists.');
} else {
console.log('Directory does not exist.');
}
Replace /path/to/directory
with the actual directory path you want to check. If the directory exists, the message "Directory exists." will be printed; otherwise, "Directory does not exist." will be printed.
0 Comment